Explain StaticRouter in React Router
Last Updated :
28 Apr, 2024
React Router is a powerful library for handling routing in React applications, allowing developers to create dynamic single-page applications (SPAs) with ease. While the BrowserRouter is commonly used for client-side routing in web applications, there are scenarios where server-side rendering (SSR) or static site generation (SSG) is preferred. This is where the StaticRouter comes into play. In this article, we’ll explore what the StaticRouter is, how it works, and when you might want to use it in your React applications.
Prerequisites:
What is StaticRouter?
The StaticRouter is a component provided by React Router designed specifically for server-side rendering (SSR) or static site generation (SSG) scenarios. Unlike BrowserRouter, which is optimized for client-side rendering in the browser environment, StaticRouter is intended for use on the server side or in environments where client-side JavaScript execution is limited or non-existent.
How does it work?
Server-side rendering (SSR):
When rendering a React application on the server side, the StaticRouter is used to match the incoming HTTP request URL to the appropriate route and render the corresponding component. This allows the server to generate the initial HTML content dynamically based on the requested URL, which is then sent to the client as part of the server response.
Static site generation (SSG):
In scenarios where you want to pre-render your React application into static HTML files at build time, the StaticRouter can be used in conjunction with tools like webpack and static site generators such as Gatsby or Next.js. During the build process, the StaticRouter is used to generate static HTML files for each route in your application, which can then be served statically without the need for server-side rendering at runtime.
When to use StaticRouter?
- Server-side rendering (SSR): When you need to render React components on the server side to improve performance, enable SEO, or support non-JavaScript clients.
- Static site generation (SSG): When you want to pre-render your React application into static HTML files at build time for improved performance, better SEO, and simpler deployment.
- Limited client-side JavaScript execution: In environments where client-side JavaScript execution is limited or non-existent, such as certain web crawlers, bots, or legacy browsers, StaticRouter can ensure that your application remains functional and accessible.
Key Props of StaticRouter
basename: string
Sets the base URL for all locations, useful for nested route configurations.
<StaticRouter basename="/calendar">
location: string
Specifies the URL location for rendering. In SSR, this often corresponds to the incoming request URL
<StaticRouter location={req.url}>
context: object
A plain JavaScript object used to capture render context during SSR.
<StaticRouter location={req.url} context={context}>
Steps to Create the Application
Step 1:Â Create a reactJS application by using this command
npx create-react-app myapp
Step 2:Â Navigate to project directory
cd myapp
Step 3:Â Install the necessary packages/libraries in your project using the following commands.
npm install react-router-dom
Project Structure:
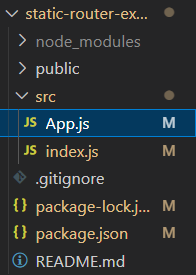
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.22.3",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
},
Example: Implementation to show the use of staticRouter with an example.
JavaScript
// src/App.js
import React from 'react';
import { StaticRouter, Route, Link } from 'react-router-dom';
const Home = () => (
<div>
<h2>Welcome to the Home Page</h2>
<Link to="/about">About</Link>
</div>
);
const About = () => (
<div>
<h2>About Us</h2>
<p>This is the about page.</p>
<Link to="/">Home</Link>
</div>
);
const App = () => (
<StaticRouter location="/home" context={{}}>
<div>
<Route exact path="/home" component={Home} />
<Route path="/about" component={About} />
</div>
</StaticRouter>
);
export default App;
JavaScript
// src/index.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
ReactDOM.render(<App />, document.getElementById('root'));
Step to Run Application:Â Run the application using the following command from the root directory of the project
npm start
Output:Â Your project will be shown in the URL http://localhost:3000/
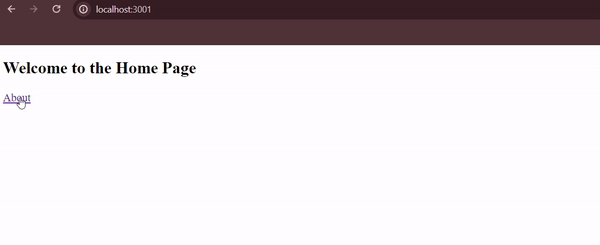
output
Conclusion
The StaticRouter in React Router is a valuable tool for server-side rendering (SSR) and static site generation (SSG) scenarios, allowing you to render React applications on the server side or pre-render them into static HTML files at build time. By understanding how and when to use the StaticRouter, you can create React applications that are optimized for performance, accessibility, and SEO in a variety of environments.
Share your thoughts in the comments
Please Login to comment...