How to Create Instagram Like Button in jQuery ?
Last Updated :
24 Oct, 2023
In this article, we will learn how to create an Instagram-like button using jQuery. An Instagram Like button is a user interface element that, when clicked, signifies appreciation or approval for a post. It often features a heart icon and toggles between filled (liked) and outlined (unliked) states.
There are several methods that can be used to create an Instagram Like button in jQuery, which are listed below:
We will explore all the above methods along with their basic implementation with the help of examples.
Approach 1: Using toggleClass() Method
This approach creates a like button using HTML and jQuery. When clicked, it toggles a “liked” class, changing the text and color to represent the like/unlike state.
Syntax
$(selector).toggleClass(classname)
Example: In this example, we are using a Font Awesome heart icon inside a button element. jQuery’s toggleClass method switches between classes, changing the heart’s color from black to red and style from regular to solid when the button is clicked, simulating Instagram’s like button.
HTML
<!DOCTYPE html>
< html >
< head >
< link rel = "stylesheet"
href =
< script src =
</ script >
< style >
button {
background-color: transparent;
border: none;
}
.fa-heart {
font-size: 25px;
}
.blank {
color: #142034;
}
.red {
color: red;
}
</ style >
</ head >
< body >
< h2 style = "color: green;" >
Welcome To GFG
</ h2 >
< p >
Instagram Like Button using toggleClass
</ p >
< button id = "like" >
< i id = "icon"
class = "fa-regular fa-heart blank" >
</ i >
</ button >
< script >
$(document).ready(function () {
$("#like").on("click", function () {
$("#icon").toggleClass("red");
$("#icon").toggleClass("fa-solid");
});
});
</ script >
</ body >
</ html >
|
Output:
Approach 2: Using if-else Conditions
In this approach, we are making an Instagram-like button with Font Awesome heart icons. Upon clicking, it alternates between regular and solid heart styles, with color changes. The behavior is managed using jQuery.
Syntax
if (condition1) {
} else if (condition2) {
} else {
}
Example: In this example we are using above-explained approach.
HTML
<!DOCTYPE html>
< html >
< head >
< link rel = "stylesheet"
href =
integrity =
"sha512-z3gLpd7yknf1YoNbCzqRKc4qyor8gaKU1qmn+CShxbuBusANI9QpRohGBreCFkKxLhei6S9CQXFEbbKuqLg0DA=="
crossorigin = "anonymous"
referrerpolicy = "no-referrer" />
< script src =
integrity =
"sha256-eKhayi8LEQwp4NKxN+CfCh+3qOVUtJn3QNZ0TciWLP4="
crossorigin = "anonymous" >
</ script >
< title >Welcome To GFG</ title >
< Style >
h2 {
color: green;
}
button {
background-color: transparent;
border: none;
}
.fa-heart {
font-size: 40px;
}
</ Style >
</ head >
< body >
< h2 >Welcome To GFG</ h2 >
< p >
Instagram Like Button
</ p >
< div class = "hello" >
< button id = "like" >
< i class = "fa-regular fa-heart" ></ i >
</ button >
</ div >
< script >
$(document).ready(function () {
let lv = 0;
$("#like").on("click", function () {
if (lv == 0) {
$('#like').html(
'< i class = "fa-regular fa-heart" ></ i >');
lv = 1;
} else {
$('#like').html(
'< i class = "fa-solid fa-heart" style = "color: #f52e4b;" ></ i >');
lv = 0
}
});
});
</ script >
</ body >
</ html >
|
Output:
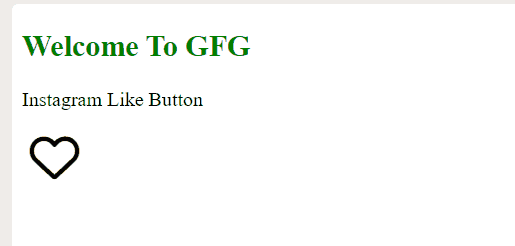
Share your thoughts in the comments
Please Login to comment...