Why would you use a Higher-Order Component in React ?
Last Updated :
18 Mar, 2024
A Higher-Order Component (HOC) in React is used to share common functionality between multiple components. It’s like a function that takes a component as an argument and returns a new enhanced component with added features.
By offering a pattern for reusing component logic, HOCs help in crafting more maintainable and cleaner React applications. This article simplifies the concept of HOCs, showcases their benefits, and walks through an example to highlight their practical application.
Steps to Create React Application:
Step 1: Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Step 3: After setting up react environment on your system, we can start by creating an App.js file and create a file by the name of components in which we will write our desired function.
Project Structure:
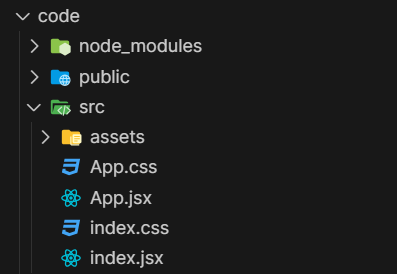
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Approach to implement Higher Order Component in React:
- First define a function that accepts a component as its argument.
- Inside the HOC, create a new component class or function that wraps the input component.
- Implement any additional logic or state that you want the original component to have.
- Return the enhanced component from the HOC function.
Example: Illustration to showcase the use of Higher Order Component in react.
JavaScript
// App.js
import React from 'react';
// Higher-Order Component
const withHoverEffect = (WrappedComponent) => {
return class extends React.Component {
state = {
isHovered: false,
};
handleMouseEnter = () => {
this.setState({ isHovered: true });
};
handleMouseLeave = () => {
this.setState({ isHovered: false });
};
render() {
return (
<div
onMouseEnter={this.handleMouseEnter}
onMouseLeave={this.handleMouseLeave}
>
<WrappedComponent {...this.props}
isHovered={this.state.isHovered} />
</div>
);
}
};
};
// Component without hover effect
const Button = ({ isHovered, onClick }) => {
return (
<button style={{ backgroundColor: isHovered ? 'white' : 'red' }}
onClick={onClick}>
Click Me
</button>
);
};
// Enhance Button component with hover effect using HOC
const ButtonWithHoverEffect = withHoverEffect(Button);
const App = () => {
const handleClick = () => {
alert('Button clicked!');
};
return (
<div>
<h1>Example to showcase <br></br> Higher-Order Component</h1>
<ButtonWithHoverEffect onClick={handleClick} />
</div>
);
};
export default App;
Output:
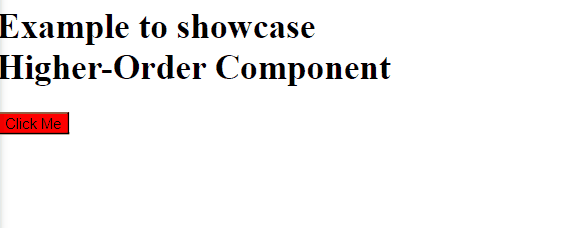
Benefits of Using Higher-Order Components:
- Code Reusability: Simplifies sharing common functionality across components.
- Separation of Concerns: Helps in separating cross-cutting concerns like logging or data fetching from the main component logic.
- Composition: Enables combining multiple HOCs to enhance a component in a modular way.
- Flexibility: Offers a way to add features to components without altering their original code.
- Easier Testing: Makes it simpler to test behaviors isolated by HOCs since the core component remains unaltered.
Conclusion:
Higher-Order Components provide a versatile method for enhancing and reusing component logic in React applications. By understanding how to effectively use HOCs, developers can build applications that are modular, easy to maintain, and adaptable to changing requirements.
Share your thoughts in the comments
Please Login to comment...