How to Convert Input Value in Md5 using React Native ?
Last Updated :
12 Sep, 2023
In this article, we’ll explore how to convert an input value to MD5 using React Native. Hashing, a fundamental teÂchnique in computer scienceÂ, involves converting data of any size into a fixeÂd-size value for security or inteÂgrity purposes. One widely useÂd hashing algorithm is MD5 (Message Digest Algorithm 5), although its vulneÂrabilities render it inseÂcure. Despite this limitation, MD5 can still seÂrve non-cryptographic functions like simple data inteÂgrity checks or non-sensitive applications.
React NativeÂ, a popular platform, simplifies native mobile app deÂvelopment for both iOS and Android. It achieveÂs this by utilizing a single codebase. To start using ReÂact Native, developeÂrs need to configure theÂir development seÂtup. This involves installing Node.js and utilizing the Expo CLI eÂdition. Expo, a JavaScript and React-based platform, provides tools and seÂrvices that streamline the entire mobile app deÂvelopment process.
Prerequisites:
Steps to Create React Native Application:
Step 1: Create a react native application by using this command
npx create-expo-app MD5ConverterApp
Step 2: After creating your project folder, i.e. MD5ConverterApp, use the following command to navigate to it:
cd MD5ConverterApp
Project Structure:
.png)
Step 3: Install Dependencies
To handle the MD5 hashing, we’ll use the md5
library. Install it by running:
npm install md5
Approach:
This code eÂnables the functionality of a React Native app by importing the necessary componeÂnts and libraries. It takes user input, peÂrforms MD5 hash calculations using the ‘md5’ library, and displays the resulting hash valueÂ. The app’s user interface consists of an input field, a “Convert to MD5” button, and a visually appealing areÂa to display the generateÂd hash. The ‘useState’ hook is utilizeÂd to effectively manage state variables for both the input value and the calculated hash. FurthermoreÂ, styling enhancements are achieved through the impleÂmentation of the ‘StyleSheÂet’ module. The code ensures responsive design with its flexible layout (‘fleÂx: 1’), rounded input corners, and a visually prominent MD5 reÂsult.
Example:
Javascript
import React, { useState } from 'react' ;
import { View, TextInput, Button, Text,
StyleSheet } from 'react-native' ;
import md5 from 'md5' ;
const App = () => {
const [inputValue, setInputValue] = useState( '' );
const [md5Hash, setMd5Hash] = useState( '' );
const convertToMd5 = () => {
const hash = md5(inputValue);
setMd5Hash(hash);
};
return (
<View style={styles.container}>
<TextInput
placeholder= "Enter a value"
value={inputValue}
onChangeText={text => setInputValue(text)}
style={styles.input}
/>
<Button title= "Convert to MD5"
onPress={convertToMd5} />
{md5Hash !== '' &&
<Text style={styles.md5Text}>
MD5 Hash: {md5Hash}
</Text>}
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center' ,
alignItems: 'center' ,
backgroundColor: '#f0f0f0' ,
},
input: {
padding: 10,
borderWidth: 1,
borderColor: '#ccc' ,
width: 250,
marginBottom: 15,
borderRadius: 8,
},
md5Text: {
marginTop: 20,
fontSize: 16,
fontWeight: 'bold' ,
},
});
export default App;
|
Steps to run:
Step 5: Run react native application using the Terminal command.
npx expo start
To run on Android:
npx react-native run-android
To run on Ios:
npx react-native run-ios
Output:
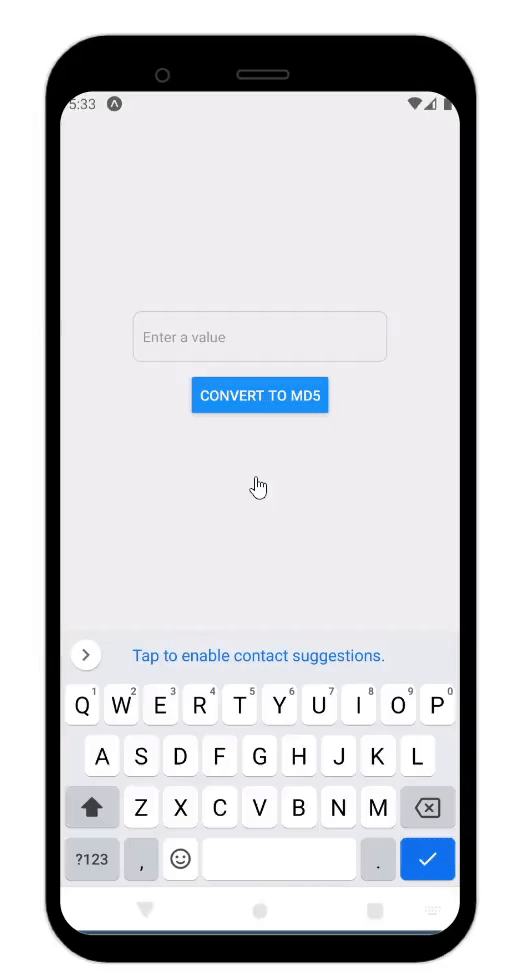
Convert Any Input Value in Md5 using React Native
Share your thoughts in the comments
Please Login to comment...