Todo list web application using MERN stack is a project that basically implements basic CRUD operation using MERN stack (MongoDB, Express JS, Node JS, React JS). The users can read, add, update, and delete their to-do list in the table using the web interface. The application gives a feature to the user to add a deadline to their task so that it user can be reminded of the last day to complete the project
Preview of final output: Let us have a look at how the final application will look like.
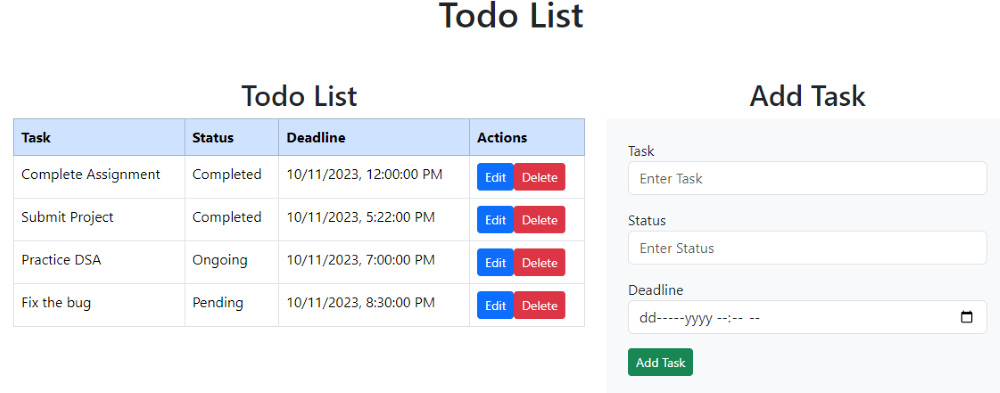
Technologies used / Pre-requisites:
Requirements:
Approach to create Todo List:
- In frontend, the react component called “Todo” is used to display tasks, deadlines and its status and form to add new todo item with edit and deletion feature.
- In backend, application fetches data from MongoDB using backend API called “/getTodoList”. New data can be added to the database using “/addTodoList” API. “/updateTodoList/:id” API is used to edit the existing specific data from the table. “/deleteTodoList/:id” API is used to delete the specific data from the table.
Functionalities Of Todo Application:
- Add new todo: User can add new todo task using the form. On clicking “submit” button, The new data will be saved to database.
- Display todo list: User can view the todo list in table format.
- Edit existing data: User can click on “Edit” button to make the table row to editable fields. On clicking “Edit” button, new buttons call “Save” and “Reset” will be created. “Save” button is to update the edited data to database. “Reset” button is to reset the data in the field.
- Delete existing data: User can click on “Delete” button available in the table row to delete the data from database.
Project Structure:
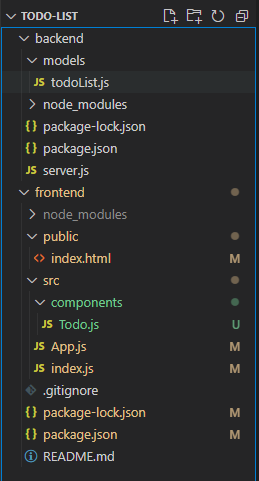
Todo list project structure
Steps to create the project:
Step 1: Create directory for project.
mkdir Todo-List
Step 2: Create sub directories for frontend and backend.
Open Todo-List directory using following command.
cd Todo-List
Create separate directories for frontend and backend.
mkdir frontend
mkdir backend
Use “ls” command to list created folders.
ls
Step 3: Open backend directory using the following command.
cd backend
Step 4: Initialize Node Package Manager using the following command.
npm init
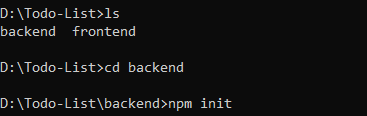
Initialize npm in backend
Step 5: Install express, mongoose and cors package in backend using the following command.
npm install express mongoose cors
Step 6: Come back to Todo-List folder (project main folder).
cd ../
Step 7: Open frontend directory using the following command.
cd frontend
Step 8: Create react application in the current directory using the following command.
npx create-react-app .
Step 9: Install bootstrap, axios and react-router-dom package in backend using the following command.
npm install bootstrap axios react-router-dom
Step 10: Open Todo-List using your familiar code editor.
Come back to Todo-List folder (project main folder).
cd ../
If you are using VS code editor, run the following command open VS code in current folder.
code .
Step 11: Navigate to frontend directory in code editor.
Step 12: Delete files inside ‘src’ folder and ‘public’ folder (Don’t delete folder).
Step 13: Add files in frontend directory as per the project structure with below code.
Step 14: Navigate to backend directory in code editor.
Step 15: Create server.js and model file as per project structure.
Step 16: Add files in backend directory as per the project structure with below code.
Step 17: Replace database connection URL with your own connection URL in server.js file.
