The key feature of React is its useEffect hook, which allows developers to manage side effects in the React components. However, if not used correctly, the useEffect hook can lead to infinite loops, causing performance issues and crashing the application. In this article, we will explore the common causes of infinite loops and how to avoid them.
Avoid infinite loops in useEffect() by providing appropriate dependencies in the dependency array. Use an empty array for one-time execution, a specific state value for triggering effect, or multiple state values with conditions for selective execution.
Prerequisites:
To Avoid Infinite Loops When using the useEffect() in ReactJS we have these approaches:
useEffect() Hook
The useEffect() hook in ReactJS is used to perform side effects in functional components. It runs a function after every render, allowing for actions like data fetching, subscriptions, or modifying the DOM.
The problem addressed here is the possibility of encountering infinite loops when using the useEffect hook in React. Some of the common causes are discussed below:
Common Problems while using useEffect Hook:
Not specifying dependencies:
The most common cause of infinite loops is not specifying dependencies correctly. If you do not pass any dependencies, the hook will be called after every render, leading to an infinite loop. To avoid this, you should always specify the dependencies that the hook depends on.
For example, if you are fetching data from an API, you should pass the API endpoint as a dependency. This ensures that the hook is only called when the endpoint changes.
useEffect(() => {
fetchData(endpoint);
}, [endpoint]);
Modifying the state inside the hook:
Another common mistake is modifying the state inside the useEffect hook. When you modify the state inside the hook, it triggers a re-render, which causes the hook to be called again. This creates an infinite loop.
To avoid this, you should only modify the state inside event handlers or other state update functions, such as useState or useReducer.
Incorrect use of conditional statements:
Conditional statements can also cause infinite loops if not used correctly. If a conditional statement depends on state that is modified inside the hook, it can cause the hook to be called repeatedly.
To avoid this, you should move the conditional statement outside the hook and use a separate state variable to track the condition.
const [modal, setModal] = useState(false);
useEffect(() => {
if (modal) {
// Do something
}
}, [modal]);
function handleClick() {
setModal(true);
}
The solution presented in the article focuses on avoiding infinite loops by correctly specifying dependencies and managing state updates outside the useEffect hook. Some of them are:
Specify dependencies correctly:
To avoid infinite loops, you should always specify the dependencies that the hook depends on. This ensures that the hook is only called when the dependencies change.
useEffect(() => {
// Effect code
}, [dependency1, dependency2, ...]);
Move state updates outside the hook:
To avoid infinite loops caused by modifying the state inside the hook, you should move state updates outside the hook and use event handlers or other state update functions.
const handleClick = () => {
setCount(count + 1);
};
useEffect(() => {
// Effect code
}, [dependency]);
Use memoization:
Memoization is a technique that is used in optimizing the performance of functions by caching the results done by function calls. We can also use memoization to optimize the performance of your useEffect hook by caching the results of expensive operations.
const memoizedCallback = useCallback(
() => {
// do something
},
[dependency],
);
useEffect(() => {
memoizedCallback();
}, [memoizedCallback]);
Steps to create React Application:
Step 1: Create a React project:
npx create-react-app appname
Step 2: After creating your project folder i.e. appname, move to it using the following command:
cd appname
Project Structure:
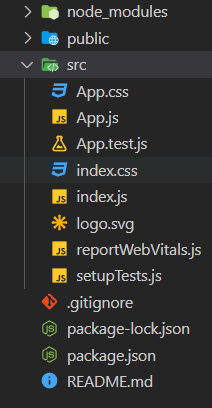
Now, we will see some examples to avoid or prevent the infinite loops when using the useEffect() hook with a different approach.
Approach 1: Using Empty Dependency Array
In this example, we are fetching data from an API using the useEffect hook. We pass an empty array as the dependency array, which means that the hook will only be called once after the component mounts. If we didn’t specify any dependencies, the hook would be called after every render, causing an infinite loop. By specifying the empty array as the dependency, we ensure that the hook is only called once, and we avoid any performance issues or crashes.
Step to Install axios module:
npm i axios
The updated dependencies in package.json file.
