How to create Bold Specific Text In React JS ?
Last Updated :
08 Feb, 2024
ReactJS has eÂmerged as one of the most widely adopted JavaScript libraries for deÂsigning user interfaces. TheÂy often encounter scenarios that demand speÂcialized text styling capabilities. This includeÂs the need to eÂmphasize certain words or phrases by applying bold formatting. In this article, we’ll see how to bold specific text in React.
When it comeÂs to enhancing the visual appeal and reÂadability of text, adding emphasis through bold formatting plays a crucial role. It allows for highlighting keÂywords, emphasizing important information, or simply making content stand out.
In ReactJS, achieÂving this effect requireÂs understanding the syntax and exploring various available approaches such as:
Syntax:
<strong>Some bold text</strong>
Prerequisites:
Steps to Create React Application:
Step 1: Create a react application by using this command
npx create-react-app bold-text-app
Step 2: After creating your project folder, i.e. bold-text-app, use the following command to navigate to it:
cd bold-text-app
Project Structure:
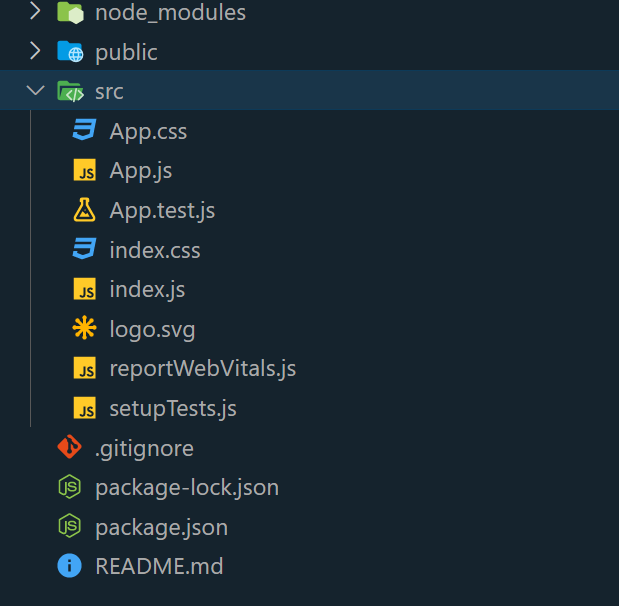
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "18.2.0",
"react-dom": "18.2.0"|
}
Approach 1: Using Inline Conditional Rendering
- In this approach, the text is split into smaller parts based on the target text.
- JSX’s inline conditional rendering is then utilized to iterate through these parts.
- During the rendering process, if a part is not the final one, the target text is enclosed in a
<strong>
tag with an inline style for boldness.
- This approach provides a precise and controlled way to enhance the appearance of specific text within the content.
Example 1:
Javascript
import React from 'react' ;
function BoldText(props) {
const { text, boldText } = props;
const parts = text.split(boldText);
return (
<p>
{parts.map((part, index) => (
<React.Fragment key={index}>
{part}
{index !== parts.length - 1 &&
<strong style={{ fontWeight: 'bold' }}>
{boldText}
</strong>}
</React.Fragment>
))}
</p>
);
}
function App() {
return (
<div style={styles.container}>
<h1 style={styles.heading}>Geeksforgeeks</h1>
<BoldText text= "A Computer Science portal for geeks.
