How to Center an Element in jQuery ?
Last Updated :
14 Feb, 2024
Centering elements in a webpage is a common task for web developers, often involving CSS. However, certain scenarios might require dynamically centering elements using JavaScript or jQuery, especially when dealing with dynamic content sizes or responsive designs. jQuery, a fast, small, and feature-rich JavaScript library, simplifies the process of HTML document traversing, event handling, animating, and Ajax interactions for rapid web development. In this article, we will explore how to center an element horizontally, vertically, or both, using jQuery.
Prerequisites
To follow along with these examples, ensure you have jQuery included in your project. You can include jQuery by adding the following script tag in the <head> section of your HTML document:
<script src=”https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js”></script>
Centering an Element Horizontally
To center an element horizontally within its parent container, you can set the element’s left margin to auto. This method works well when the element’s display property is set to block.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< script src =
</ script >
< title >Centering an Element Horizontally</ title >
< style >
.container {
width: 100%;
text-align: center;
}
#centerElement {
display: inline-block;
margin: 0 auto;
}
</ style >
</ head >
< body >
< div class = "container" >
< div id = "centerElement" >
Welcome to GeeksforGeeks
</ div >
</ div >
< script >
$(document).ready(function () {
$('#centerElement').css({
'display': 'inline-block',
'margin-left': 'auto',
'margin-right': 'auto'
});
});
</ script >
</ body >
</ html >
|
Output

Centering an Element Vertically
Centering an element vertically requires calculating the top margin based on the parent’s height and the element’s height. This method can be particularly useful for centering an element within the viewport or a div that has a variable height.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< script src =
</ script >
< title >Centering an Element Vertically</ title >
< style >
.container {
position: relative;
height: 100vh;
}
#centerElement {
position: absolute;
}
</ style >
</ head >
< body >
< div class = "container" >
< div id = "centerElement" >
Welcome to GeeksforGeeks
</ div >
</ div >
< script >
$(document).ready(function () {
var containerHeight = $('.container').height();
var elementHeight = $('#centerElement').outerHeight();
var marginTop = (containerHeight - elementHeight) / 2;
$('#centerElement').css('top', marginTop + 'px');
});
</ script >
</ body >
</ html >
|
Output
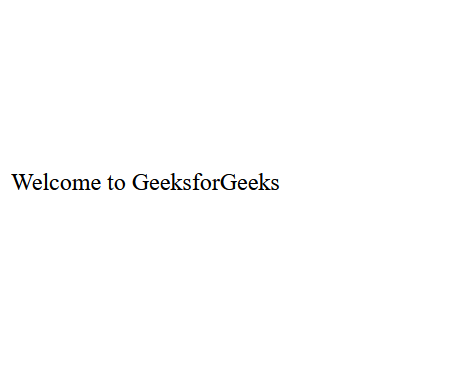
Centering an Element Both Horizontally and Vertically
Combining the methods from the first two examples, you can center an element both horizontally and vertically within its parent.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< script src =
</ script >
< title >Centering an Element Vertically</ title >
< style >
.container {
position: relative;
width: 100%;
height: 100vh;
}
#centerElement {
position: absolute;
display: inline-block;
}
</ style >
</ head >
< body >
< div class = "container" >
< div id = "centerElement" >
Welcome to GeeksforGeeks
</ div >
</ div >
< script >
$(document).ready(function () {
var containerHeight = $('.container').height();
var containerWidth = $('.container').width();
var elementHeight = $('#centerElement').outerHeight();
var elementWidth = $('#centerElement').outerWidth();
var marginTop = (containerHeight - elementHeight) / 2;
var marginLeft = (containerWidth - elementWidth) / 2;
$('#centerElement').css({
'top': marginTop + 'px',
'left': marginLeft + 'px'
});
});
</ script >
</ body >
</ html >
|
Output
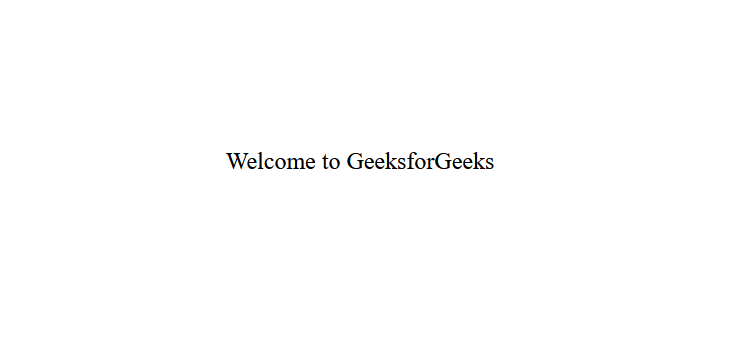
Share your thoughts in the comments
Please Login to comment...