How to Insert a Row in an HTML Table in JavaScript ?
Last Updated :
21 Mar, 2024
In JavaScript, we can insert a row dynamically in the HTML table using various approaches. JavaScript provides us with different methods to accomplish this task as listed below.
Using insertRow() and insertCell()
In this approach, we are using the insertRow() and insertCell() methods in JavaScript to dynamically insert a new row into an HTML table.
Syntax:
tableObject.insertRow(index)
tablerowObject.insertCell(index)
Example: The below example uses insertRow() and insertCell() to insert a row in an HTML table in JavaScript.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 1</title>
<style>
body {
text-align: center;
font-family: Arial, sans-serif;
}
h3 {
margin-bottom: 20px;
}
table {
margin: 0 auto;
border-collapse: collapse;
}
td,
th {
border: 1px solid black;
padding: 8px;
}
</style>
</head>
<body>
<h3>
Using insertRow() and insertCell()
<br/> to add a new row in HTML table.
</h3>
<table id="tab">
<thead>
<tr>
<th>Subject</th>
<th>Marks</th>
</tr>
</thead>
<tbody>
<tr>
<td>Python</td>
<td>56</td>
</tr>
<tr>
<td>JS</td>
<td>60</td>
</tr>
</tbody>
</table>
<br/>
<button onclick="insertFn()">
Insert Row
</button>
<script>
function insertFn() {
const table = document.getElementById("tab").
getElementsByTagName('tbody')[0];
const newRow = table.insertRow();
const cell1 = newRow.insertCell(0);
const cell2 = newRow.insertCell(1);
cell1.innerHTML = "CSS";
cell2.innerHTML = "67";
}
</script>
</body>
</html>
Output:
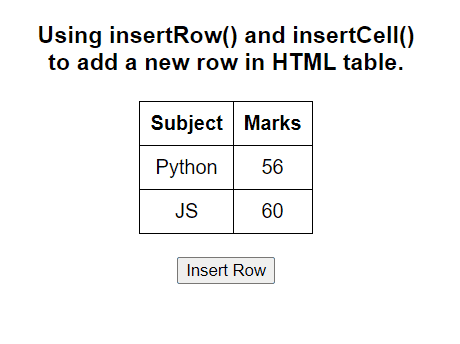
Using insertAdjacentHTML()
In this approach, we are using the insertAdjacentHTML() method in JavaScript to dynamically insert a rown in the HTML table.
Syntax:
node.insertAdjacentHTML(specify-position, text-to-enter)
Example: The below example uses insertAdjacentHTML() to insert a row in an HTML table using JavaScript.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 2</title>
<style>
body {
text-align: center;
font-family: Arial, sans-serif;
}
h3 {
margin-bottom: 20px;
}
table {
margin: 0 auto;
border-collapse: collapse;
}
td,
th {
border: 1px solid rgb(255, 0, 0);
padding: 8px;
}
</style>
</head>
<body>
<h3>
Using insertAdjacentHTML() to insert a Row
<br/> dynamically inside an HTML table.
</h3>
<table id="tab">
<thead>
<tr>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<tr>
<td>GFG 1</td>
<td>30</td>
</tr>
<tr>
<td>GFG 2</td>
<td>25</td>
</tr>
</tbody>
</table>
<br/>
<button onclick="insertFn()">
Insert Row
</button>
<script>
function insertFn() {
const newRow =
`<tr>
<td>GFG 3</td>
<td>34</td>
</tr>`;
document.getElementById('tab').
getElementsByTagName('tbody')[0].
insertAdjacentHTML('beforeend', newRow);
}
</script>
</body>
</html>
Output:
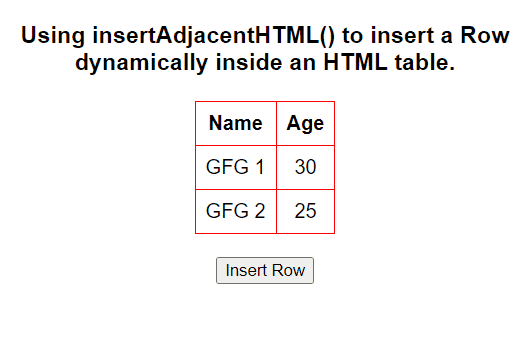
Share your thoughts in the comments
Please Login to comment...