How to Add an Input Field on Button Click in JavaScript ?
Last Updated :
21 Mar, 2024
JavaScript allows us to dynamically add an input field upon button click. We can use the below approaches for adding an input field on Button Click in JavaScript.
Using DOM Manipulation
DOM manipulation is used to dynamically create and append input fields upon clicking the “Add Input Field” button. We create a div wrapper for each input field to ensure the proper layout and styling.
Example: The below example uses DOM Manipulation to Add an input Field on a Button Click in JavaScript.
HTML
<!DOCTYPE html>
<head>
<title>Example 1</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
}
.container {
display: block;
text-align: center;
margin-top: 20px;
}
.input-field {
width: 200px;
padding: 8px;
border: 1px solid #ccc;
border-radius: 5px;
margin: 10px auto;
}
.add-button {
padding: 8px 20px;
background-color: green;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
}
</style>
</head>
<body>
<h3>Using DOM Manipulation</h3>
<div class="container">
<button class="add-button" onclick="addFn()">Add Input Field</button>
<div id="inputFields"></div>
</div>
<script>
function addFn() {
const divEle = document.getElementById("inputFields");
const wrapper = document.createElement("div");
const iFeild = document.createElement("input");
iFeild.setAttribute("type", "text");
iFeild.setAttribute("placeholder", "Enter value");
iFeild.classList.add("input-field");
wrapper.appendChild(iFeild);
divEle.appendChild(wrapper);
}
</script>
</body>
</html>
Output:
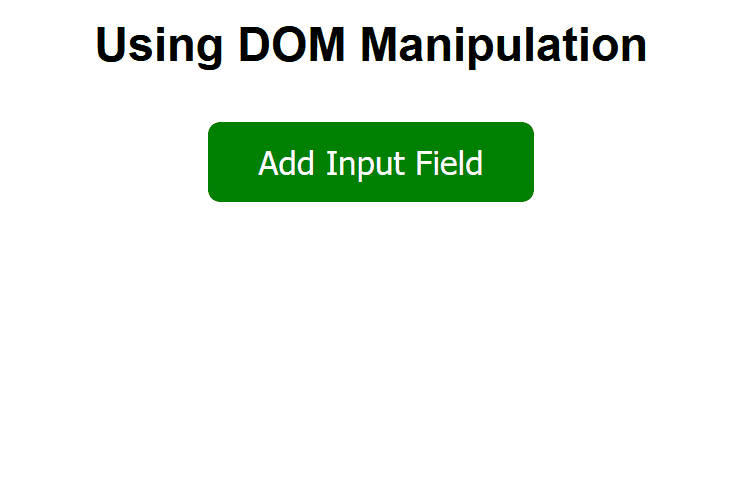
Using Template Literal and InnerHTML
In this approach, we are using template literals and innerHTML to dynamically generate HTML content upon clicking the “Add Input Fields” button. Two input fields are added inside a div wrapper each time the button is clicked.
Example: The below example uses Template Literal and InnerHTML to Add an input Field on a Button Click in JavaScript.
HTML
<!DOCTYPE html>
<head>
<title>Example 2</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
}
.container {
display: block;
text-align: center;
margin-top: 20px;
}
.input-field {
width: 200px;
padding: 8px;
border: 1px solid #ccc;
border-radius: 5px;
margin: 10px auto;
}
.add-button {
padding: 8px 20px;
background-color: green;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
}
</style>
</head>
<body>
<h3>Using Template Literal and InnerHTML</h3>
<div class="container">
<button class="add-button" onclick="addFn()">Add Input Fields</button>
<div id="inputFields">
</div>
</div>
<script>
function addFn() {
const divEle = document.getElementById("inputFields");
divEle.innerHTML += `
<div>
<input type="text" placeholder="Enter value" class="input-field">
<input type="text" placeholder="Enter value" class="input-field">
</div>
`;
}
</script>
</body>
</html>
Output:
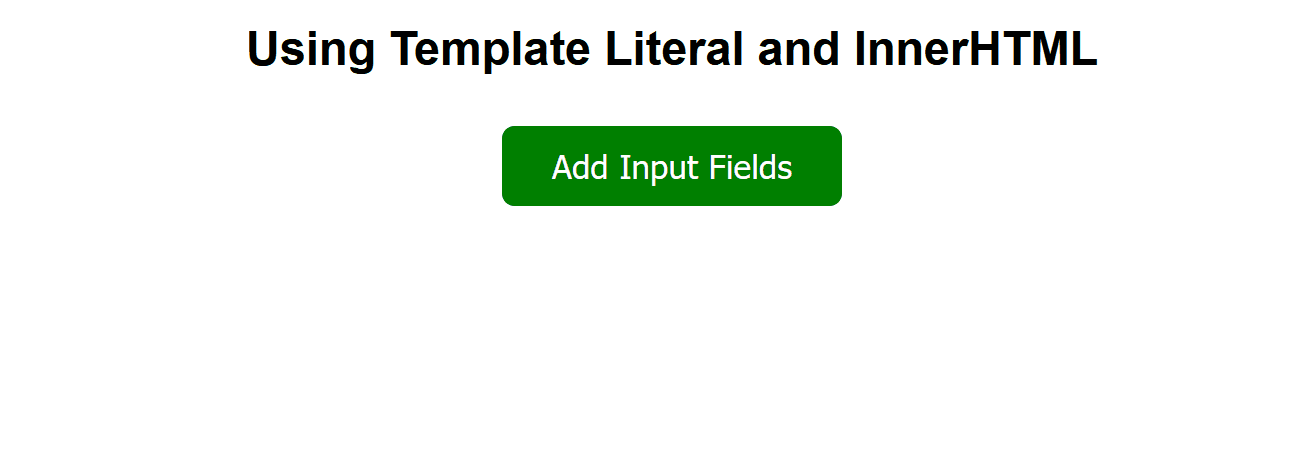
Share your thoughts in the comments
Please Login to comment...