Difference between index.ejs and index.html
Last Updated :
09 Apr, 2021
What is HTML?
HTML (Hypertext Markup Language) is used to design the structure of a web page and its content. HTML is actually not technically programming languages like C++, JAVA, or python. It is a markup language, and it ultimately gives declarative instructions to the browser. When a web page is loaded, the browser first reads the HTML and constructs DOM (Document Object Model) tree from it. HTML consists of a series of elements.
What is a Template Engine?
A template engine is a tool that enables developers to write HTML markup using plain JavaScript. The template engine has its own defined syntax or tags that will either insert variables or run some programming logic at run time and generate final HTML before sending it to the browser for display.
What is EJS?
EJS simply stands for Embedded JavaScript. It is a simple template language or engine. EJS has its own defined syntax and tags. It offers an easier way to generate HTML dynamically.
Relation between HTML and EJS:
We actually define HTML in the EJS syntax and specify how and where various data will go on the page. Then the template engine combines these data and runs programming logic to generate the final HTML page. So, the task of EJS is to take your data and inserts it into the web page according to how you’ve defined the template and generate the final HTML.
For example, you could have a table of dynamic data from a database, and you want EJS to generate the table of data according to your display rules.
When to use HTML/ EJS?
It varies according to your application. If you want to render a static page then go for an HTML file and if you want to render a dynamic page where your data coming from various sources then you must choose an EJS file.
Difference between index.ejs and index.html:
HTML file |
EJS file
|
Good for the static web page. |
Good for the dynamic web page. |
HTML makup language is used to write HTML file. |
JavaScript is used to write EJS files. |
Written in HTML language. |
HTML markup generates dynamically using JavaScript. |
It could not bind dynamic data before sending it to the browser. |
Dynamic data will bind with the template then the final output will send to the browser. |
Extension of HTML file is .html |
Extension of EJS file is .ejs |
Example: Assume you have a web page to show employee’s salaries. If you create a static HTML file then you need to rewrite it every time when new employee added or salary changes. But, with EJS it becomes too easy to insert dynamic data into it.
HTML Version Code:
index.html
<!DOCTYPE html>
< html >
< head >
< title >Employee Salary</ title >
< style >
table {
margin: 20% auto;
border-collapse: collapse;
border-spacing: 0;
width: 20%;
border: 1px solid #ddd;
}
th,
td {
text-align: left;
padding: 16px;
}
tr:nth-child(even) {
background-color: #d1d1d1;
}
</ style >
</ head >
< body >
< table >
< tr >
< th > Employee </ th >
< th > Salary </ th >
</ tr >
< tr >
< td > Sayan Ghosh </ td >
< td > 37000 </ td >
</ tr >
< tr >
< td > Susmita Sahoo </ td >
< td > 365000 </ td >
</ tr >
< tr >
< td > Nabonita Santra </ td >
< td > 36000 </ td >
</ tr >
< tr >
< td > Anchit Ghosh </ td >
< td > 30000 </ td >
</ tr >
</ table >
</ body >
</ html >
|
Output:
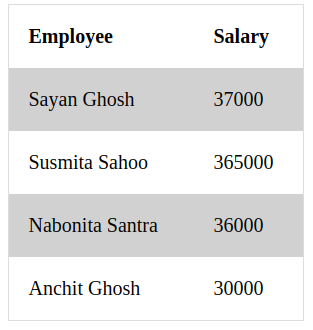
EJS Version Code:
Step 1: First, create a NodeJS application and install the required modules like express.js and ejs modules.
Note: Refer to https://www.geeksforgeeks.org/how-to-use-ejs-in-javascript/ to get started with EJS.
Step 2: Create an index.js file which is our basic server with the following code.
index.js
var express = require( 'express' );
var app = express();
app.set( 'view engine' , 'ejs' );
const empSalary = [
{
name: "Sayan Ghosh" ,
salary: 37000
},
{
name: "Susmita Sahoo" ,
salary: 365000
},
{
name: "Nabonita Santra" ,
salary: 36000
},
{
name: "Anchit Ghosh" ,
salary: 30000
}
]
app.get( '/employee/salary' , (req, res) => {
res.render( 'index.ejs' , { empSalary: empSalary });
})
app.listen(3000, function (req, res) {
console.log( "Connected on port:3000" );
});
|
Step 3: Create an index.ejs file and put it in the views folder with the following code.
index.ejs
<!DOCTYPE html>
< html >
< head >
< title >Employee Salary</ title >
< style >
table {
margin: 20% auto;
border-collapse: collapse;
border-spacing: 0;
width: 20%;
border: 1px solid #ddd;
}
th, td {
text-align: left;
padding: 16px;
}
tr:nth-child(even) {
background-color: #d1d1d1;
}
</ style >
</ head >
< body >
< table >
< tr >
< th > Employee </ th >
< th > Salary </ th >
</ tr >
<% empSalary.forEach(emp => { %>
< tr >
< td > <%= emp.name %>
< td > <%= emp.salary %>
</ tr >
<% }); %>
</ table >
</ body >
</ html >
|
Step 4: Run the server using the following command:
node index.js
Output: Now open your browser and go to http://localhost:3000/employee/salary, you will see the following output:
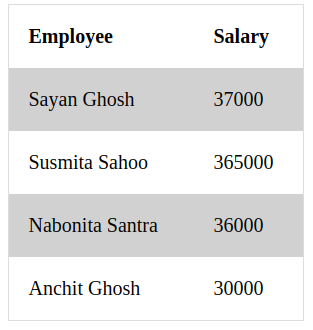
Share your thoughts in the comments
Please Login to comment...