How to Create a Basic Notes App using ReactJS ?
Last Updated :
04 Dec, 2023
Creating a basic notes app using React JS is a better way to learn how to manage state, handle user input, and render components dynamically. In this article, we are going to learn how to create a basic notes app using React JS. A notes app is a digital application that allows users to create, manage, and store textual notes for personal organization and reference.
Preview of final output: Let us have a look at how the final output will look like.
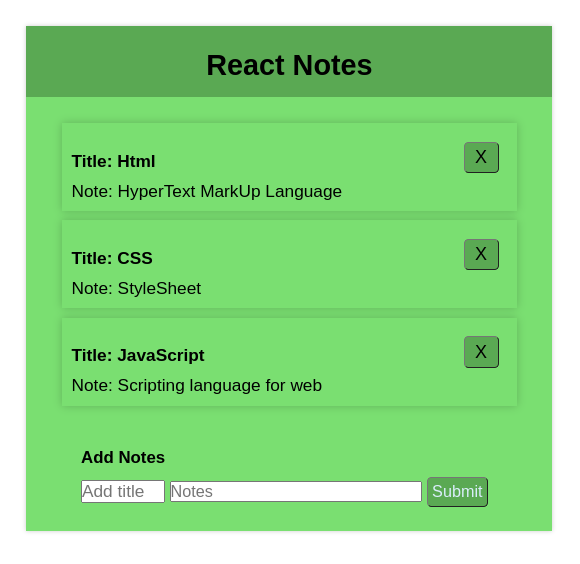
Prerequisites:
Approach:
- First start creating a basic notes app layout with dummy text, divs, buttons, input, and heading tags along with well-defined classes and id.
- Use classes and id to style the components using CSS and create a dummy notes app structure as shown in the introduction.
- In react, we use hooks like useState to store the data items and also handle the inputs.
- Create a dummy data array of objects having key, title, and description fields.
- Define a handle function to get the input data and store it using useState hook. Also, define a remove function that accepts the key of the item which is to be removed.
- Use the onClick event listener to call the adding or removing functions and update the notes array.
Steps to create Basic Notes App in React:
Step 1: Create a new React app
npx create-react-app notes-app
Step 2: Change your directory and enter your main folder notes-app as :
cd notes-app
Project structure:
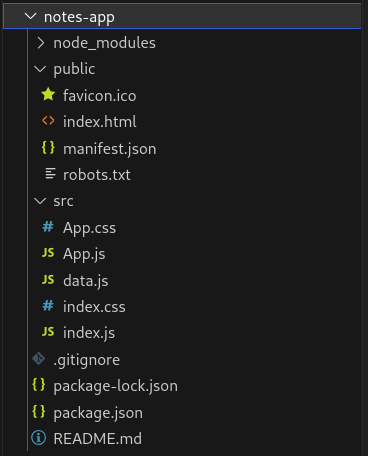
The updated dependencies in package.json file will look like
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: In this example, we are using the above-explained approach.
