Explain Route matching priority in VueJS ?
Last Updated :
20 Sep, 2023
Route Matching
Route Matching is the concept in which the Vue Router specifies which component is to be rendered according to the URL entered by the end user. If the user navigates to any specific URL, Vue Router checks for the defined routes and finds the match for that URL. It later compares the entered URL with the path patterns specified in the route config file to identify the correct component to render.
Route Matching Priority
In the Vue.JS framework, route matching priority is the concept that refers to the order or sequence in which the routes are evaluated to determine which one should handle a particular URL. If a user navigates to the specific URL in a Vue.js project, the router looks for the matching route in the defined routes list. The router evaluates the routes one by one in the order they are defined, and the first route that matches the URL is used to render the corresponding component.
Syntax:
import { createRouter, createWebHistory }
from 'vue-router';
const routes = [
// Route configuration objects
{ path: '/route1', component: Route1 },
{ path: '/route2', component: Route2 },
{ path: '/route3', component: Route3 },
// ...
];
const router = createRouter({
history: createWebHistory(),
routes,
});
export default router;
In the above syntax, we have defined an array of routes that consists of all the route configuration objects.
Installation of Vue Project:
Step 1: Initially, for creating the Vue project, we need to install the Vue modules using the below command. Execute the command in the terminal to install the modules.
npm install vue
Step 2: We can use Vue JS through CLI. We need to execute the below command in the command prompt or terminal.
npm install --global vue-cli
Step 3: Now, we will execute the below command to create the new project.
vue init webpack <<project_name>>
Step 4: Navigate to the project folder by using the cd command.
cd <<project_name>>
Step 5: Vue Router can be installed through Npm with the package named vue-router using the below command.
npm install vue-router // OR
vue add router
Project Structure:
It will look like this.
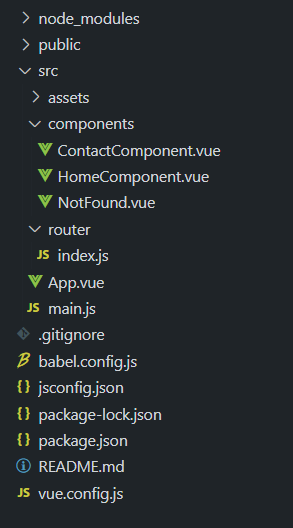
package.json: Below is the package.json for reference:
{
"name": "my-fresh-project",
"version": "0.1.0",
"private": true,
"scripts": {
"serve": "vue-cli-service serve",
"build": "vue-cli-service build",
"lint": "vue-cli-service lint"
},
"dependencies": {
"core-js": "^3.8.3",
"vue": "^3.2.13",
"vue-router": "^4.2.4"
},
"devDependencies": {
"@babel/core": "^7.12.16",
"@babel/eslint-parser": "^7.12.16",
"@vue/cli-plugin-babel": "~5.0.0",
"@vue/cli-plugin-eslint": "~5.0.0",
"@vue/cli-service": "~5.0.0",
"eslint": "^7.32.0",
"eslint-plugin-vue": "^8.0.3"
},
"eslintConfig": {
"root": true,
"env": {
"node": true
},
"extends": [
"plugin:vue/vue3-essential",
"eslint:recommended"
],
"parserOptions": {
"parser": "@babel/eslint-parser"
},
"rules": {}
},
"browserslist": [
"> 1%",
"last 2 versions",
"not dead",
"not ie 11"
]
}
Example: In this example, we will display the implementation of route matching in VueJS.
Javascript
import { createApp } from 'vue' ;
import App from './App.vue' ;
import router from './router' ;
const app = createApp(App);
app.use(router);
app.mount( '#app' );
|
Javascript
import { createRouter, createWebHistory }
from 'vue-router' ;
import Home from '../components/HomeComponent.vue' ;
import Contact from '../components/ContactComponent.vue' ;
import NotFound from '../components/NotFound.vue' ;
const routes = [
{ path: '/' , component: Home },
{ path: '/contact' , component: Contact },
{ path: '/:pathMatch(.*)' , component: NotFound },
];
const router = createRouter({
history: createWebHistory(),
routes,
});
export default router;
|
HTML
< template >
< div class = "home-container" >
< h1 class = "title"
style = "color: green;" >
Welcome to GeeksforGeeks
</ h1 >
< p class = "description" >
GeeksforGeeks is a leading platform that
provides computer science resources and
coding challenges for programmers and
technology enthusiasts, along with interview
and exam preparations for upcoming aspirants.
With a strong emphasis on enhancing coding
skills and knowledge, it has become a
trusted destination for over 12 million
plus registered users worldwide. The platform
offers a vast collection of tutorials, practice
problems, interview tutorials, articles,
and courses, covering various domains of
computer science.
