How to Add Input Field inside Table Cell in HTML ?
Last Updated :
18 Mar, 2024
In HTML, adding input fields inside the table cells can be done to make the application user interactive and useful in the scenarios of building forms, data entry systems, etc.
Below are the approaches to add input field inside a Table Cell in HTML:
In this approach, we are using the <input> tag directly within table cells to allow users to input values. This enables the creation of editable fields for specific data entries, such as names and ages, directly within the table structure.
Syntax:
<tr>
<td><input type="text" placeholder="Enter value"></td>
<td>Data</td>
</tr>
Example: The below example uses the <input> tag Directly to Add an input field inside the table cell in HTML.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 1</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
margin: 50px;
background-color: #f8f8f8;
color: #333;
}
h1 {
color: #4CAF50;
}
h3 {
margin-bottom: 20px;
}
table {
border-collapse: collapse;
width: 50%;
margin: 20px auto;
}
th,
td {
padding: 10px;
border: 1px solid #ddd;
text-align: left;
}
th {
background-color: #4CAF50;
color: white;
}
input {
padding: 8px;
width: 80%;
box-sizing: border-box;
}
</style>
</head>
<body>
<h1>GeeksforGeeks</h1>
<h3>Using <input> tag Directly</h3>
<table>
<tr>
<th>Name</th>
<th>Age</th>
</tr>
<tr>
<td>
<input type="text"
placeholder="Enter value">
</td>
<td>23</td>
</tr>
<tr>
<td>Gaurav</td>
<td>
<input type="text"
placeholder="Enter value">
</td>
</tr>
</table>
</body>
</html>
Output:
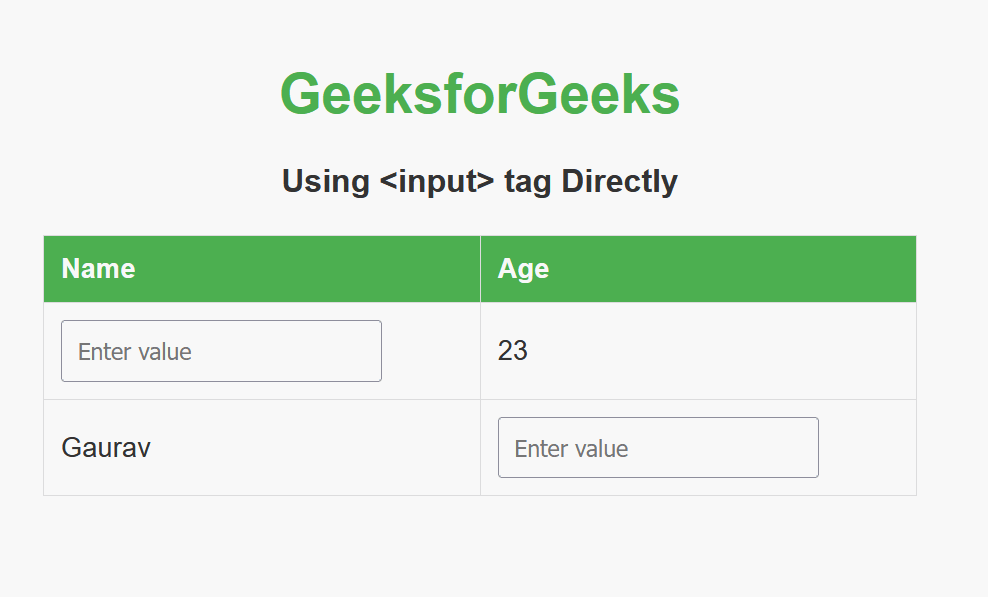
Using data Attribute with JavaScript
In this approach, we are using the data attribute (data-value) in HTML along with JavaScript to dynamically replace specific table cells with input fields. The script, executed when the DOM content is loaded, selects cells with the specified data attribute, creates an input element, initializes its value from the data attribute, and then replaces the original content with the input field.
Syntax:
<tr>
<td data-value=""></td>
<td>Data</td>
</tr>
<script>
// JavaScript code to create an input element using data attribute
</script>
Example: The below example uses data Attribute with JavaScript to Add input field inside table cell in HTML.
HTML
<!DOCTYPE html>
<html>
<head>
<style>
table {
border-collapse: collapse;
width: 60%;
}
th,
td {
border: 1px solid black;
padding: 8px;
text-align: left;
}
h1 {
color: green;
}
</style>
<title>Example 2</title>
</head>
<body>
<h1>GeeksforGeeks</h1>
<h3>Using data Attribute with JavaScript</h3>
<table>
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
</tr>
<tr>
<td>1</td>
<td data-value=""></td>
<td>gfg@gmail.com</td>
</tr>
</table>
<script>
document.addEventListener(
'DOMContentLoaded',
() => {
let cells =
document.querySelectorAll('td[data-value]');
cells.forEach(function (cell) {
let input =
document.createElement('input');
input.type = 'text';
input.value = cell.dataset.value;
cell.innerHTML = '';
cell.appendChild(input);
});
});
</script>
</body>
</html>
Output:
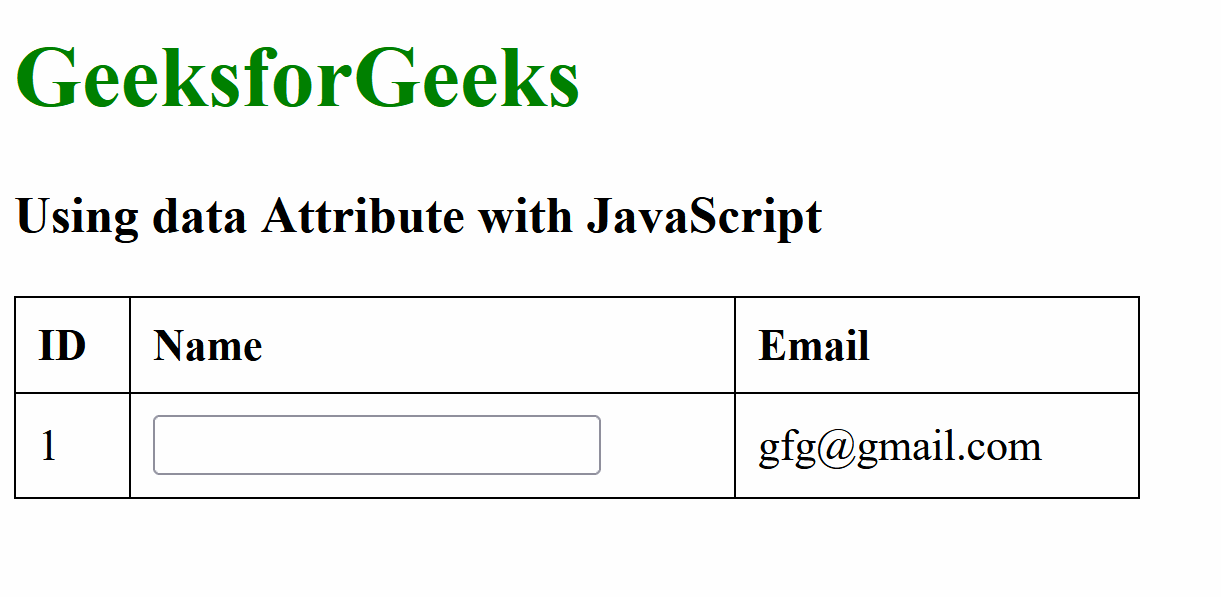
Share your thoughts in the comments
Please Login to comment...