How to create Like Button Using React JS ?
Last Updated :
15 Feb, 2024
This is a social media world and the like button is one of the main components of it. From social media accounts to shopping platforms like buttons are everywhere.
In this tutorial, we’ll explore how to create a dynamic button in a React application. The button will change its appearance based on different states, including default, hover, and liked. Additionally, we’ll incorporate asynchronous handling for API calls when the button is clicked.
Preview of final output: Let us have a look at how the final output will look like.
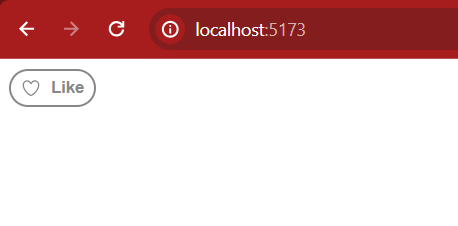
Prerequisites
Approach to create Like button:
The task is to design a like button with the following states:
- Default State: The button appears in a default state.
- Hover State: When hovered, the button turns red.
- Liked State: After clicking, the button transitions to a loading state, indicating that an API call is in progress. The API can either succeed or fail, affecting the final state of the button.
Steps to Create the Project
Step 1: Set Up Your React App with Vite
npm create vite@latest
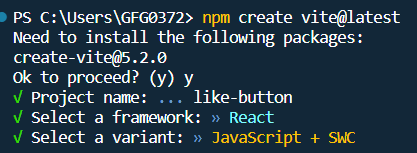
Step 2: Navigate to the Project Directory
cd like-button
Step 3: Install the project dependencies using:
npm install
Project Structure:
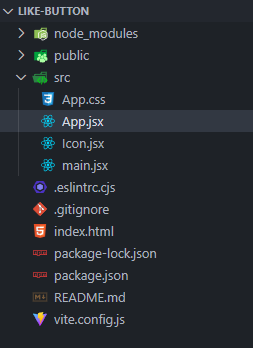
project structure
The updated dependencies in package.json will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0"
},
"devDependencies": {
"@types/react": "^18.2.55",
"@types/react-dom": "^18.2.19",
"@vitejs/plugin-react-swc": "^3.5.0",
"eslint": "^8.56.0",
"eslint-plugin-react": "^7.33.2",
"eslint-plugin-react-hooks": "^4.6.0",
"eslint-plugin-react-refresh": "^0.4.5",
"vite": "^5.1.0"
}
Example: Create the required files and add the following code.
Javascript
import { HeartIcon, SpinnerIcon } from "./Icon" ;
import { useState } from "react" ;
import "./App.css" ;
export default function App() {
const [liked, setLiked] = useState( false );
const [isFetching, setIsFetching] = useState( false );
const [error, setError] = useState( null );
const handleLikeUnlike = async () => {
setError( null );
setIsFetching( true );
try {
const response = await fetch(
{
method: "POST" ,
headers: { "Content-Type" : "application/json" },
body: JSON.stringify({
action: liked ? "unlike" : "like" ,
}),
}
);
if (response.status >= 200 && response.status < 300) {
setLiked(!liked);
} else {
const res = await response.json();
setError(res.message);
return ;
}
} finally {
setIsFetching( false );
}
};
return (
<div>
<button
onClick={handleLikeUnlike}
className={`likeBtn ${liked ? "liked" : "" }`}
>
{isFetching ? <SpinnerIcon /> : <HeartIcon />}
{liked ? "Liked" : "Like" }
</button>
{error && <div className= "error" >{error}</div>}
</div>
);
}
|
Javascript
export function SpinnerIcon({ className }) {
return (
<svg
className={className}
width={16}
height={16}
viewBox= "0 0 38 38"
stroke= "currentColor"
>
<g fill= "none" fillRule= "evenodd" >
<g transform= "translate(1 1)" strokeWidth= "2" >
<circle strokeOpacity= ".5" cx= "18" cy= "18" r= "18" />
<path d= "M36 18c0-9.94-8.06-18-18-18" >
<animateTransform
attributeName= "transform"
type= "rotate"
from= "0 18 18"
to= "360 18 18"
dur= "1s"
repeatCount= "indefinite"
/>
</path>
</g>
</g>
</svg>
);
}
export function HeartIcon({ className }) {
return (
<svg
className={className}
fill= "currentColor"
viewBox= "0 0 24 24"
width= "16"
height= "16"
>
<g>
<path d= "M12 21.638h-.014C9.403 21.59 1.95 14.856 1.95 8.478c0-3.064
2.525-5.754 5.403-5.754 2.29 0 3.83 1.58 4.646 2.73.814-1.148 2.354-2.73
4.645-2.73 2.88 0 5.404 2.69 5.404 5.755 0 6.376-7.454 13.11-10.037
13.157H12zM7.354 4.225c-2.08 0-3.903 1.988-3.903 4.255 0 5.74 7.034 11.596
8.55 11.658 1.518-.062 8.55-5.917 8.55-11.658 0-2.267-1.823-4.255-3.903-4.255-2.528
0-3.94 2.936-3.952 2.965-.23.562-1.156.562-1.387 0-.014-.03-1.425-2.965-3.954-2.965z" >
</path>
</g>
</svg>
);
}
|
Javascript
import React from "react" ;
import ReactDOM from "react-dom/client" ;
import App from "./App.jsx" ;
ReactDOM.createRoot(document.getElementById( "root" )).render(
<React.StrictMode>
<App />
</React.StrictMode>
);
|
CSS
:root {
--default- color : #888 ;
--active- color : #f00 ;
}
* {
font-family : sans-serif ;
}
.likeBtn {
border : 2px solid var(--default-color);
display : flex;
align-items: center ;
gap: 8px ;
border-radius: 32px ;
cursor : pointer ;
font-weight : bold ;
height : 30px ;
padding : 4px 8px ;
margin-bottom : 5px ;
color : var(--default-color);
background-color : #fff ;
}
.likeBtn:hover {
border-color : var(--active-color);
color : var(--active-color);
}
.liked,
.liked:hover {
background-color : var(--active-color);
border-color : var(--active-color);
color : #fff ;
}
.likeBtn- icon {
display : flex;
}
.error {
font-size : 12px ;
}
|
Steps to run the application:
Step 1: Type the following command in the terminal.
npm run dev
Step 2: Open web browser and type the following URL.
http://localhost:5173/
Output:
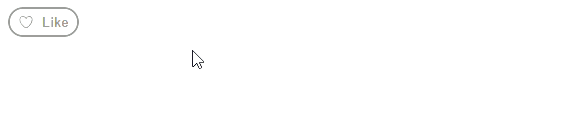
output
Share your thoughts in the comments
Please Login to comment...