Final Project Structure should be like this,
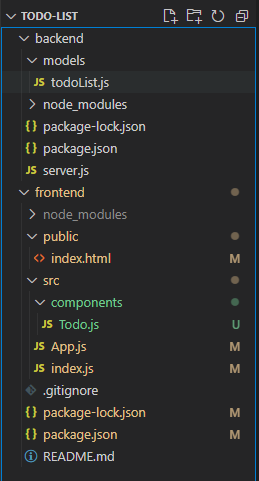
Project Structure
The updated dependencies in package.json for frontend will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"axios": "^1.5.0",
"bootstrap": "^5.3.2",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.16.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
The updated dependencies in package.json for backend will look like:
"dependencies": {
"cors": "^2.8.5",
"express": "^4.18.2",
"mongoose": "^7.5.2"
}
Example : Write the following code in respective files
Frontend code:
- App.js: This is our main file which routes to the Todo component.
- Todo.js: This component contains all the logic for displaying Tasks, Status of tasks and deadlines of the tasks fetched from database in table format, adding new todo list to database, deleting existing todo from database and editing existing todo in database.
Javascript
import React from 'react' ;
import { BrowserRouter, Routes, Route } from 'react-router-dom' ;
import 'bootstrap/dist/css/bootstrap.min.css' ;
import Todo from './components/Todo' ;
function App() {
const headStyle = {
textAlign: "center" ,
}
return (
<div>
<h1 style={headStyle}>Todo List</h1>
<BrowserRouter>
<Routes>
<Route path= '/' element={<Todo/>}></Route>
</Routes>
</BrowserRouter>
</div>
);
}
export default App;
|
Javascript
import axios from "axios" ;
import React from "react" ;
import { useEffect, useState } from "react" ;
function Todo() {
const [todoList, setTodoList] = useState([]);
const [editableId, setEditableId] = useState( null );
const [editedTask, setEditedTask] = useState( "" );
const [editedStatus, setEditedStatus] = useState( "" );
const [newTask, setNewTask] = useState( "" );
const [newStatus, setNewStatus] = useState( "" );
const [newDeadline, setNewDeadline] = useState( "" );
const [editedDeadline, setEditedDeadline] = useState( "" );
useEffect(() => {
.then(result => {
setTodoList(result.data)
})
. catch (err => console.log(err))
}, [])
const toggleEditable = (id) => {
const rowData = todoList.find((data) => data._id === id);
if (rowData) {
setEditableId(id);
setEditedTask(rowData.task);
setEditedStatus(rowData.status);
setEditedDeadline(rowData.deadline || "" );
} else {
setEditableId( null );
setEditedTask( "" );
setEditedStatus( "" );
setEditedDeadline( "" );
}
};
const addTask = (e) => {
e.preventDefault();
if (!newTask || !newStatus || !newDeadline) {
alert( "All fields must be filled out." );
return ;
}
.then(res => {
console.log(res);
window.location.reload();
})
. catch (err => console.log(err));
}
const saveEditedTask = (id) => {
const editedData = {
task: editedTask,
status: editedStatus,
deadline: editedDeadline,
};
if (!editedTask || !editedStatus || !editedDeadline) {
alert( "All fields must be filled out." );
return ;
}
.then(result => {
console.log(result);
setEditableId( null );
setEditedTask( "" );
setEditedStatus( "" );
setEditedDeadline( "" );
window.location.reload();
})
. catch (err => console.log(err));
}
const deleteTask = (id) => {
.then(result => {
console.log(result);
window.location.reload();
})
. catch (err =>
console.log(err)
)
}
return (
<div className= "container mt-5" >
<div className= "row" >
<div className= "col-md-7" >
<h2 className= "text-center" >Todo List</h2>
<div className= "table-responsive" >
<table className= "table table-bordered" >
<thead className= "table-primary" >
<tr>
<th>Task</th>
<th>Status</th>
<th>Deadline</th>
<th>Actions</th>
</tr>
</thead>
{Array.isArray(todoList) ? (
<tbody>
{todoList.map((data) => (
<tr key={data._id}>
<td>
{editableId === data._id ? (
<input
type= "text"
className= "form-control"
value={editedTask}
onChange={(e) => setEditedTask(e.target.value)}
/>
) : (
data.task
)}
</td>
<td>
{editableId === data._id ? (
<input
type= "text"
className= "form-control"
value={editedStatus}
onChange={(e) => setEditedStatus(e.target.value)}
/>
) : (
data.status
)}
</td>
<td>
{editableId === data._id ? (
<input
type= "datetime-local"
className= "form-control"
value={editedDeadline}
onChange={(e) => setEditedDeadline(e.target.value)}
/>
) : (
data.deadline ? new Date(data.deadline).toLocaleString() : ''
)}
</td>
<td>
{editableId === data._id ? (
<button className= "btn btn-success btn-sm" onClick={() => saveEditedTask(data._id)}>
Save
</button>
) : (
<button className= "btn btn-primary btn-sm" onClick={() => toggleEditable(data._id)}>
Edit
</button>
)}
<button className= "btn btn-danger btn-sm ml-1" onClick={() => deleteTask(data._id)}>
Delete
</button>
</td>
</tr>
))}
</tbody>
) : (
<tbody>
<tr>
<td colSpan= "4" >Loading products...</td>
</tr>
</tbody>
)}
</table>
</div>
</div>
<div className= "col-md-5" >
<h2 className= "text-center" >Add Task</h2>
<form className= "bg-light p-4" >
<div className= "mb-3" >
<label>Task</label>
<input
className= "form-control"
type= "text"
placeholder= "Enter Task"
onChange={(e) => setNewTask(e.target.value)}
/>
</div>
<div className= "mb-3" >
<label>Status</label>
<input
className= "form-control"
type= "text"
placeholder= "Enter Status"
onChange={(e) => setNewStatus(e.target.value)}
/>
</div>
<div className= "mb-3" >
<label>Deadline</label>
<input
className= "form-control"
type= "datetime-local"
onChange={(e) => setNewDeadline(e.target.value)}
/>
</div>
<button onClick={addTask} className= "btn btn-success btn-sm" >
Add Task
</button>
</form>
</div>
</div>
</div>
)
}
export default Todo;
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >Todo List</ title >
</ head >
< body >
< noscript >You need to enable JavaScript to run this app.</ noscript >
< div id = "root" ></ div >
</ body >
</ html >
|
Backend:
- Server.js: This is a file which contains logic to start the backend server, APIs to interact with database.
