JQuery Set the value of an input text field
Last Updated :
14 Dec, 2023
In this article, we will learn about the different methods of setting the value of the input field on any web page by clicking on a button using jQuery. There are some in-built methods available in jQuery that we can use for this purpose as listed below:
Let us now discuss all of these methods one by one in detail with the help of code examples.
jQuery val() method
This method can be used to return as well as to set the value attribute of selected elements. Here, we are going to use this method to set the value of an input field on the web page. To set the value, you need to pass the value inside the round brackets of the method in the form of a string.
Syntax:
$(selector).val("value")
Example: In this example the value of the input element is set by val() method by selecting the input element using its ID.
html
<!DOCTYPE HTML>
< html >
< head >
< title >
JQuery | Set value of input text.
</ title >
< script src =
</ script >
</ head >
< body style = "text-align: center;" >
< h1 style = "color: green;" >
GeeksForGeeks
</ h1 > Input Box:
< input id = "input"
type = "text"
class = "Disable"
value = "" />
< br >
< br >
< button id = "setText" >
setText
</ button >
< script >
$("#setText").click(function(event) {
$('#input').val("GeeksForGeeks");
});
</ script >
</ body >
</ html >
|
Output:
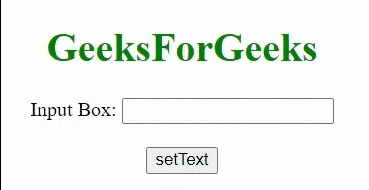
jQuery prop() method
The jQuery prop() method can be used to set the value of a input field by passing the value property and the value to be set as parameters to the prop() method in the form of strings separated by comma.
Syntax:
$('selected_element').prop("value", "value_to_be_set");
Example: The below example will explain the use of the prop() method to set the value of an input text field.
html
<!DOCTYPE HTML>
< html >
< head >
< title >
JQuery | Set value of input text.
</ title >
< script src =
</ script >
</ head >
< body style = "text-align: center;" >
< h1 style = "color: green;" >
GeeksForGeeks
</ h1 > Input Box:
< input id = "input"
type = "text"
class = "Disable"
value = "" />
< br >
< br >
< button id = "setText" >
setText
</ button >
< script >
$("#setText").click(function(event) {
$('#input').prop("value", "GeeksForGeeks");
});
</ script >
</ body >
</ html >
|
Output:
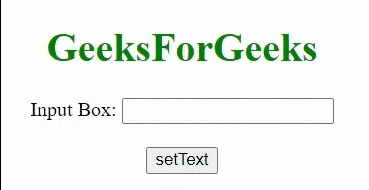
jQuery attr() method
The attr() method can also be used to set the value of an input text field in the sam way as the prop() method was used. You need to pass the value attribute and the value to be set as parameters to this method.
Syntax:
$('selected_element').attr("value", "value_to_be_set");
Example: Below example implements the attr() method to set the value of an input text field.
HTML
<!DOCTYPE HTML>
< html >
< head >
< title >
JQuery | Set value of input text.
</ title >
< script src =
</ script >
</ head >
< body style = "text-align: center;" >
< h1 style = "color: green;" >
GeeksForGeeks
</ h1 > Input Box:
< input id = "input"
type = "text"
class = "Disable"
value = "" />
< br >
< br >
< button id = "setText" >
setText
</ button >
< script >
$("#setText").click(function(event) {
$('#input').attr("value", "GeeksForGeeks");
});
</ script >
</ body >
</ html >
|
Output:
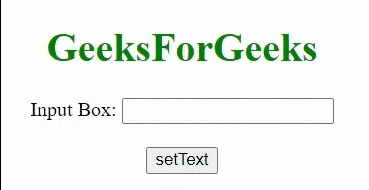
jQuery is an open source JavaScript library that simplifies the interactions between an HTML/CSS document, It is widely famous with it’s philosophy of “Write less, do more”. You can learn jQuery from the ground up by following this jQuery Tutorial and jQuery Examples.
Share your thoughts in the comments
Please Login to comment...