How to Add Button Inside an Input Field in HTML ?
Last Updated :
14 Feb, 2024
Integrating a button inside an input box is a common design pattern in web development, often seen in search bars, where a “search” button sits within the text input field, or in password fields that include a “show/hide” toggle. This UI element enhances user experience by offering a clear, interactive way to perform actions directly from the input field. Achieving this layout involves a combination of HTML, CSS, and sometimes JavaScript, depending on the functionality required.
Method 1: Using HTML and CSS
The simplest way to place a button inside an input box is by wrapping both elements within a parent div and using CSS to position the button over the input field.
Example: Basic Implementation to add button in input field.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >Input with Button</ title >
< style >
.input-wrapper {
position: relative;
display: inline-block;
}
.input-wrapper input {
padding-right: 40px;
}
.input-wrapper button {
position: absolute;
top: 0;
right: 0;
border: none;
background-color: transparent;
cursor: pointer;
}
</ style >
</ head >
< body >
< div class = "input-wrapper" >
< input type = "text" placeholder = "Search..." >
< button onclick = "alert('Button clicked!');" >
🔍
</ button >
</ div >
</ body >
</ html >
|
Output
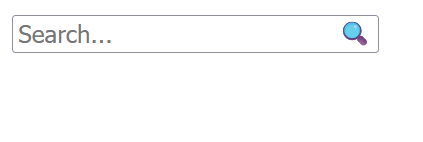
In this example, the button is absolutely positioned within the input-wrapper container, aligning it to the right of the input field. The padding on the right side of the input ensures that text entered by the user does not overlap with the button.
Method 2: Advanced Customization with Icons and JavaScript
For a more advanced implementation, such as adding an icon inside the input field that toggles the input type (e.g., for a password field), you might use Font Awesome for the icon and JavaScript for the toggle functionality.
Example: Show/Hide Password Toggle
First, include Font Awesome for the icons:
<link rel=”stylesheet” href=”https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css”>
Then, add the HTML structure with embedded JavaScript:
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >Show/Hide Password</ title >
< link rel = "stylesheet" href =
< style >
.input-wrapper {
position: relative;
display: inline-block;
}
.input-wrapper input {
padding-right: 30px;
}
.toggle-password {
position: absolute;
top: 50%;
right: 10px;
transform: translateY(-50%);
border: none;
background: none;
cursor: pointer;
}
</ style >
</ head >
< body >
< div class = "input-wrapper" >
< input id = "password" type = "password"
placeholder = "Password" >
< button class = "toggle-password"
onclick = "togglePasswordVisibility()" >
< i id = "toggleIcon" class = "fas fa-eye" ></ i >
</ button >
</ div >
< script >
function togglePasswordVisibility() {
var passwordInput = document.getElementById('password');
var toggleIcon = document.getElementById('toggleIcon');
if (passwordInput.type === "password") {
passwordInput.type = "text";
toggleIcon.classList.remove('fa-eye');
toggleIcon.classList.add('fa-eye-slash');
} else {
passwordInput.type = "password";
toggleIcon.classList.remove('fa-eye-slash');
toggleIcon.classList.add('fa-eye');
}
}
</ script >
</ body >
</ html >
|
Output
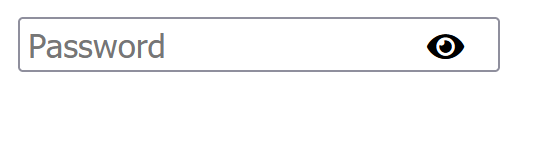
This setup offers an interactive user experience by allowing the user to toggle the visibility of their password input.
Share your thoughts in the comments
Please Login to comment...