What is EJS and why do I need it?
Last Updated :
18 Apr, 2024
In web development, there are many tools available for developers to choose from. Choosing the right tools and technologies can have a significant impact on the efficiency and functionality of projects. One of the popular tools in web development is EJS, which stands for Embedded JavaScript. EJS is a simple JavaScript templating language that generates HTML with plain JavaScript. In this article, we will cover what EJS is, why it is needed, its features, how to install it, and provide an example with output.
What is EJS
EJS or Embedded JavaScript is a template engine for JavaScript that is used for web development which allows users to generate dynamic HTML markup using JavaScript code within HTML templates. It is designed to simplify the process of rendering dynamic content in web applications. It contains a mixture of HTML and JavaScript which makes it easy to generate dynamic content based on data from your application.
Features of EJS
- Simple Syntax: EJS offers a straightforward syntax that combines HTML and JavaScript, making it easy to learn and use.
- Dynamic Content: EJS enables the generation of HTML and JavaScript content dynamically within HTML tags, enhancing flexibility in content creation.
- Layout and Partials: EJS supports layouts and partials, allowing users to break templates into reusable components, reducing code duplication and enhancing maintainability.
- Error Handling: EJS provides error messages that aid developers in debugging, improving the overall development experience.
Why Do You Need EJS?
- Dynamic HTML Generation: EJS allows you to generate dynamic HTML content based on variables, conditions, loops, and other JavaScript logic. This is particularly useful for rendering dynamic data fetched from databases or APIs.
- Code Reusability: By using EJS templates, you can create reusable components or partials that can be included in multiple pages. This promotes code modularity and reduces duplication in your web applications.
- Server-Side Rendering: With EJS, you can perform server-side rendering (SSR) of web pages. SSR is beneficial for SEO (Search Engine Optimization) as it allows search engines to crawl and index your content more effectively compared to client-side rendering (CSR) done by frameworks like React or Angular.
- Easy Integration with Node.js and Express.js: EJS integrates seamlessly with Node.js and Express.js, making it a popular choice for developers working on server-side JavaScript applications. It’s easy to set up and use within an Express.js project.
- Familiar Syntax: If you’re already familiar with HTML and JavaScript, learning and using EJS is straightforward. The syntax is similar to HTML with embedded JavaScript code enclosed in
<% %>
tags, making it accessible to developers of varying skill levels. - Template Inheritance and Layouts: EJS supports template inheritance and layouts, allowing you to create consistent layouts for your web pages. You can define a base layout and extend it in other templates, making it easier to maintain a consistent look and feel across your application.
How to Use EJS?
Step 1: Install EJS as a dependency in your project
npm install ejs
Step 2: Create a ‘views’ folder in your project directory if it doesn’t already exist. Inside the “views” folder, create a new file with a “.ejs” extension for example, “index.ejs”
Step 3: To integrate EJS with Express in an Express.js application, set EJS as the view engine in your Express app configuration. This configuration allows Express to use EJS for rendering views.
app.set('view engine', 'ejs');
Step 4: Render EJS Template, In your Express route handlers we render the EJS template using ‘res.render()’ and provide necessary data to be passed to the template.
res.render('hello', { name: 'Geeks' });
Project Structure:
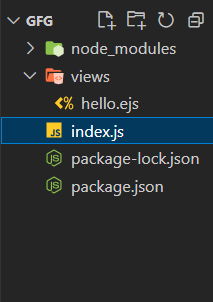
The updated dependencies in package.json file will look like:
"dependencies": {
"ejs": "^3.1.9",
"express": "^4.18.2"
}
Example: Implementation to showcase the use of ejs with an example.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,
initial-scale=1.0">
<title>EJS Example</title>
</head>
<body>
<h1>Hello, <%= name %>!</h1>
</body>
</html>
JavaScript
// index.js
const express = require('express');
const app = express();
const port = 3000;
app.set('view engine', 'ejs');
app.get('/', (req, res) => {
res.render('hello', { name: 'Geeks' });
});
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
Step to Run Application:Â Run the application using the following command from the root directory of the project
node index.js
Output:Â Your project will be shown in the URL http://localhost:3000/
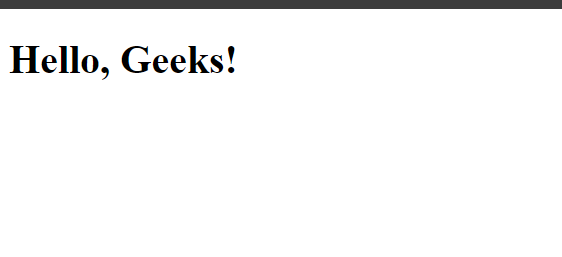
Share your thoughts in the comments
Please Login to comment...