How to read a local text file using JavaScript?
Last Updated :
24 Nov, 2023
HTML 5 provides a standard way to interact with local files with the help of File API. The File API allows interaction with single, multiple as well and BLOB files.
The FileReader API can be used to read a file asynchronously in collaboration with JavaScript event handling. However, all the browsers do not have HTML 5 support so it is important to test the browser compatibility before using the File API.
There are four inbuilt methods in the FileReader API to read local files:
- FileReader.readAsArrayBuffer(): Reads the contents of the specified input file. The result attribute contains an ArrayBuffer representing the file’s data.
- FileReader.readAsBinaryString(): Reads the contents of the specified input file. The result attribute contains the raw binary data from the file as a string.
- FileReader.readAsDataURL(): Reads the contents of the specified input file. The result attribute contains a URL representing the file’s data.
- FileReader.readAsText(): Reads the contents of the specified input file. The result attribute contains the contents of the file as a text string. This method can take the encoding version as the second argument(if required). The default encoding is UTF-8.
Example 1: In this example, we are using the FileReader.readAsText() method to read the local file.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Read Text File</ title >
</ head >
< body >
< input type = "file" name = "inputfile" id = "inputfile" >
< br >
< pre id = "output" ></ pre >
< script type = "text/javascript" >
document.getElementById('inputfile')
.addEventListener('change', function () {
let fr = new FileReader();
fr.onload = function () {
document.getElementById('output')
.textContent = fr.result;
}
fr.readAsText(this.files[0]);
})
</ script >
</ body >
</ html >
|
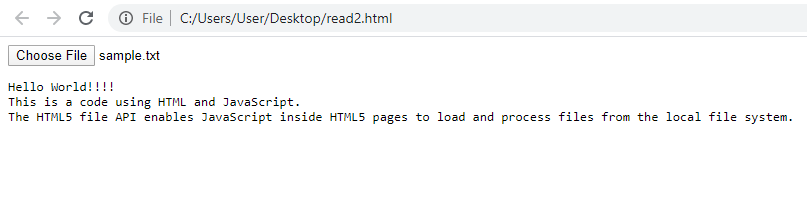
Example 2: In this example, we are using the FileReader.readAsBinaryString()method to read the local file.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Read Text File</ title >
</ head >
< body >
< input type = "file" name = "inputfile" id = "inputfile" >
< br >
< pre id = "output" ></ pre >
< script type = "text/javascript" >
document.getElementById('inputfile')
.addEventListener('change', function () {
let fr = new FileReader();
fr.onload = function () {
document.getElementById('output')
.textContent = fr.result;
}
fr.readAsBinaryString(this.files[0]);
})
</ script >
</ body >
</ html >
|
Output:
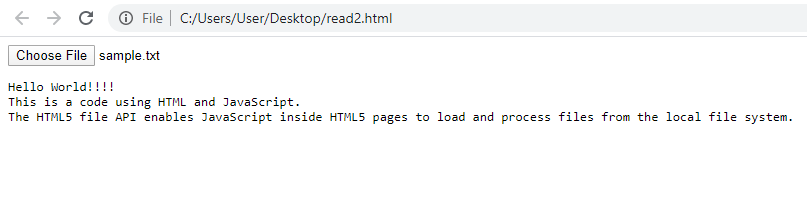
Output
JavaScript is best known for web page development but it is also used in a variety of non-browser environments. You can learn JavaScript from the ground up by following this JavaScript Tutorial and JavaScript Examples.
Share your thoughts in the comments
Please Login to comment...