How to Fix React useEffect running twice in React 18 ?
Last Updated :
07 May, 2024
In React applications, the useEffect hook is commonly used for handling side effects, such as data fetching, subscriptions, or manually changing the DOM. However, encountering the issue of useEffect running twice can lead to unexpected behavior and impact the performance of your application. In this article, we’ll explore various approaches to resolve this error and provide examples for each approach.
Steps to Create React Application
Step 1: Create a React application using the following command:
npm create vite@latest
Step 2: Provide the Project name and move to the root directory using the below command.
cd <foldername>
Step 3: In the directory of the project write a command in the terminal to install the dependency.
npm install
Why useEffect is running twice?
The useEffect hook in React is designed to execute side effects in function components. However, if not used correctly, it might run multiple times, leading to unexpected behavior in your application.
JavaScript
import { useEffect } from "react"
function App() {
useEffect(() => {
console.log("Calling GFG by use effect")
}, [])
return (
<>
<h1 className="gfg">Geeks for Geeks </h1>
</>
)
}
export default App
Output:
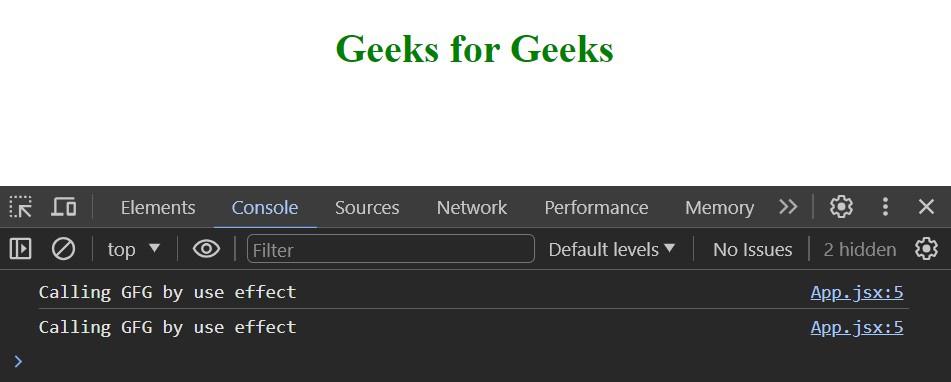
Approaches for Resolving the Error
- Ensure correct dependency array: If you’re using React hooks like
useState
or useEffect
, ensure that the dependency array is correct. Missing dependencies can lead to stale data or infinite loops. - Check component rendering logic: Review your component’s rendering logic to ensure it’s correct. Check conditional rendering, mapping of arrays, and logic inside JSX elements.
- Use conditional execution: Implement conditional execution where necessary to handle edge cases or avoid errors. This could involve using
if
statements or ternary operators to conditionally render components or execute code. - Optimize event handlers: If you’re experiencing performance issues related to event handlers, consider optimizing them. This could involve debouncing or throttling functions, reducing unnecessary re-renders, or optimizing event listener attachment/removal.
- Utilize debugging tools: Take advantage of debugging tools provided by NextJS, React, and your browser’s developer tools. Use console logging, breakpoints, and error boundaries to identify and debug issues in your application.
Steps to Solve useEffect Running Twice
- Understand the Error: Reacts useEffect hook is designed to handle side effects in function components. When useEffect runs twice, it can lead to unexpected behavior and performance issues in your application.
- Using React Strict Mode: Enable React Strict Mode in your application to detect and highlight potential issues during development. React Strict Mode helps identify common mistakes and encourages best practices for React development.
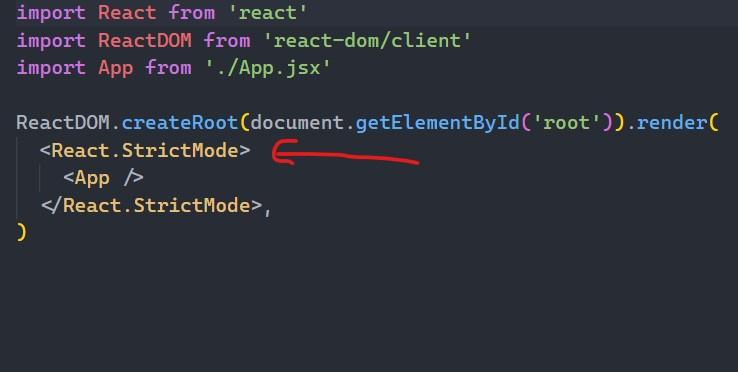
Fig :- Restrict mode in react
- Remove Strict Mode : While React Strict Mode performs additional checks and warnings to help you identify and fix potential issues in your application, it may also inadvertently trigger re-renders and cause the useEffect hook to run multiple times. Removing Strict Mode can sometimes resolve the issue of useEffect running twice by eliminating these additional checks and allowing the component to render only once.
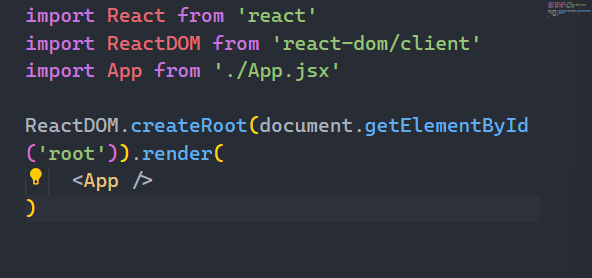
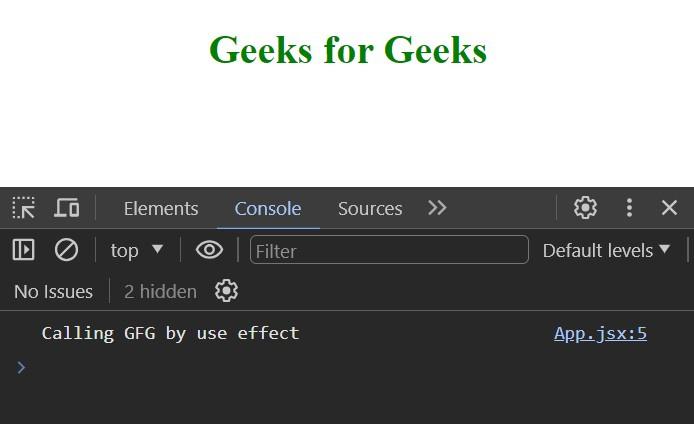
Fig :- Console after removing of Strict mode
Some other reasons are as follows
- Parent Component Re-renders: If the parent component of App re-renders, it will cause App to re-render as well, triggering the useEffect hook again.
- Global State Changes: If the useEffect hook depends on global state managed by a state management library like Redux or Context API, changes to that global state may trigger re-renders and consequently re-run the useEffect hook.
- Changes to Context Values: If App consumes context values provided by a Context Provider, changes to those context values may cause App to re-render and the useEffect hook to be called again.
More possible approached to prevent useEffect running twice
- Review the dependency array of the useEffect hook and ensure that it includes only the necessary dependencies. If the useEffect hook relies on specific state or props, include those dependencies in the dependency array to control when the effect should run.
- If the useEffect hook should only run once when the component mounts, ensure that the dependency array is empty ([]). This tells React to run the effect only once when the component mounts and not re-run it on subsequent re-renders.
- If the useEffect hook depends on global state or context values, consider optimizing the component rendering to minimize unnecessary re-renders. You can use React.memo for functional components or shouldComponentUpdate for class components to prevent re-renders when the component’s props or state haven’t changed.
Share your thoughts in the comments
Please Login to comment...