Vue.js Reusing Components
Last Updated :
20 Dec, 2022
Vue.js is a progressive javascript framework for developing web user interfaces. It is a performant, approachable, and versatile framework. We can create Single Page Applications as well as Full Stack applications. It is built on top of HTML, CSS, and Javascript which makes it easier for developers to integrate Vue.js in any application at any stage.
Components are used to build the combination of UI elements that need to be called any number of times. In simple terms, you call a function many times when you need to perform the same action on some different sets of input. This is a similar approach to calling the same UI element on different web pages. The UI element called is the Component and the process is Reusing of Components, Some data might be component-specific. Those data need to be bound with a component on a repeated basis. This is when passing data through props to a component comes in handy.
Syntax:
<Component prop=data></Component>
Approach: Here, we will create a Vue project, and then we will create a “To do list” web app. Reuse different task components.
Creating Vue Project:
Step 1: To create a Vue app you need to install Vue modules using this npm command. You need to make sure you have the node installed previously.
npm install vue
Step 2: Use Vue JS through CLI. Open your terminal or command prompt and run the below command.
npm install --global vue-cli
Step 3: Run the below command to create the project.
vue init webpack myproject
Step 4: After creating your Vue project move into the folder to perform different operations.
cd myproject
Step to run the application: Open the terminal and type the following command.
npm run dev
Open your browser. Open a tab with localhost running (http://localhost:8080/) and see the output shown in the image.
Project Structure: After running the commands (mentioned in the above steps), if you open the project in an editor, you can see a similar project structure (as shown below).
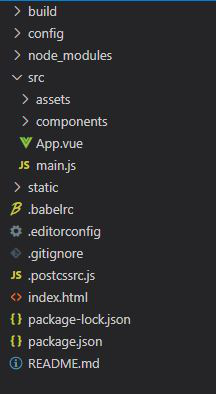
Project Structure
Example 1: In this example, we are creating a UI Card component by creating a card component that will inform the user and that can be reused by the user if he needs to add more cards.
Card.vue
<template>
<div style=
"margin-right:10px;
color:green;
width:400px;
height:200px;
border-style: solid;" >
<h1>{{heading}}</h1>
<h3>{{message}}</h3>
<a :href= "url" >Visit Page</a>
</div>
</template>
<script>
export default {
name: 'Card' ,
props : [ 'message' , 'heading' , 'url' ]
}
</script>
|
App.vue: This file is calling the task component 3 times to display the whole UI.
App.vue
<template>
<center id= "app" >
<h1 style= "color: green" >
GeeksforGeeks
</h1>
<h2>UI Cards</h2>
<div style= "display: inline-block" >
<Card
message=
"Knowledge is power! Come with us
and contribute your knowledge about
Computer Science to our world of Geeks!"
heading= "Write"
</div>
<div style= "display: inline-block" >
<Card
message=
"Master the art of coding by
testing and compiling your codes
at our IDE platform!"
heading= "IDE"
</div>
<div style= "display: inline-block" >
<Card
message=
"There is no limit to the knowledge
that one can have.Learn and code
with industry experts who have designed a
number of problems and courses just for you!"
heading= "Practice"
</div>
</center>
</template>
<script>
import Card from "./components/Card" ;
export default {
name: "App" ,
components: { Card },
};
</script>
|
Output:
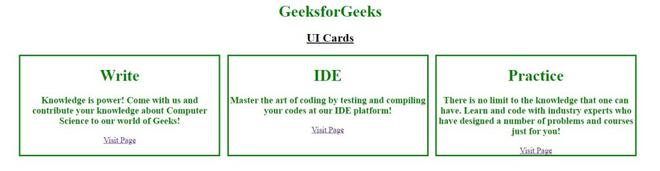
UI Card Components
Example 2: In this example, we are creating a to-do list by using the Reusing Components in VueJS.
Task.vue
<template>
<h3 style=
"color:white;
backgroundColor:green;
width:300px;
height:30px;
padding:15px;" >
{{index}}: {{task}}
</h3>
</template>
<script>
export default {
name: 'Task' ,
props : [ 'task' , 'index' ]
}
</script>
|
App.vue: This file is calling the task component 5 times to display the user’s to-do list.
App.vue
<template>
<center id= "app" >
<h1 style= "color: green" >GeeksforGeeks</h1>
<h2>Vue.js Reusing Components</h2>
<Task task= "Learn DSA" index= "1" />
<Task task= "Daily Practice Problem" index= "2" />
<Task task= "Basic Problems" index= "3" />
<Task task= "Medium-Level Porblem" index= "4" />
<Task task= "Difficult Problem" index= "5" />
</center>
</template>
<script>
import Task from './components/Task' ;
export default {
name: 'App' ,
components: { Task },
};
</script>
|
Output:
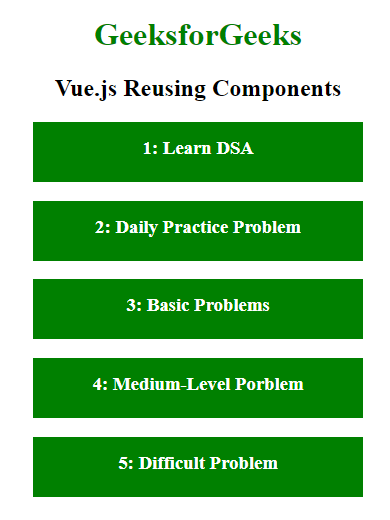
Reusing Component
Reference: https://v2.vuejs.org/v2/guide/components.html#Reusing-Components
Share your thoughts in the comments
Please Login to comment...