How to change selected value of a drop-down list using jQuery?
Last Updated :
14 Dec, 2023
In this article, we are going to change the default selected value of the dropdown using jQuery. In an HTML select tag, the default selected value is the value given to the first option tag. With jQuery, it is easy to change the selected value from a drop-down list.
To achieve this feature you can use different methods, some of those methods are listed below:
By using the val() method
The val() method can be used by specifying a value attribute with some default values inside the option tags of the select element. Once you set the value attribute, you can now pass the value of the particular element that you want to replace with the default shown value as a parameter to the val() method in the form of a string.
Syntax:
$('element_selector').val('value');
Example: This example uses val() function to set the option corresponding to the passed value.
html
<!DOCTYPE html>
< html >
< head >
< title >
Change selected value
of a drop-down list
</ title >
< style >
div {
width:15%;
background-color:green;
padding:8px;
color:azure;
}
</ style >
< script src =
</ script >
< script type = "text/javascript" >
$(document).ready(()=>{
$("#select").val('2');
});
</ script >
</ head >
< body >
< div >
< p id = "gfg" >GeeksforGeeks courses:</ p >
< select id = "select" >
< option value = "0" >System Design</ option >
< option value = "1" >DSA-Online</ option >
< option value = "2" >Fork Python</ option >
< option value = "3" >Fork Java</ option >
</ select >
</ div >
</ body >
</ html >
|
Output:
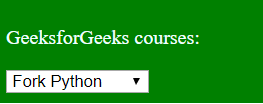
By using prop() method
You can also use the prop() method to change the value of a dropdown list. You can select the particular element using th element selector and use the selected property and set it to true.
Syntax:
$('element_selector').prop('selected', true);
Example: The below example uses the prop() method to change the value of the dropdown list:
HTML
<!DOCTYPE html>
< html >
< head >
< title >
Change selected value
of a drop-down list
</ title >
< style >
div {
width:15%;
background-color:green;
padding:8px;
color:azure;
}
</ style >
< script src =
</ script >
< script type = "text/javascript" >
$(document).ready(()=>{
$("#select option[value=1]").prop('selected', true);
});
</ script >
</ head >
< body >
< center >
< div >
< p id = "gfg" >GeeksforGeeks courses:</ p >
< select id = "select" >
< option value = "0" >System Design</ option >
< option value = "1" >DSA-Online</ option >
< option value = "2" >Fork Python</ option >
< option value = "3" >Fork Java</ option >
</ select >
</ div >
</ center >
</ body >
</ html >
|
Output:
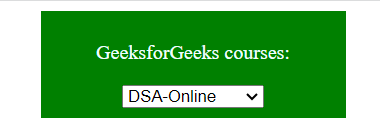
By using the attr() method
The attr() method can also be used to change the value of the dropdown list. You have to select the element and use the attr() method with selected attribute and set it to true to replace the value of dropdown with the selected value of the selected element.
Syntax:
$('selected_element').attr('selected', true)
Example: This example uses attr() function to assign the selected attribute to the corresponding option.
html
<!DOCTYPE html>
< html >
< head >
< title >
Change selected value
of a drop-down list
</ title >
< style >
div {
width:15%;
background-color:green;
padding:8px;
color:azure;
}
</ style >
< script src =
</ script >
< script type = "text/javascript" >
$(document).ready(()=>{
$("#select option[value=3]").attr('selected', true);
});
</ script >
</ head >
< body >
< div >
< p id = "gfg" >GeeksforGeeks courses:</ p >
< select id = "select" >
< option value = "0" >System Design</ option >
< option value = "1" >DSA-Online</ option >
< option value = "2" >Fork Python</ option >
< option value = "3" >Fork Java</ option >
</ select >
</ div >
</ body >
</ html >
|
Output:
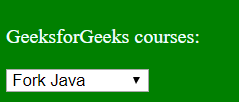
jQuery is an open source JavaScript library that simplifies the interactions between an HTML/CSS document, It is widely famous with it’s philosophy of “Write less, do more”. You can learn jQuery from the ground up by following this jQuery Tutorial and jQuery Examples.
Share your thoughts in the comments
Please Login to comment...