Create a Quick access Pin using HTML , CSS and JavaScript
Last Updated :
17 Nov, 2023
Quick Access Pins are a useful feature in web applications that allow users to save and quickly access their favorite or frequently visited websites, tools, or pages. These pins provide a convenient and efficient way to navigate a website or web application. In this post, we will create a Quick Access PIN using HTML, CSS, and JavaScript.
Prerequisite:
Approach:
- create a static HTML structure with predefined pinned items.
- Design a simple layout with the pinned items.
- Use HTML to create pins and provide links.
- Style the pins using the CSS for an attractive appearance.
- Use JavaScript for the navigation when a pin is clicked.
Example: Below is the basic implementation of the above project
Javascript
const pinContainer =
document.getElementById( 'pin-container' );
const pinnedItems = [
{
title: 'Website' ,
},
{
title: 'Email' ,
url: 'mailto:example@example.com' , icon: '✉️'
},
{
title: 'Notes' ,
},
];
function create(item) {
const pin = document.createElement( 'div' );
pin.classList.add( 'pin' );
pin.innerHTML =
`<div>${item.icon}</div><div>${item.title}</div>`;
pin.addEventListener( 'click' , () => {
window.location.href = item.url;
});
pinContainer.appendChild(pin);
}
pinnedItems.forEach(create);
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >The Quick Access Pin</ title >
< link rel = "stylesheet" href = "style.css" >
</ head >
< body >
< header >
< h1 >Quick Access Pin</ h1 >
</ header >
< main >
< div class = "pin-container" id = "pin-container" >
</ div >
</ main >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
body {
font-family : Arial , sans-serif ;
background-color : #C0C0C0 ;
margin : 0 ;
padding : 0 ;
display : flex;
flex- direction : column;
align-items: center ;
text-align : center ;
}
header {
background-color : #800080 ;
color : white ;
padding : 1 rem;
width : 100% ;
}
main {
display : flex;
align-items: center ;
flex- direction : column;
padding : 2 rem;
}
.pin-container {
display : flex;
flex-wrap: wrap;
gap: 20px ;
}
.pin {
width : 150px ;
height : 150px ;
background-color : #3498db ;
color : white ;
display : flex;
flex- direction : column;
justify- content : center ;
align-items: center ;
border-radius: 15px ;
cursor : pointer ;
transition: background-color 0.3 s;
}
.pin:hover {
background-color : #1e6a9d ;
}
|
Output:
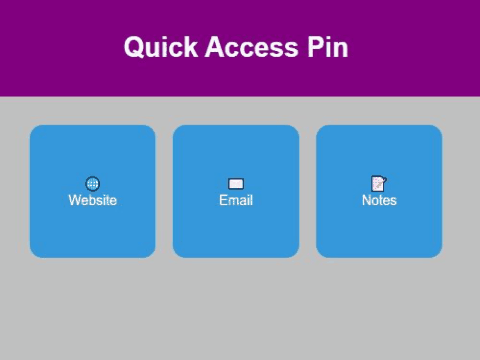
Share your thoughts in the comments
Please Login to comment...