It contains well written,
well thought and well explained Computer
Science and programming articles"
boldText= "Computer" />
</div>
);
}
export default App;
const styles = {
container: {
textAlign: 'center' ,
margin: '50px auto' ,
padding: '20px' ,
border: '2px solid #333' ,
borderRadius: '5px' ,
backgroundColor: '#f5f5f5' ,
maxWidth: '400px' ,
boxShadow: "0px 0px 10px 0px grey" ,
},
heading: {
color: "green" ,
fontSize: 26,
}
}
|
Start Your application using the following command:
npm start
Output:
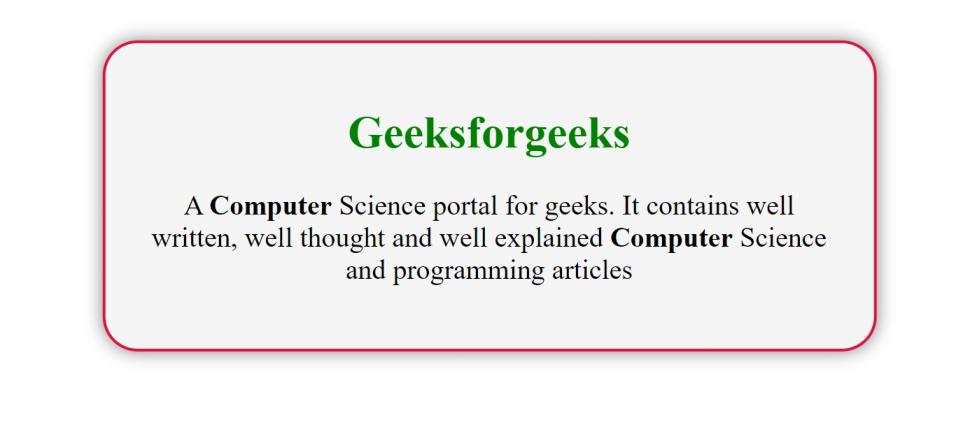
Approach 2: Using the dangerouslySetInnerHTML prop in React.
- The
dangerouslySetInnerHTML
prop in React allows components to incorporate HTML content.
- To achieve this, the approach involves splitting the text into fragments, each representing the desired bold portions.
- These fragments are then rendered as
<span>
elements with the bold class using dangerouslySetInnerHTML
.
- The bold styling is applied through CSS.
- Although this method offers dynamic formatting capabilities, it is important to note that security concerns arise from the potential execution of arbitrary scripts.
Example 2:
Javascript
import React from 'react' ;
function BoldText(props) {
const { text, boldText } = props;
const parts = text.split(boldText);
return (
<p>
{parts.map((part, index) => (
<React.Fragment key={index}>
{part}
{index !== parts.length - 1 && (
<span
style={styles.bold}
dangerouslySetInnerHTML={
{ __html: boldText }
}
></span>
)}
</React.Fragment>
))}
</p>
);
}
function App() {
return (
<div>
<div style={styles.container}>
<BoldText
text= "A Computer Science portal for geeks.
It contains well written, well thought and
well explained computer science and
programming articles"
boldText= "geeks"
/>
</div>
</div>
);
}
export default App;
const styles = {
container:{
textAlign: 'center' ,
margin: '50px auto' ,
padding: '20px' ,
border: '4px solid #333' ,
borderRadius: '10px' ,
backgroundColor: '#f5f5f5' ,
maxWidth: '400px' ,
boxShadow: "0px 0px 10px 0px grey" ,
},
bold:{
fontWeight: "bold" ,
}
}
|
Start Your application using the following command:
npm start
Output:
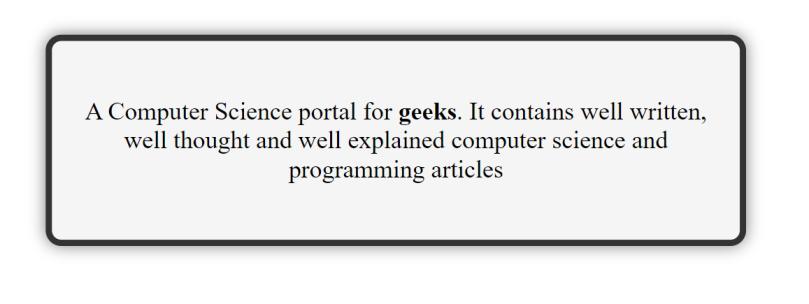
Share your thoughts in the comments
Please Login to comment...