Effect Management with useEffect Hook in React
Last Updated :
26 Feb, 2024
useEffect serves as a foundational tool in React development, enabling developers to orchestrate side effects within functional components systematically. It facilitates the management of asynchronous tasks, such as data fetching and DOM manipulation, enhancing code organization and maintainability. By defining when these side effects should occur, useEffect promotes a structured approach to development, leading to more robust and scalable applications.
Approach to manage Effects with useEffect Hook:
- Before useEffect, managing side effects in functional components was cumbersome, and often required class components or external libraries. The useEffect offers a concise and integrated solution:
- Declarative Nature: You describe the desired side effect, and React takes care of the when and how, simplifying your code.
- Lifecycle Integration: Execute effects at specific stages of a component’s lifecycle, like componentDidMount or componentWillUnmount, for granular control.
- Dependency Array: Fine-tune when the effect re-runs by specifying the values it relies on. This optimizes performance by preventing unnecessary re-renders.
- Cleanup Function: Like a responsible citizen, clean up after yourself! This optional function allows you to release resources acquired in the effect, preventing memory leaks or other issues.
Importing useEffect Hook in React:
To utilize useEffect , import it from the react library at the top of your component file.
import React, { useEffect } from 'react';
Syntax of useEffect Hook:
Within your functional component, call useEffect with the effect function and an array of dependencies as arguments.
useEffect(() => {
// Your effect function here
}, [dependencies]);
- Effect function: This function contains the side effect logic (e.g., fetching data, setting up subscriptions).
- Dependencies (optional): An array of values that control when the effect runs. If no dependencies are provided, the effect run every render. It determining when the effect re-runs. An empty array [ ] means the effect runs only once on mount.
- The useEffect runs after the component renders, so don’t directly modify the DOM inside it. Use state updates instead.
- Always clean up resources in the cleanup function (return function) to avoid memory leaks and unexpected behavior.
Steps to Create React Application:
Step 1: Create a react application using the following command.
npx create-react-application my-react-app
Step 2: Naviate to the root directory of your application using the following command.
cd my-react-app
Example of useEffect Hook for Effect Management:
Example 1: Fetching Data: This React component fetches data from an API when it’s first shown on the screen. It then displays the fetched data or a loading message while waiting.
Javascript
import React,
{
useState,
useEffect
} from 'react' ;
function MyComponent() {
const [data, setData] = useState( null );
useEffect(
() => {
.then(response => response.json())
.then(data => setData(data));
}, []
);
return (
<div>
{
data ?
<p>Data: {JSON.stringify(data)}</p> :
<p>Loading...</p>
}
</div>
);
}
export default MyComponent;
|
Step to start the Application:
npm start
Output:
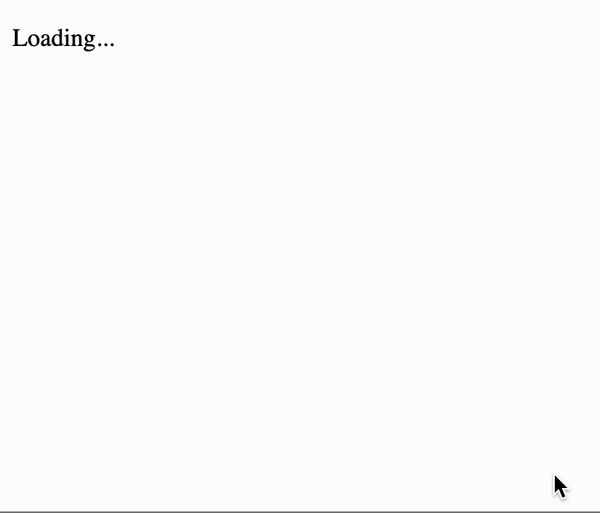
output 1
Example 2: Setting Up and Cleaning Up Timers:
- Adapt the code to your specific use cases.
- Be mindful of dependency array optimization to avoid unnecessary re-renders.
- Use the cleanup function responsibly to prevent memory leaks or other issues.
By effectively mastering useEffect, you can create well-structured, maintainable, and performant React applications that manage side effects gracefully.
Javascript
import React,
{
useState,
useEffect
} from 'react' ;
function MyComponent() {
const [count, setCount] = useState(0);
useEffect(() => {
const intervalId =
setInterval(
() => setCount(count + 1), 1000);
return () => clearInterval(intervalId);
}, []);
return (
<div>
<p>
Count: {count}
</p>
</div>
);
}
export default MyComponent;
|
Step to start the Application:
npm start
Output:
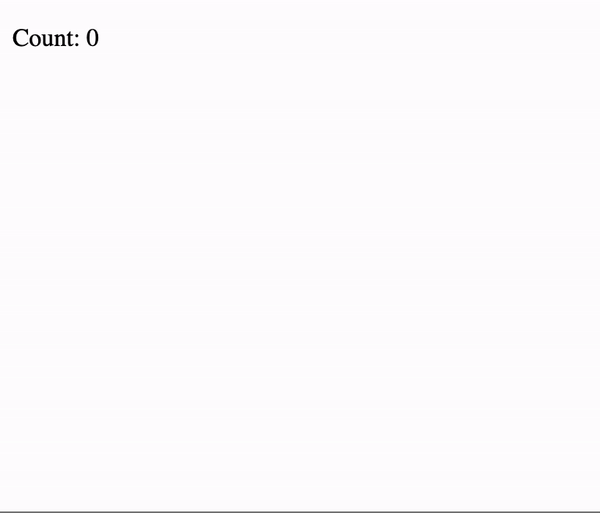
output
Share your thoughts in the comments
Please Login to comment...