How to Change the Color of a Link in ReactJS ?
Last Updated :
06 Dec, 2023
In this article, we’ll see how we can change the color of a link in ReactJS. To change a link’s color in React, add a CSS style to the link element using the style attribute in JSX code.
Default link styles in HTML and CSS often include blue color and underlining. However, they may not align with your React app’s design. You might need to change the link color to match your app’s theme, and branding, or differentiate link states.
Syntax:
<a href="#"> Home </a>
Prerequisites:
Approaches to change the color of link in React JS are:
Steps to Create React Application:
Step 1: Create a react application by using this command
npx create-react-app change-color-project
Step 2: After creating your project folder, i.e. change-color-project, use the following command to navigate to it:
cd change-color-project
Project Structure
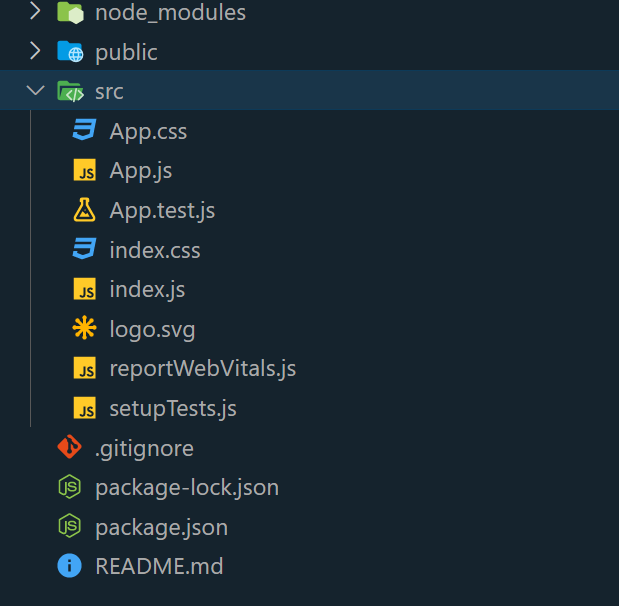
Â
To change the color of a link in React using CSS modules, create a CSS file with a unique class name, define the desired color property for that class, and import it into your React component.
CSS modules modularize CSS in React by defining styles in a separate file and importing them into the component.
Example: This example use CSS to change the color of links on hover.
Javascript
import React from "react" ;
import "./style.css" ;
export default function App() {
return (
<nav className= "navbar" >
<ul className= "navMenu" >
<li>
<a href= "#" className= "link" >
Home
</a>
</li>
<li>
<a href= "#" className= "link" >
About
</a>
</li>
<li>
<a href= "#" className= "link" >
Contact
</a>
</li>
</ul>
</nav>
);
}
|
CSS
.navbar {
background-color : #333 ;
display : flex;
justify- content : space-between;
align-items: center ;
padding : 10px ;
}
.navMenu {
list-style : none ;
display : flex;
}
.link {
color : #fff ;
text-decoration : none ;
padding : 10px ;
font-size : 1.4 rem;
}
.link:hover {
color : red ;
}
|
Step to Run the Application: To Run Application: Open the terminal and type the following command.
npm start
Output: This output will be visible on the http://localhost:3000/ on the browser window.
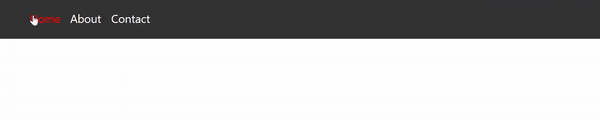
To change the color of a link in React using styled-components and import the styled function from the styled-components library. Create a styled component using the styled function and define the desired styles,Â
Styled components in React let you write CSS-in-JS, where a single component can contain both the HTML markup and the corresponding CSS.
Step to Install Module:
npm i styled-components
The updated dependencies in package.json file will look like.
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"styled-components": "^6.1.0",
"web-vitals": "^2.1.4"
}
Example: This example is the same as the previous but the difference is that in the previous example, we use CSS modules but in this example, we used styled components. If you got an error like Can’t resolve ‘styled-components’. Install the styled-components package by using the command:Â
Javascript
import React from "react" ;
import styled from "styled-components" ;
const Navbar = styled.nav`
background-color: #333;
display: flex;
justify-content: space-between;
align-items: center;
padding: 10px;
font-size:1.4rem;
`;
const NavbarLinks = styled.ul`
list-style: none;
display: flex;
`;
const NavbarLink = styled.a`
color: #fff;
text-decoration: none;
padding: 10px;
&:hover {
color: red;
}
`;
export default function App() {
return (
<div>
<Navbar>
<NavbarLinks>
<li>
<NavbarLink href= "#" >
Home
</NavbarLink>
</li>
<li>
<NavbarLink href= "#" >
About
</NavbarLink>
</li>
<li>
<NavbarLink href= "#" >
Contact
</NavbarLink>
</li>
</NavbarLinks>
</Navbar>
</div>
);
}
|
Step to Run the Application: To Run Application: Open the terminal and type the following command.
npm start
Output: This output will be visible on the http://localhost:3000/ on the browser window.
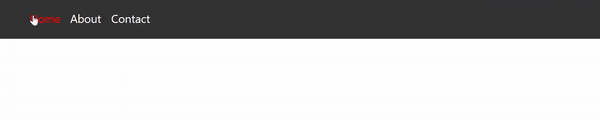
Share your thoughts in the comments
Please Login to comment...