How to Load an Audio Buffer & Play it using the Audio Tag ?
Last Updated :
21 Mar, 2024
Playing audio files on a web page is something people often need when making websites. One way to do this is by loading the audio into a buffer and playing it using the HTML <audio> tag. This method makes it easy to handle and play audio files right in the web browser.
Approach
- Firstly we select a file input element (fileInput) from the DOM and attach a change event listener to it.
- When a file is selected (change event), we retrieve the selected file from the event object.
- We create a new instance of FileReader (reader) to read the contents of the selected file.
- Upon successful reading (reader.onload), the file contents are available in event.target.result as an ArrayBuffer.
- Finally, we can then decode the ArrayBuffer into an audio buffer for playback or further processing.
Example: Implementation of Loading an Audio buffer and playing it using the audio tag.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Audio Player - Loading Audio Buffer</title>
<style>
body {
margin: auto;
margin-top: 10%;
margin-left: 40vw;
}
h1,
h3 {
text-align: justify;
color: green;
margin: 20px;
}
.audioele {
margin-left: 10vw;
}
</style>
</head>
<body>
<h3>Load an Audio Buffer and
Play it using the Audio Tag?
</h3>
<div class="audioele">
<input type="file" id="fileInput"
accept="audio/*">
</div>
<script>
let fileInput = document.getElementById('fileInput');
fileInput.addEventListener('change', function (event) {
let file = event.target.files[0];
let reader = new FileReader();
reader.onload = function (event) {
let audioContext = new (window.AudioContext ||
window.webkitAudioContext)();
audioContext.decodeAudioData(event
.target.result, function (buffer) {
let audioPlayer = document.createElement('audio');
audioPlayer.controls = true;
audioPlayer.src = URL.createObjectURL(file);
document.body.appendChild(audioPlayer);
});
};
reader.readAsArrayBuffer(file);
});
</script>
</body>
</html>
Output:
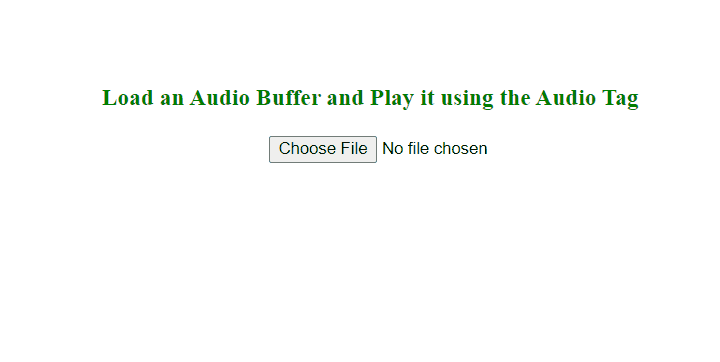
Output
Share your thoughts in the comments
Please Login to comment...