Server Side Rendering using Express.js And EJS Template Engine
Last Updated :
19 Mar, 2024
Server-side rendering involves generating HTML on the server and sending it to the client, as opposed to generating it on the client side using JavaScript. This improves initial load time, and SEO, and enables dynamic content generation. Express is a popular web application framework for NodeJS, and EJS is a simple templating language that lets you generate HTML with plain JavaScript.
Basic Approach:
- Using Express with EJS templating engine to render HTML on the server side.
- In this approach, we set up an Express server with EJS as the templating engine. We define routes and render EJS templates directly. Data can be passed to the templates as variables.
Advanced Approach (with Data Fetching):
- Utilizing asynchronous data fetching within Express routes to dynamically render content with EJS.
- This approach extends the basic one by incorporating asynchronous data fetching within Express routes. This could involve fetching data from databases, external APIs, or other sources before rendering the EJS templates.
Steps to Create Application ( And Installing Required Modules):
Step 1: Create a new directory for your project:
mkdir express-ssr
Step 2: Navigate into the project directory:
cd express-ssr
Step 3: Initialize npm (Node Package Manager) to create a package.json file:
npm init -y
Step 4: Install required modules (Express and EJS) using npm:
npm install express ejs
Project Structure:
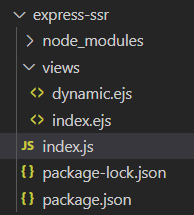
The updated dependencies in package.json file will look like:
"dependencies": {
"ejs": "^3.1.9",
"express": "^4.18.3"
}
Example: Below is an example of ServerSide Rendering With Express and EJS Templates.
HTML
//views/index.ejs
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Express SSR with EJS</title>
</head>
<body>
<h1>Hello, <%= name %>!</h1>
</body>
</html>
HTML
//views/dynamic.ejs
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Express SSR with EJS</title>
</head>
<body>
<h1>Dynamic Content: <%= data %></h1>
</body>
</html>
JavaScript
const express = require('express');
const app = express();
const path = require('path');
// Set the view engine to EJS
app.set('view engine', 'ejs');
app.set('views', path.join(__dirname, 'views'));
// Basic Approach: Render EJS template with static data
app.get('/', (req, res) => {
res.render('index', { name: 'World' });
});
/*
Advanced Approach: Render EJS template
with asynchronously fetched data
*/
app.get('/dynamic', async (req, res) => {
const dynamicData = await fetchData();
res.render('dynamic', { data: dynamicData });
});
// Function to simulate asynchronous data fetching
async function fetchData() {
return new Promise(resolve => {
setTimeout(() => {
resolve('Dynamic Content');
}, 1000);
});
}
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Start your server using the following command.
node index.js
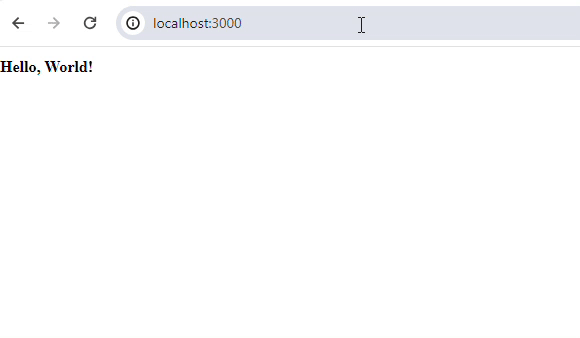
Share your thoughts in the comments
Please Login to comment...