Disabling the Button when input field is empty in Angular
Last Updated :
07 Dec, 2023
A Disabled button is un-clickable and unusable. This is useful when suppose user has not entered a password then the submit button should be disabled. In this article, we will see the Disable button when input is empty in Angular.Â
Steps for Installing & Configuring the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Project Structure
It will look like the following:
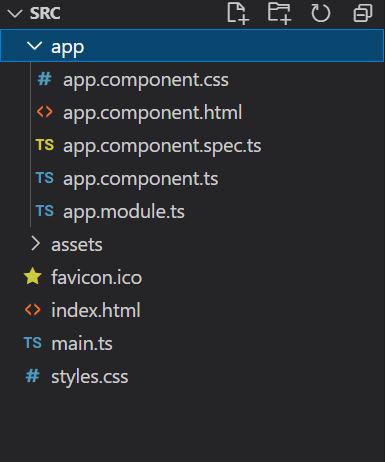
Example 1: In this example, we will use a function that will check the length of the password. If it is equal to zero then make the button disabled otherwise, enable.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >
Disable button when input is empty in Angular </ h2 >
< p >
Will you vote for me for Geeks Premier League 2023 ?
</ p >
< input required [(ngModel)]="password"
(ngModelChange)="checkPasswordEmpty()">
< button [disabled]="invalid">Submit</ button >
|
Javascript
import { DatePipe } from '@angular/common' ;
import { Component } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
})
export class AppComponent {
password: string = ""
invalid: boolean = true
console = console
checkPasswordEmpty() {
console.log( "hi" )
if ( this .password.length == 0) {
this .invalid = true
}
else {
this .invalid = false
}
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { FormsModule }
from '@angular/forms'
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent,
],
imports: [
BrowserModule,
HttpClientModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output
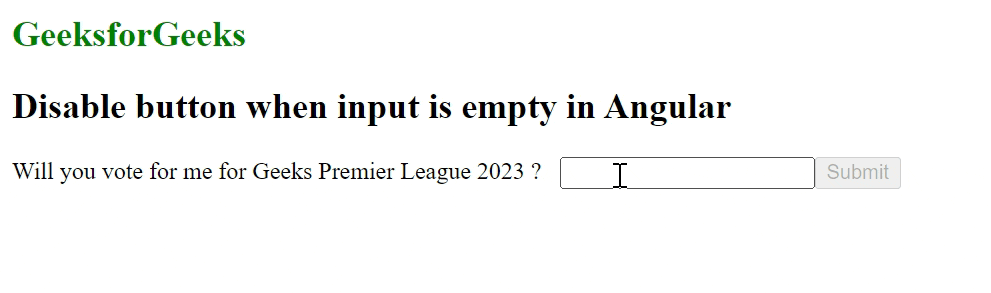
Example 2: In this example, we will use form validators using the ngForm Directive.
HTML
app.component.html
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >
Disable button when input
is empty in Angular
</ h2 >
< p >
Will you vote for me for
Geeks Premier League 2023 ?
</ p >
< form # formCtrl = "ngForm" >
< input required [(ngModel)]="password"
name = "password" >
< button [disabled]="!formCtrl.form.valid">
Submit
</ button >
{{console.log(formCtrl.form.valid)}}
</ form >
|
Javascript
import { DatePipe } from '@angular/common' ;
import { Component } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
})
export class AppComponent {
console = console
password: string = ""
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { FormsModule }
from '@angular/forms'
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent,
],
imports: [
BrowserModule,
HttpClientModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
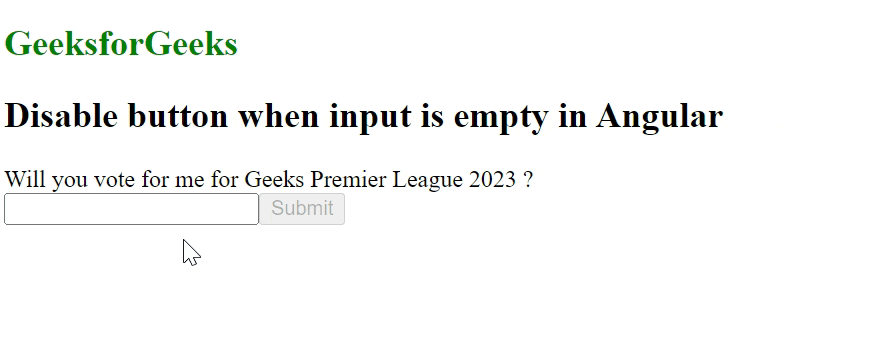
Share your thoughts in the comments
Please Login to comment...