How to Dynamically Add/Remove Table Rows using jQuery ?
Last Updated :
14 Dec, 2023
In this article, we will learn how to dynamically add/remove rows from an HTML table using jQuery. Before reading this article, make sure you have some basic idea about jQuery, its in-built methods, and element selection.
jQuery provides us with a lot of methods to perform various tasks. To dynamically add and remove the rows from an HTML table, we are also going to use some of these jQuery methods like append(), remove(), etc.
Adding a row
To add a row, we will use the append() method. The append() method takes the HTML as a parameter in the form of a string and appends it to the selected element as its child. We will attach a click event to the button and use the append method to append the HTML and create a new row once the user clicks the button.
Removing a row
The remove() method of jQuery will be used to remove the selected element. You just need to select the element to be removed using the jQuery selector’s syntax and use the remove method on the element that you want to remove. Here again, a remove button will be generated dynamically with the addition of the new row which removes the corresponding row once user clicks the button.
Example: The below example will show the practical implementation of Adding and Deleting rows dynamically from an HTML table using jQuery.
HTML
<!DOCTYPE html>
< html >
< head >
< link href =
rel = "stylesheet"
integrity =
"sha384-T3c6CoIi6uLrA9TneNEoa7RxnatzjcDSCmG1MXxSR1GAsXEV/Dwwykc2MPK8M2HN"
crossorigin = "anonymous" >
</ head >
< body >
< div class = "container pt-4" >
< div class = "table-responsive" >
< table class = "table table-bordered" >
< thead >
< tr >
< th class = "text-center" >
Row Number
</ th >
< th class = "text-center" >
Remove Row
</ th >
</ tr >
</ thead >
< tbody id = "tbody" >
</ tbody >
</ table >
</ div >
< button class = "btn btn-md btn-primary"
id = "addBtn" type = "button" >
Add New Row
</ button >
</ div >
< script src =
integrity =
"sha384-C6RzsynM9kWDrMNeT87bh95OGNyZPhcTNXj1NW7RuBCsyN/o0jlpcV8Qyq46cDfL"
crossorigin = "anonymous" >
</ script >
< script src =
integrity =
"sha512-v2CJ7UaYy4JwqLDIrZUI/4hqeoQieOmAZNXBeQyjo21dadnwR+8ZaIJVT8EE2iyI61OV8e6M8PP2/4hpQINQ/g=="
crossorigin = "anonymous" referrerpolicy = "no-referrer" >
</ script >
< script >
$(document).ready(() => {
let count=1;
// Adding row on click to Add New Row button
$('#addBtn').click(function () {
let dynamicRowHTML = `
< tr class = "rowClass" ">
< td class = "row-index text-center" >
Dynamically added Row ${count}
</ td >
< td class = "text-center" >
< button class = "btn btn-danger remove"
type = "button" >Remove
</ button >
</ td >
</ tr >`;
$('#tbody').append(dynamicRowHTML);
count++;
});
// Removing Row on click to Remove button
$('#tbody').on('click', '.remove', function () {
$(this).parent('td.text-center').parent('tr.rowClass').remove();
});
})
</ script >
</ body >
</ html >
|
Output:
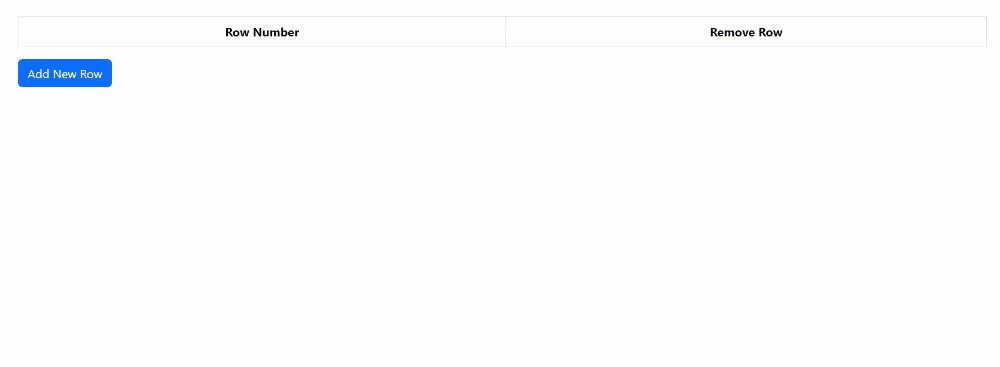
HTML is the foundation of webpages, is used for webpage development by structuring websites and web apps.You can learn HTML from the ground up by following this HTML Tutorial and HTML Examples.
CSS is the foundation of webpages, is used for webpage development by styling websites and web apps.You can learn CSS from the ground up by following this CSS Tutorial and CSS Examples.
jQuery is an open source JavaScript library that simplifies the interactions between an HTML/CSS document, It is widely famous with it’s philosophy of “Write less, do more”.
You can learn jQuery from the ground up by following this jQuery Tutorial and jQuery Examples.
Share your thoughts in the comments
Please Login to comment...