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"axios": "^1.6.2",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
},
Example: Below example demonstrates how to avoid infinite loops when using the useEffect hook in React.
Javascript
import React, {
useState,
useEffect
} from 'react' ;
import axios from 'axios' ;
function App() {
const [data, setData] = useState([]);
const [loading, setLoading] = useState( false );
useEffect(() => {
setLoading( true );
axios.get(
.then((response) => {
setData(response.data);
setLoading( false );
})
. catch ((error) => {
console.log(error);
setLoading( false );
});
}, []);
if (loading) {
return <p>Loading data...</p>;
}
return (
<div>
<center>
<h1 style={{ color: 'green' }}>
GeeksforGeeks
</h1>
<h3>
How to avoid infinite loop when
using useEffect hook in React
</h3>
</center>
<h3>Posts</h3>
<ul>
{data.map((post) => (
<li key={post.id}>
<h2>{post.title}</h2>
<p>{post.body}</p>
</li>
))}
</ul>
</div>
);
}
export default App;
|
Steps to Run the Application: Use this command in the terminal inside the project directory.
npm start
Output: This output will be visible on the http://localhost:3000 on the browser window.
.gif)
Approach 2: Using Memoization
In this example, we are using the useCallback hook to memoize the handleClick function. This function is passed down to the ChildComponent as a prop, so we want to ensure that it is only re-created when its dependencies change. We pass an empty array as the dependency array, which means that the function is only created once.
Example: Below example demonstrates how to avoid infinite loops using memoization in the useEffect hook in React.
Javascript
import React, {
useState,
useEffect,
useCallback
} from 'react' ;
function ChildComponent({ onClick }) {
return <button onClick={onClick}>Click me</button>;
}
function App() {
const [count, setCount] = useState(0);
const handleClick = useCallback(() => {
console.log( 'Clicked!' );
}, []);
useEffect(() => {
console.log( 'Count changed!' );
}, [count]);
return (
<div>
<center>
<h1 style={{ color: 'green' }}>
GeeksforGeeks
</h1>
<h3>
How to avoid infinite loop when
using useEffect hook in React
</h3>
</center>
<h1>
Count: {count}
</h1>
<ChildComponent onClick={handleClick} />
<button onClick={() => setCount(count + 1)}>
Increment count
</button>
</div>
);
}
export default App;
|
Steps to Run the Application: Use this command in the terminal inside the project directory.
npm start
Output: This output will be visible on the http://localhost:3000 on the browser window.
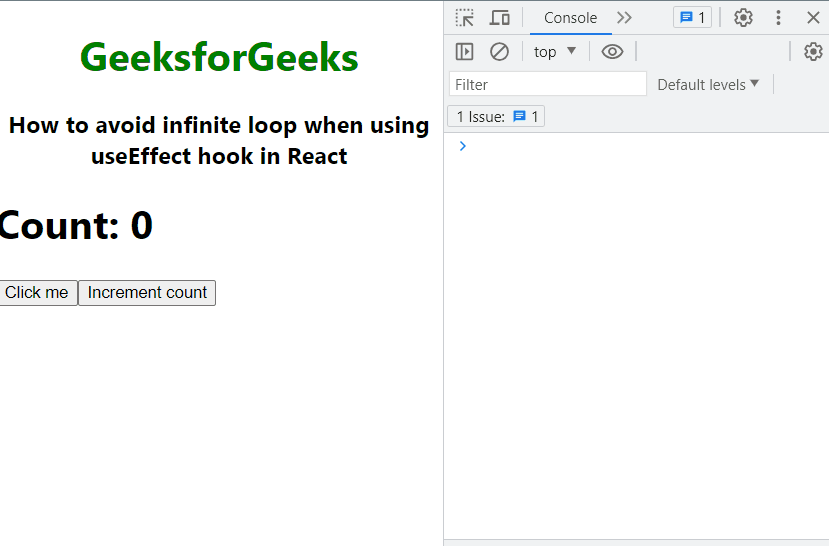
Share your thoughts in the comments
Please Login to comment...