How a View-model works in Vue.js?
Last Updated :
15 Feb, 2023
Vue.js is a front-end progressive javascript framework for building User Interfaces ( UI ) and Single Page Applications. This framework focuses on Model – View – ViewModel architecture pattern that connects the View ( User Interface ) and the Model ( Data Objects ). Vue.js uses many built-in directives for interacting between View and Model. It helps to manipulate/modify the DOM with help of Vue instances. The v-model is a special directive that helps to create reactive objects with Vue instances to interact between Model and View layers for changing data properties in the DOM. In this article, we will see how a View-model Works In Vue.js.
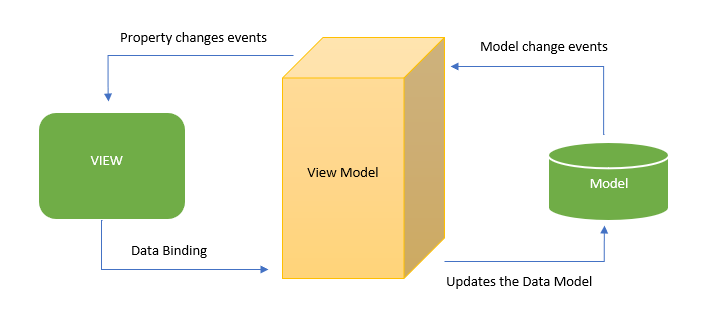
Model–View–ViewModel
View-model has the following components:
- View is a Presentation layer the User Interface represents the structure of DOM. The Document Object Model (DOM) is managed by Vue instances. It works as a template in the ViewModel layer.
Syntax:
<div>
<p> {{ name }} </p>
<div>
- Model is a Business-Logic Layer containing plain JavaScript objects or Data model objects that become reactive when created by Vue instances. whenever objects changed, the View also updated simultaneously.
Syntax:
data : { name: "geeksforgeeks" }
- View-Model layer that projects and transforms Model objects for state changes. View layer listens to that property changes and renders in DOM. It is a bridge between the Model and View for creating Vue instance with synchronized objects. Vue directives are used to help Data manipulations and DOM listeners in Vue instances. Vue instances created by Vue() Constructor.
Syntax:
<script>
const app = new Vue( { /*.....Connect the View and Model Here.....*/ } );
</script>
The following Vue directives are used:
- v-model: This directive is used to create two-way data bindings on form input, text area, etc.
- v-bind: This directive is used to manipulate HTML attributes, change the style, and assign classes with help of binding.
- v-if: This directives are used for conditional rendering.
- v-on: This directive is used to the DOM elements to listen to the events.
We will understand the Model-View-ViewModel implementation with the help of examples.
Example 1: In the below example v-model directive is used for two-way data binding. v-model is one of the Vue directives used to DOM input elements to be able to modify the data through the Vue instance. This directive synchronizes with the state of the Vue instance (data properties). v-model.lazy is a data binding modifier that only syncs after change events. For example when you enter a text in the input field and then click ENTER or lose focus of the input field it will bind the data. Creating a new Vue instance for two-way binding data using v-model and v-model.lazy directives.
HTML
< template >
< div >
< h1 v-bind:style = "{ color: 'green' }" >
{{name}}
</ h1 >
< input v-model = "userText"
type = "text"
placeholder = 'Type Text Here' />
< h3 >
This is two way binding text:
< span >{{userText}}</ span >
</ h3 >
< input v-model.lazy = "lazyText"
type = "text"
placeholder = 'Type Text Here' />
< h3 >
This is lazy text updates when input field loses focus:
< span >{{lazyText}}</ span >
</ h3 >
</ div >
</ template >
< script >
export default {
name: "App",
data() {
return {
name: 'GeeksforGeeks',
userText: '',
lazyText: ''
};
},
};
</ script >
< style >
span {
color: red
}
</ style >
|
Output:
Example 2: In the below example, we are creating a Vue instance for radio buttons binding with the v-model directive. whenever click any radio button it will render the value for the selected input. v-model directive helps Vue instances to interact between Model and View layers for changing data properties in the DOM.
HTML
< template >
< div >
< h1 >Rate this content</ h1 >
< label >
< input type = "radio"
value = "⭐ Good"
v-model = "rating" />
good
</ label >
< label >
< input type = "radio"
value = "⭐⭐ Very good"
v-model = "rating" />
very good
</ label >
< label >
< input type = "radio"
value = "⭐⭐⭐ Excellent"
v-model = "rating" />
excellent
</ label >
< h1 v-if = rating >
Rating : {{rating}}
</ h1 >
</ div >
</ template >
< script >
export default {
name: "App",
data() {
return {
rating: ''
};
},
};
</ script >
< style >
h1 {
color: green
}
</ style >
|
Output:
Share your thoughts in the comments
Please Login to comment...