do…while Loop in C
Last Updated :
24 Feb, 2023
Loops in C language are the control flow statements that are used to repeat some part of the code till the given condition is satisfied. The do-while loop is one of the three loop statements in C, the others being while loop and for loop. It is mainly used to traverse arrays, vectors, and other data structures.
What is do…while Loop in C?
The do…while in C is a loop statement used to repeat some part of the code till the given condition is fulfilled. It is a form of an exit-controlled or post-tested loop where the test condition is checked after executing the body of the loop. Due to this, the statements in the do…while loop will always be executed at least once no matter what the condition is.
Syntax of do…while Loop in C
do {
// body of do-while loop
} while (condition);
How to Use do…while Loop in C
The following example demonstrates the use of do…while loop in C programming language.
C
#include <stdio.h>
int main()
{
int i = 0;
do {
printf ( "Geeks\n" );
i++;
} while (i < 3);
return 0;
}
|
How does the do…while Loop works?
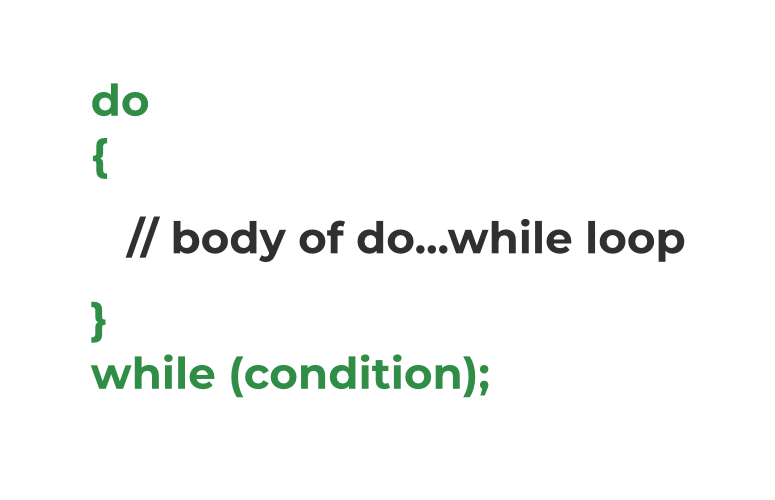
Syntax Structure of do while loop
The working of the do…while loop is explained below:
- When the program control first comes to the do…while loop, the body of the loop is executed first and then the test condition/expression is checked, unlike other loops where the test condition is checked first. Due to this property, the do…while loop is also called exit controlled or post-tested loop.
- When the test condition is evaluated as true, the program control goes to the start of the loop and the body is executed once more.
- The above process repeats till the test condition is true.
- When the test condition is evaluated as false, the program controls move on to the next statements after the do…while loop.
As with the while loop in C, initialization and updation is not a part of the do…while loop syntax. We have to do that explicitly before and in the loop respectively.
The flowchart below shows the visual representation of the flow of the do…while loop in C.
C do…while Loop Flowchart
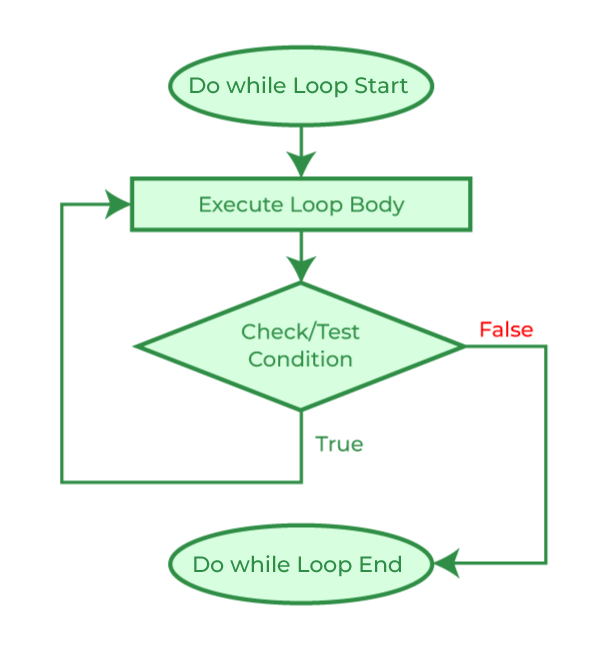
Flowchart of do…while Loop in C
Nested do…while Loop in C
As with other loops, we can also nest one do…while loop into another loop. It is demonstrated using the following C program.
