Building Custom Hooks Library in React
Last Updated :
01 Apr, 2024
A custom hooks library is a collection of custom hooks in React that are designed to encapsulate reusable logic and functionality. These hooks are typically organized into a single library or module, making it easy to share and reuse them across different components and projects.
Prerequisites
What are Custom Hooks ?
Custom Hooks are JavaScript functions that follow a specific naming convention (`useSomething`) and leverage existing React hooks to encapsulate reusable logic. They allow you to abstract complex behavior into a reusable function that can be shared across multiple components.
Approach to Create custom hooks library in React
Here are the some steps to building custom hooks library within react application.
Step 1: Create react application
npx create-react-app <-foldername->
Step 2: Move to the application directory
cd <-foldername->
Step 3: update App.js with following code.
JavaScript
// App.js
import { useState } from "react";
import { useDivBgColor, useBodyBgColor } from "./custom-hooks/your-custom-hook-lib"; // Import custom hooks
function App() {
const [value, setValue] = useState("green");
const [bgcolor, setBgColor] = useDivBgColor("green"); // Use custom hook for div background color
const toggleBodyBgColor = useBodyBgColor();
const handleInput = (e) => {
setValue(e.target.value);
setBgColor(e.target.value);// Toggle background color of div
};
return (
<>
<div className='main'>
<div>
<div className="background" style={{ border:"2px solid red", background: bgcolor, color: "white", height: "100px", width: "100px", margin: "25px 0 10px 25px" }}> </div>
<input type="text" placeholder="Enter color name" value={value} onChange={handleInput} />
</div>
<div style={{marginTop:"12px"}}>
<button onClick={toggleBodyBgColor}>Toggle body background color</button>
</div>
</div>
</>
);
}
export default App;
This App.js file importing custom-hooks library where we have defined two custom-hooks, `useDivBgColor` for changing div background color and `useBgBodyColor` for changing body background color.
Project Structure:
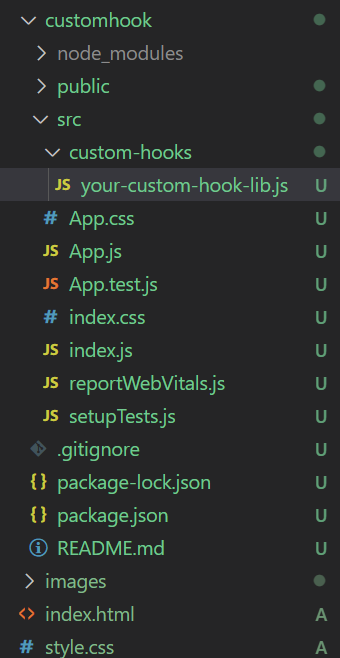
Step 4: As we build custom hooks library and already imported to App.js.
To create a Custom Hooks Library, follow these rules:
- Define your custom hooks as standalone functions prefixed with `use`.
- Import any necessary React hooks within your custom hooks.
- Ensure your custom hooks are pure functions and don’t rely on component-specific state or props.
- Export each custom hook from your library for use in other components.
- Code for Custom-hooks-library.
JavaScript
// your-custom-hooks-lib.js
import { useState, useEffect } from "react";
// Custom hook for toggling background color of a div
export function useDivBgColor(initialColor) {
const [color, setColor] = useState(initialColor);
useEffect(() => {
setColor(initialColor);
}, [initialColor]); // Reset color when initialColor changes
return [color, setColor];
}
// Custom hook for toggling background color of the body
export function useBodyBgColor() {
const [isToggled, setIsToggled] = useState(false);
const toggleBgColor = () => {
if (isToggled) {
document.body.style.backgroundColor = "white";
} else {
document.body.style.backgroundColor = "black";
}
setIsToggled((prevIsToggled) => !prevIsToggled);
};
return toggleBgColor;
}
useDivBgColor hook uses two hooks- useState and useEffect. and soon as input field in App.js have some color name, it uses custom hooks that have function that changes Div Background color, default color is green. And useBodyBgColor hook using useState hook that toggle body background color when we click button in App.js. Meanwhile we are also exporting them so used by App.js.
Step 5: Run your Application.
npm run start
Output :
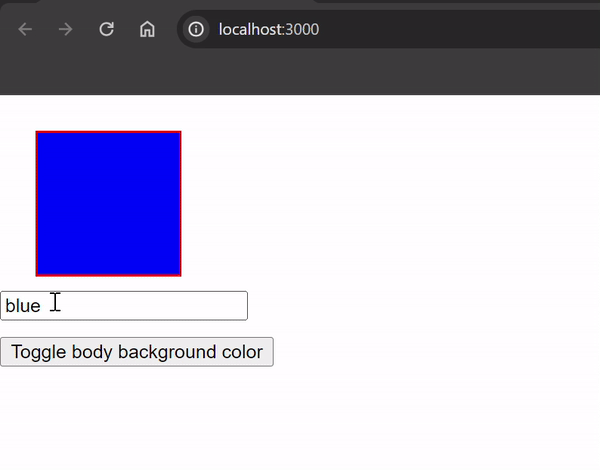
Share your thoughts in the comments
Please Login to comment...