</ p >
< button class = "btn" @ click = "showAlert" >
Show Alert
</ button >
< router-link to = "/contact" class = "link" >
Go to Contact
</ router-link >
</ div >
</ template >
< script >
export default {
methods: {
showAlert() {
alert('You clicked the button!');
},
},
};
</ script >
< style >
.home-container {
padding: 20px;
text-align: center;
max-width: 700px;
margin: 0 auto;
background-color: #f7f7f7;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
}
.title {
font-size: 3rem;
margin-bottom: 20px;
color: #30ee00;
}
.description {
font-size: 1.2rem;
color: #444;
}
.btn {
background-color: #007bff;
color: #fff;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
font-size: 1.2rem;
transition: background-color 0.3s;
margin-top: 20px;
}
.btn:hover {
background-color: #0056b3;
}
.link {
color: #007bff;
text-decoration: none;
transition: color 0.3s;
margin-top: 10px;
}
.link:hover {
color: #0056b3;
}
</ style >
|
HTML
< template >
< div class = "contact-container" >
< h1 class = "title"
style = "color: green;" >
Contact Us
</ h1 >
< p class = "description" >
You can contact us via email at
< a href = "support@geeksforgeeks.org" >
support@geeksforgeeks.org
</ a >
or by phone at
< a href = "tel:+91-7838223507" >
+91-7838223507
</ a >.
</ p >
< form @ submit.prevent = "submitForm"
class = "form" >
< div class = "form-group" >
< label for = "name" >Name:</ label >
< input type = "text"
id = "name"
v-model = "formData.name" required />
</ div >
< div class = "form-group" >
< label for = "email" >Email:</ label >
< input type = "email"
id = "email"
v-model = "formData.email" required />
</ div >
< div class = "form-group" >
< label for = "message" >Message:</ label >
< textarea id = "message"
v-model = "formData.message" required>
</ textarea >
</ div >
< button type = "submit"
class = "btn" >
Submit
</ button >
</ form >
< router-link to = "/"
class = "link" >
Go to Home
</ router-link >
</ div >
</ template >
< script >
export default {
data() {
return {
formData: {
name: '',
email: '',
message: '',
},
};
},
methods: {
submitForm() {
alert('Form submitted successfully!');
this.formData = {
name: '',
email: '',
message: '',
};
},
},
};
</ script >
< style >
.contact-container {
padding: 20px;
text-align: center;
max-width: 600px;
margin: 0 auto;
background-color: #f7f7f7;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
}
.title {
font-size: 3rem;
margin-bottom: 20px;
color: #007bff;
}
.description {
font-size: 1.2rem;
color: #444;
}
.description a {
color: #007bff;
text-decoration: none;
transition: color 0.3s;
}
.description a:hover {
color: #0056b3;
}
.form {
margin-top: 20px;
}
.form-group {
margin-bottom: 20px;
}
form input,
form textarea {
width: 100%;
padding: 10px;
font-size: 1rem;
border: 1px solid #ccc;
border-radius: 5px;
}
form .btn {
background-color: #007bff;
color: #fff;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
font-size: 1.2rem;
transition: background-color 0.3s;
}
form .btn:hover {
background-color: #0056b3;
}
.link {
color: #007bff;
text-decoration: none;
transition: color 0.3s;
margin-top: 10px;
}
.link:hover {
color: #0056b3;
}
</ style >
|
HTML
< template >
< div class = "not-found-container" >
< h1 class = "title" >404 Not Found</ h1 >
< p class = "description" >
Oops! The page you are looking
for does not exist.
</ p >
< router-link to = "/"
class = "link" >Go to Home
</ router-link >
</ div >
</ template >
< script >
export default {
};
</ script >
< style >
.not-found-container {
padding: 20px;
text-align: center;
max-width: 600px;
margin: 0 auto;
background-color: #f7f7f7;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
}
.title {
font-size: 3rem;
margin-bottom: 20px;
color: #007bff;
}
.description {
font-size: 1.2rem;
color: #444;
}
.link {
color: #007bff;
text-decoration: none;
transition: color 0.3s;
margin-top: 10px;
}
.link:hover {
color: #0056b3;
}
</ style >
|
HTML
< template >
< div id = "app" >
< router-view ></ router-view >
</ div >
</ template >
< script >
export default {};
</ script >
< style >
#app {
font-family: Arial, sans-serif;
}
</ style >
|
Explanation: The “routes” array in the above code snippet refers to the sequence in which the routes are matched by the Vue Router. When a user accesses a URL, the Vue Router will navigate through each route definition in the order listed in the “routes” array before looking for a match with the URL the user entered.