- todoList.js: This is a model file called a wrapper of the Mongoose schema. This file is used to define the schema of table that stores the todo list.
Javascript
const express = require( 'express' )
const mongoose = require( 'mongoose' )
const cors = require( 'cors' )
const TodoModel = require( "./models/todoList" )
var app = express();
app.use(cors());
app.use(express.json());
mongoose.connection.on( "error" , (error) => {
console.error( "MongoDB connection error:" , error);
});
app.get( "/getTodoList" , (req, res) => {
TodoModel.find({})
.then((todoList) => res.json(todoList))
. catch ((err) => res.json(err))
});
app.post( "/addTodoList" , (req, res) => {
TodoModel.create({
task: req.body.task,
status: req.body.status,
deadline: req.body.deadline,
})
.then((todo) => res.json(todo))
. catch ((err) => res.json(err));
});
app.post( "/updateTodoList/:id" , (req, res) => {
const id = req.params.id;
const updateData = {
task: req.body.task,
status: req.body.status,
deadline: req.body.deadline,
};
TodoModel.findByIdAndUpdate(id, updateData)
.then((todo) => res.json(todo))
. catch ((err) => res.json(err));
});
app. delete ( "/deleteTodoList/:id" , (req, res) => {
const id = req.params.id;
TodoModel.findByIdAndDelete({ _id: id })
.then((todo) => res.json(todo))
. catch ((err) => res.json(err));
});
app.listen(3001, () => {
console.log( 'Server running on 3001' );
});
|
Javascript
const mongoose = require( 'mongoose' );
const todoSchema = new mongoose.Schema({
task: {
type: String,
required: true ,
},
status: {
type: String,
required: true ,
},
deadline: {
type: Date,
},
});
const todoList = mongoose.model( "todo" , todoSchema);
module.exports = todoList;
|
Steps to run application:
Step 1: Open Todo-List/backend folder in new terminal / command prompt.
Step 2: Execute the command to start backend API server.
npm start
Now, our backend server is running in localhost on port 3001.
http://localhost:3001/
Step 3: Open Todo-List/frontend folder in another new terminal / command prompt.
Step 4: Execute the following command to run react app.
npm start
Open the browser and navigate to the following link to open the application.
http://localhost:3000/
Output:
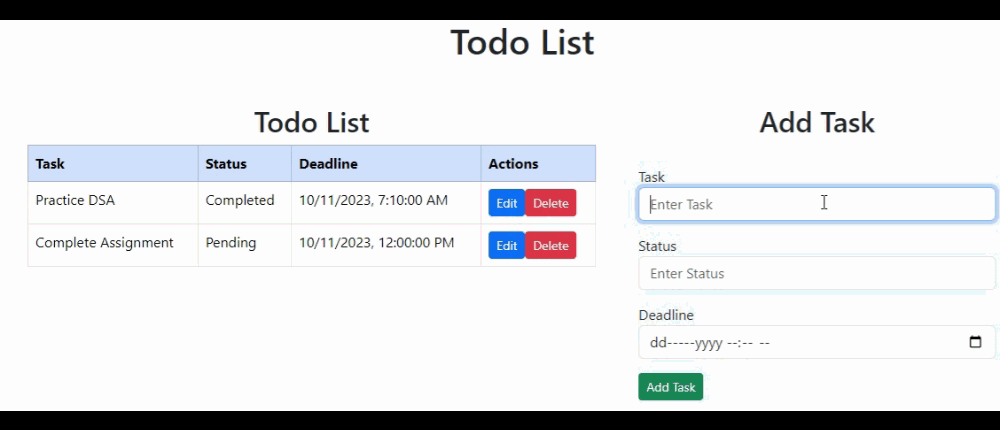
Todo list application using MERN
Share your thoughts in the comments
Please Login to comment...