Example of Nested do…while Loop in C:
C
#include <stdio.h>
int main()
{
int i = 0, j;
int count = 0;
do {
j = 0;
do {
printf ( "%d " , count++);
j++;
} while (j < 3);
printf ( "\n" );
i++;
} while (i < 3);
return 0;
}
|
To know more about nested loops in C, refer to this article – Nested Loops in C with Examples
Examples of do…while Loop in C
Example 1. C Program to demonstrate the behavior of do…while loop if the condition is false from the start.
C
#include <stdbool.h>
#include <stdio.h>
int main()
{
bool condition = false ;
do {
printf ( "This is loop body." );
} while (condition);
return 0;
}
|
Output
This is loop body.
As we can see, even when the condition is false at the start, the loop body is executed once. This is because in the do…while loop, the condition is checked after going through the body so when the control is at the start,
- It goes through the loop body.
- Executes all the statements in the body.
- Checks the condition which turns out to be false.
- Then exits the loop.
Example 2. C Program to print Multiplication Table of N using do…while loop
The following example demonstrates the use of do…while loop for printing the multiplication table of N.
C
#include <stdio.h>
int main()
{
int N = 5, i = 1;
do {
printf ( "%d\tx\t%d\t=\t%d\n" , N, i, N * i);
} while (i++ < 10);
return 0;
}
|
Output
5 x 1 = 5
5 x 2 = 10
5 x 3 = 15
5 x 4 = 20
5 x 5 = 25
5 x 6 = 30
5 x 7 = 35
5 x 8 = 40
5 x 9 = 45
5 x 10 = 50
Difference between while and do…while Loop in C
The following table lists the important differences between the while and do…while Loop in C.
while Loop
|
do…while Loop
|
The test condition is checked before the loop body is executed. |
The test condition is checked after executing the body. |
When the condition is false, the body is not executed not even once. |
The body of the do…while loop is executed at least once even when the condition is false. |
It is a type of pre-tested or entry-controlled loop. |
It is a type of post-tested or exit-controlled loop. |
Semicolon is not required. |
Semicolon is required at the end. |
To know more about these differences, please refer to this article – Difference between while and do-while loop in C, C++, Java
Conclusion
In conclusion, the use of the only exit-controlled loop in C, the do…while loop is also to iterate a particular part of code but the way it works makes it different from entry-controlled loops such as the while loop and the for loop. It is useful in cases where we need to execute the statement inside the loop body at least once such as in traversing circular linked lists.
FAQs on C do…while Loops
1. How many types of loops are there in C?
Ans: There are 3 types of loops in C language:
- for Loop
- while Loop
- do…while Loop
2. What are the entry-controlled or pre-tested loops?
Ans: The entry-controlled loops or pre-tested loops are those loops in which the loop condition is checked before executing the body of the loop.
3. What are the exit-controlled or post-tested loops?
Ans: The exit-controlled loops or post-tested loops are those loops in which the program control comes to the body of the loop before checking the loop condition.
4. Which loop is guaranteed to execute at least once?
Ans: The do…while loop is guaranteed to execute the statements in the body of the loop at least once as it is a type of exit-controlled loop.
5. Can we skip braces in C do…while loop syntax if there is only one statement in the body?
Ans: No, we cannot skip braces in C do…while syntax even if there is only a single statement unlike while and for loop.
6. How to create an infinite loop in C using the do…while loop?
Ans: We can create an infinite loop in C by specifying a condition that will always be true as the loop condition. The below program demonstrates how to do that:
C
#include <stdio.h>
int main()
{
do {
printf ( "gfg " );
} while (1);
return 0;
}
|
Output
gfg gfg gfg gfg gfg gfg gfg .... (infinite)
Related Articles:
Share your thoughts in the comments
Please Login to comment...