The route with the most specific path match will take priority, and the catch-all route will act as the fallback for any of the unmatched paths. The <router-view> can be used to display the routing components based on the routes.
Step 6: Step to run the application
Run the Vue application using the following command in the VS Code IDE.
npm run serve
Output:
To view the application, paste the URL “http://localhost:8080” into the web browser.
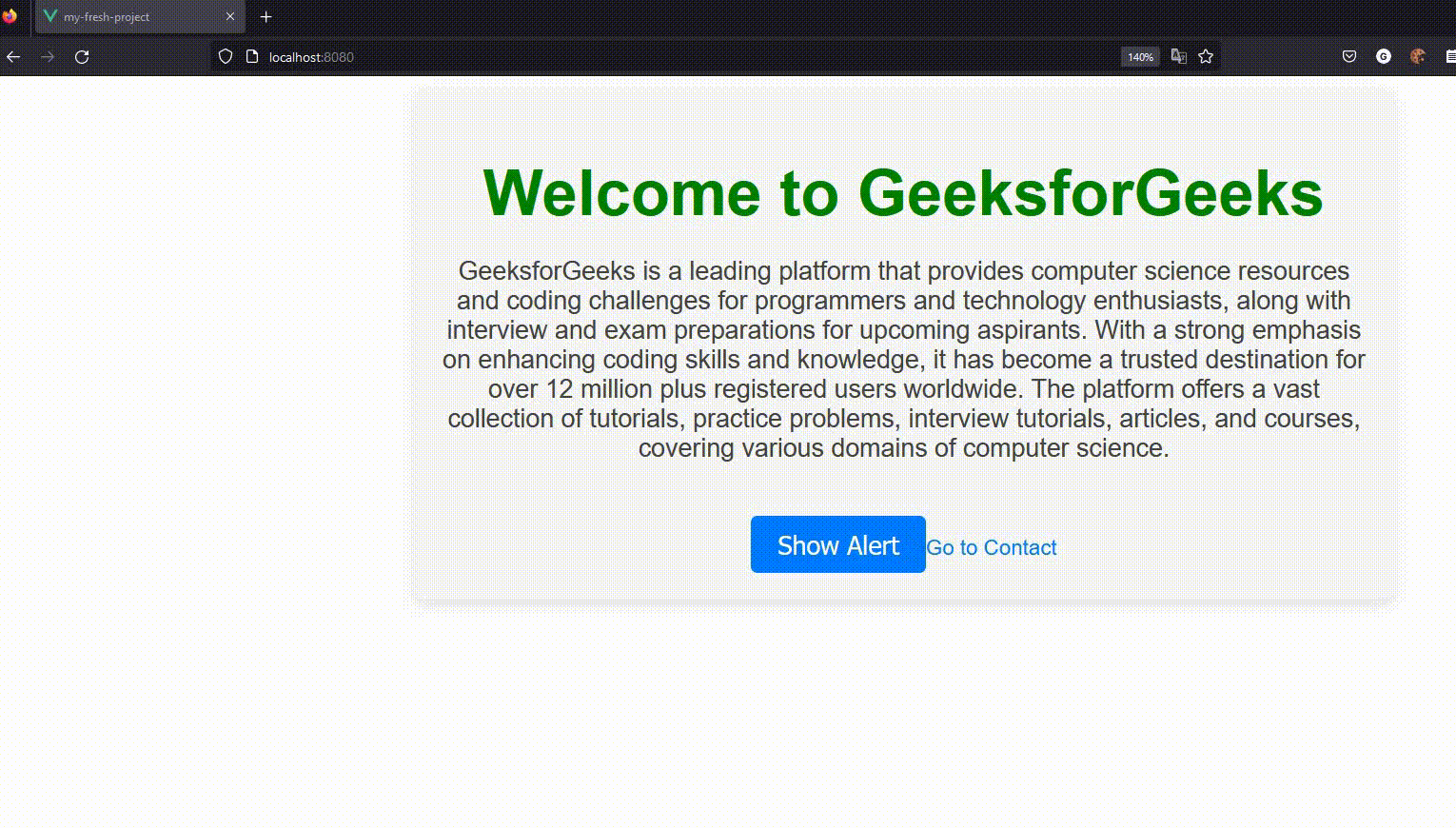
Conclusion:
Understanding the concept of route matching priority in Vue.js is important for developing reliable and dynamic web applications. Route Matching specifies which component should be rendered by matching the pattern from the route configuration. By setting up the routes in the correct order, web developers can actually handle the low of the application response to different URL paths.
Share your thoughts in the comments
Please Login to comment...