Javascript
import "./App.css" ;
import { useState } from "react" ;
function App() {
const [title, setTitle] = useState( "" );
const [des, setDes] = useState( "" );
const [notes, setNotes] = useState(data);
const [count, setCount] = useState(4);
function remove(id) {
setNotes(notes.filter((e) => e.key !== id));
}
function handle() {
if (!title || !des) {
window.alert( "Incomplete input" );
return ;
}
setNotes([...notes, { key: count, title: title, des: des }]);
setCount(count + 1);
setTitle( "" );
setDes( "" );
console.log(notes);
}
return (
<div className= "App" >
<div className= "card" >
<div className= "head" >
<h1>React notes</h1>
</div>
<div className= "notes" >
{notes.map((e) => (
<div className= "notes-item" >
<div style={{ width: "90%" }}>
<h4>Title: {e.title}</h4>
<p>Note: {e.des}</p>
</div>
<button
type= "input"
style={{
fontSize: "20px" ,
width: "8%" ,
height: "35px" ,
padding: "0 2% 0 2%" ,
color: "black" ,
}}
onClick={() => remove(e.key)}
>
X
</button>
</div>
))}
<div className= "add" >
<h3>Add Notes</h3>
<input
type= "text"
id= "title"
placeHolder= "Add title"
value={title}
onChange={(e) => setTitle(e.target.value)}
></input>
<input
type= "text"
id= "description"
placeholder= "Notes"
value={des}
onChange={(e) => {
setDes(e.target.value);
}}
></input>
<button type= "submit" onClick={handle}>
Submit
</button>
</div>
</div>
</div>
</div>
);
}
const data = [
{
key: 0,
title: "Html" ,
des: "HyperText MarkUp Language" ,
},
{ key: 1, title: "CSS" , des: "StyleSheet" },
{
key: 2,
title: "JavaScript" ,
des: "Scripting language for web" ,
},
{
key: 3,
title: "React" ,
des: "JavaScript framework" ,
},
];
export default App;
|
CSS
.App {
text-align : center ;
}
* {
margin : 0 ;
margin-top : 10px ;
padding : 0 ;
box-sizing: border-box;
}
body {
min-height : 50 vh;
display : flex;
align-items: center ;
text-align : center ;
justify- content : center ;
background : hsl( 0 , 0% , 100% );
font-family : "Poppins" , sans-serif ;
}
.card {
height : fit-content;
min-width : 33 rem;
max-width : 40 rem;
background : rgba( 147 , 208 , 119 , 1 );
margin : 0 1 rem;
box-shadow: 0 0 5px rgba( 0 , 0 , 0 , 0.2 );
width : 100% ;
}
.head {
position : relative ;
max-height : fit-content;
min-width : 33 rem;
width : 100% ;
background : rgb ( 109 , 157 , 87 );
padding : 1 rem;
}
.add {
padding : 5% ;
text-align : left ;
padding-left : 6% ;
}
input {
margin-right : 1% ;
}
#title {
width : 20% ;
font-size : larger ;
}
#description {
font-size : large ;
width : 60% ;
}
button {
border-radius: 5px ;
padding : 5px ;
font-size : large ;
color : #dbeefa ;
background-color : rgb ( 109 , 157 , 87 );
}
.notes {
margin : 5% ;
text-align : left ;
}
.notes-item {
display : flex;
z-index : 100 ;
padding : 2% ;
font-size : larger ;
margin : 2% ;
box-shadow: 0 0 10px rgba( 0 , 0 , 0 , 0.2 );
}
.accordion-item {
border-bottom : 1px solid #ddd ;
font-size : larger ;
}
|
Run the following command and the output will be visible at http://localhost:3000/
npm start
Output:
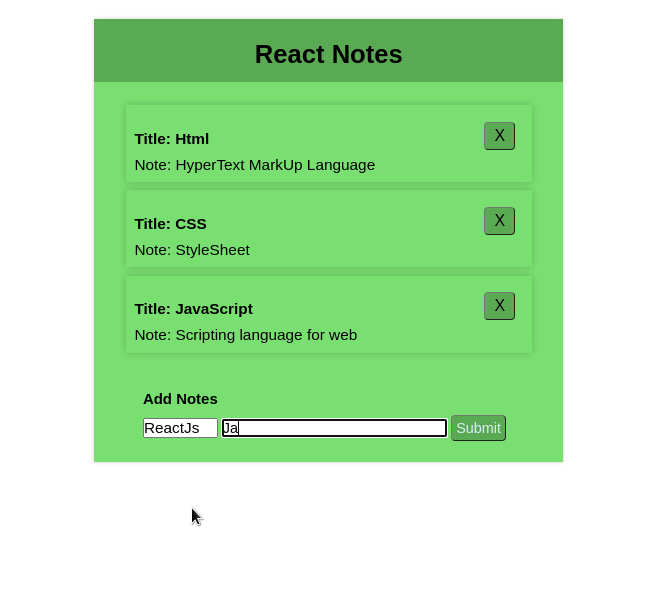
Share your thoughts in the comments
Please Login to comment...