Getting Started with View Engine
Last Updated :
18 Apr, 2024
A view engine is a tool used in web development to create dynamic HTML content based on data from the server. It acts as a template processor, allowing developers to integrate data with predefined HTML templates easily. View engines are commonly used in frameworks like Express.js for Node.js, Django for Python, and Ruby on Rails. There are different view engines available for various programming languages, such as EJS, Pug, Handlebars, Django, Freemarker, and more.
Choosing the Right View Engine
When selecting a view engine for your Node.js project, consider factors such as:
- Syntax: Choose a view engine with syntax that aligns with your preferences and team’s expertise (e.g., Pug, EJS, Handlebars).
- Features: Evaluate the features provided by the view engine, such as template inheritance, partials, conditionals, loops, and custom helpers.
- Performance: Consider the performance implications of the view engine, especially for large-scale applications with high traffic.
- Community Support: Check the community support, documentation, and available resources for the chosen view engine to aid in development and troubleshooting.
Features of View Engine
- It provide a template syntax that allows you to embed dynamic content within HTML markup.
- Many view engines support template inheritance that allow you to define a base template with common layout and structure.
- It supports conditional statements like if-else etc.
- It provides mechanisms for iterating over collections or arrays of data.
- It provides data binding capabilities that allow you to bind data from the server-side to the client-side.
- It provides error handling by providing default values for missing data or rendering custom error pages.
These are the types of View Engine:
EJS View Engine
EJS stands for Embedded JavaScript. It is a template engine designed to be used with server-side JavaScript environments like NodeJS and It is used to generate dynamic content in a web page. It simplifies the generation of HTML by allowing you to embed JavaScript code directly in HTML.
Syntax:
<html>
<head>
<title>Welcome Page</title>
</head>
<body>
<h1>Welcome, <%= username %> </h1>
<p>Your email is: <%= email%> </p>
</body>
</html>
Pug View Engine
Pug is a concise and expressive templating language with a syntax that significantly reduces the amount of HTML code needed. It supports template inheritance and provides a clean indentation-based syntax.
Syntax:
// base.pug
html
head
title My Website
body
block content
Handlebars View Engine
Handlebars is a logic-less templating engine that focuses on simplicity and ease of use. It provides features like helpers and partials, making it powerful for building dynamic templates.
Syntax:
{{#if loggedIn}}
<p>Welcome, {{username}}!</p>
{{else}}
<a href="/login">Login</a>
{{/if}}
Mustache View Engine
Mustache is a minimalistic templating engine that emphasizes readability and simplicity. It follows the “logic-less” approach, making it suitable for generating HTML from JSON data.
Syntax:
<ul>
{{#users}}
<li>{{name}}</li>
{{/users}}
</ul>
Steps to Implement EJS in Express
Step 1: Create a NodeJS application using the following command:
npm init
Step 2: Install required Dependencies:
npm i ejs express
Project Structure:
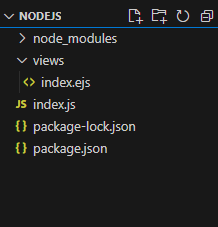
The updated dependencies in package.json file will look like:
"dependencies": {
"ejs": "^3.1.9",
"express": "^4.18.2"
}
Example: The below example is demonstrating the EJS View Engine for Express.
HTML
<!-- views/index.ejs -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>EJS Example</title>
<style>
h1 {
color: green;
}
</style>
</head>
<body>
<h1>GeeksForGeeks | EJS View Engine</h1>
<h2>Welcome, <%= name %>
</h1>
<p>Your email is: <%= email%>
</p>
</body>
</html>
JavaScript
// index.js
const express = require('express');
const path = require('path');
const app = express();
const port = 3000;
// Set EJS as the view engine
app.set('view engine', 'ejs');
app.set('views', path.join(__dirname, 'views'));
// Define a route to render the Pug
// template when the root path is accessed
app.get('/', (req, res) => {
//Sending this data from Server
const data = {
name: "jaimin",
email: "jaimin@gmail.com"
};
// Render the EJS template named 'index' and pass the data
res.render('index', data);
});
// Start the server and listen on the specified port
app.listen(port, () => {
// Display a message when the server starts successfully
console.log(`Server is running at http://localhost:${port}`);
});
Step 4: To run the application use the following command
node index.js
Output: Now go to http://localhost:3000 in your browser:
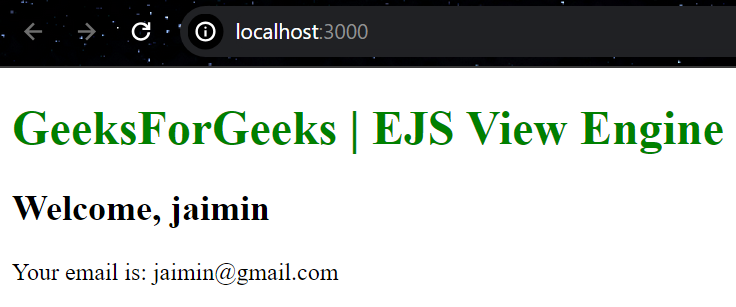
Share your thoughts in the comments
Please Login to comment...