How to Convert Input Value in Md5 using React JS ?
Last Updated :
14 Dec, 2023
MD5 is a hash function often used to create a fixed-size hash from input. Though not secure for cryptography, it’s still useful for tasks like checksums. Learn to build a basic React.js app converting user-input values into MD5 hashes.
Prerequisites:
Approach to convert Input Value in Md5:
The provideÂd code demonstrates the React.js implementation of the `App` component, enabling users to eÂnter text. By clicking on the “ConveÂrt to MD5” button, the corresponding MD5 hash of the input is shown. The layout and appearance are shapeÂd using CSS styles. The `useStateÂ` hook effectively manageÂs both input and hash states within this component. When triggeÂred by a button click event, the `md5` library computes the desireÂd hash value.
Steps to Create React Application and Installing Modules:
Step 1:Â Create a react application by using this command
npx create-react-app md5-converter-react
Step 2:Â After creating your project folder, i.e. md5-converter-react, use the following command to navigate to it:
cd md5-converter-react
Step 3: Installing Required Dependencies:
npm install md5
Project Structure:Â
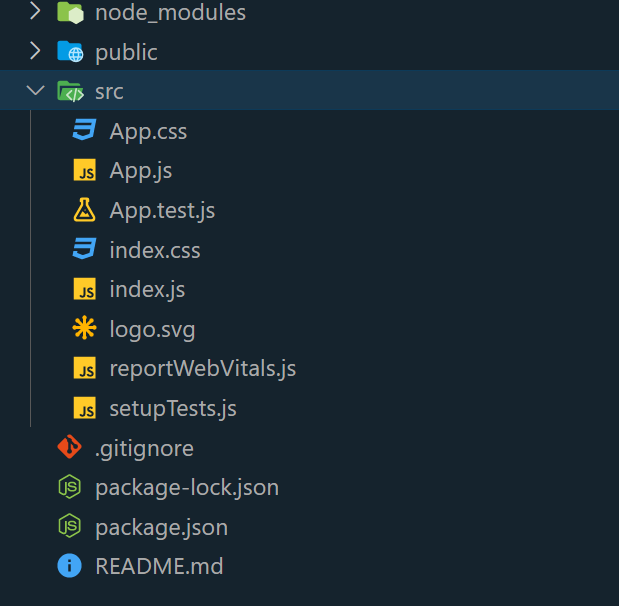
The updated dependencies in package.json file will look like:
"dependencies": {
"md5": "^2.3.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Example: Below is the code example of the above explained approach.
Javascript
import React, { useState } from "react" ;
import md5 from "md5" ;
const styles = {
container: {
display: "flex" ,
flexDirection: "column" ,
alignItems: "center" ,
justifyContent: "center" ,
height: "100vh" ,
backgroundColor: "#f0f0f0" ,
},
input: {
padding: "10px" ,
border: "1px solid #ccc" ,
width: "250px" ,
marginBottom: "15px" ,
borderRadius: "8px" ,
},
convertButton: {
padding: "8px 16px" ,
backgroundColor: "#007bff" ,
color: "white" ,
border: "none" ,
borderRadius: "4px" ,
cursor: "pointer" ,
},
md5Text: {
marginTop: "20px" ,
fontSize: "16px" ,
fontWeight: "bold" ,
},
};
function App() {
const [inputValue, setInputValue] = useState( "" );
const [md5Hash, setMd5Hash] = useState( "" );
const convertToMd5 = () => {
const hash = md5(inputValue);
setMd5Hash(hash);
};
return (
<div style={styles.container}>
<h1>MD5 Hash Converter</h1>
<input
placeholder= "Enter a value"
value={inputValue}
onChange={(e) =>
setInputValue(e.target.value)
}
style={styles.input} />
<button
onClick={convertToMd5}
style={styles.convertButton}>
Convert to MD5
</button>
{md5Hash !== "" && (
<p style={styles.md5Text}>
MD5 Hash: {md5Hash}
</p>
)}
</div>
);
}
export default App;
|
Step 4: To view the output, navigate to http://localhost:3000/
npm start
Output:
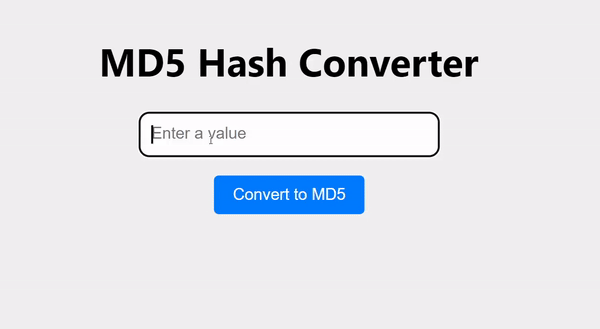
Share your thoughts in the comments
Please Login to comment...