Are Class Components still useful in React ?
Last Updated :
26 Mar, 2024
With the rise of functional components and hooks in React, class components have taken a backseat in modern React development. However, there are still scenarios where class components offer advantages over functional components.
In this article, we’ll explore the continued relevance of class components in React and discuss situations where they might still be preferred.
Scenarios to use Class Components:
1. Complex State Management:
Class components provide a straightforward way to manage complex state logic, especially when dealing with deeply nested state or multiple interconnected pieces of state. The `setState()` method in class components allows for granular control over state updates, making them suitable for applications with intricate state management requirements.
2. Lifecycle Methods:
Class components offer a wide range of Lifecycle Methods such as `componentDidMount()`, `componentDidUpdate()`, and `componentWillUnmount()`. These lifecycle methods are useful for performing actions such as data fetching, subscriptions, and cleanup, which are not as straightforward to achieve with functional components and hooks.
3. Codebase Compatibility:
In existing React codebases that heavily rely on class components, transitioning to functional components and hooks may not always be feasible or practical. Class components allow for gradual migration and coexistence with functional components, enabling teams to adopt modern React practices at their own pace without disrupting existing code.
4. Third-party Library Integration:
Some third-party libraries or APIs may rely on class components or expect certain lifecycle methods to be present. While many libraries have adapted to support functional components and hooks, there are still cases where class components may be preferred for seamless integration with existing libraries or APIs.
5. Personal Preference and Familiarity:
For developers who are more comfortable and experienced with class components, choosing to use them may simply be a matter of personal preference. Class components offer a familiar programming model for developers who have been working with React for a long time, and they may find them easier to understand and reason about.
Fetching Data using Lifecycle Methods
In this example, UserDataFetcher is a class component that makes use of the componentDidMount lifecycle method to perform a fetch request to an API for user data when the component is first mounted to the DOM. The componentWillUnmount lifecycle method is also defined to demonstrate where you might include cleanup logic, such as cancelling fetch requests to prevent memory leaks in more complex applications.
JavaScript
import React, { Component } from "react";
class UserDataFetcher extends Component {
constructor(props) {
super(props);
this.state = {
userData: null,
isLoading: true,
error: null,
};
}
componentDidMount() {
fetch("https://jsonplaceholder.typicode.com/users/1")
.then((response) => {
if (!response.ok) {
throw new Error("Network response was not ok");
}
return response.json();
})
.then((data) => this.setState({ userData: data,
isLoading: false }))
.catch((error) => this.setState({error,isLoading: false }));
}
componentWillUnmount() {
// Perform any necessary cleanup here,
// such as aborting fetch requests
// or unsubscribing from external data sources.
console.log(`Component is being unmounted and
cleanup is in progress.`);
}
render() {
const { userData, isLoading, error } = this.state;
if (isLoading) {
return <div>Loading...</div>;
}
if (error) {
return <div>Error: {error.message}</div>;
}
return (
<div>
<h1>User Data</h1>
<p>Name: {userData.name}</p>
<p>Email: {userData.email}</p>
{/* Render more user details as needed */}
</div>
);
}
}
export default UserDataFetcher;
Output:
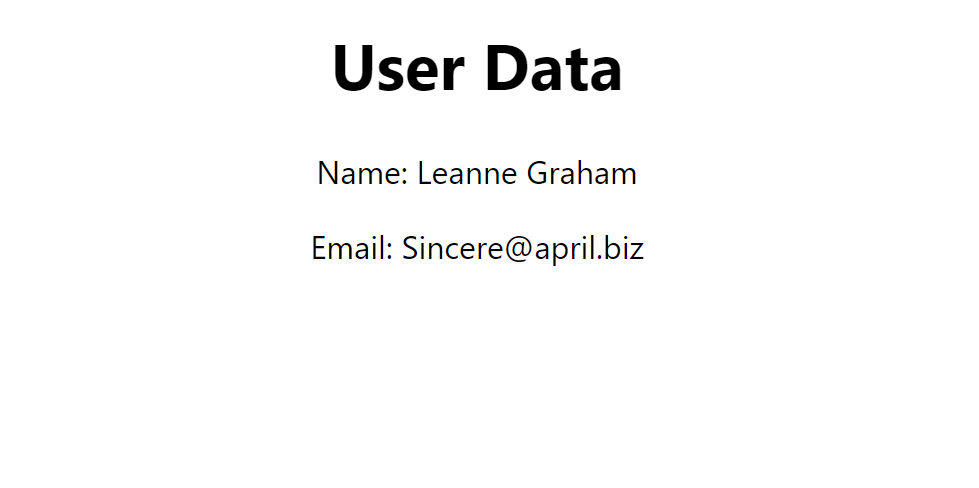
Conclusion
While functional components and hooks have become the preferred choice for most React development due to their simplicity and flexibility, class components still have their place in certain scenarios. Whether it’s managing complex state, leveraging lifecycle methods, ensuring compatibility with existing codebases, integrating with third-party libraries, or catering to personal preferences, class components continue to offer value in the React ecosystem.
Share your thoughts in the comments
Please Login to comment...