3D Multiple Regression Graph with rgl package in R
Last Updated :
17 Oct, 2023
In this article, we are going to walk through the process of creating a 3D multiple regression graph using the rgl package in R programming language in detail.
What is Multiple Regression?
Regression analysis is a powerful statistical tool for understanding the relationship between variables. One of the well-known regression techniques is Linear Regression, which deals with predicting a dependent variable using only one independent variable.
Multiple Linear Regression also known as Multiple Regression, is the prediction of the dependent variable using more than one independent variable. In simple words, it is a statistical technique used to analyze the relationship between a single dependent variable and multiple independent variables.
The equation of multiple regression is:
Y = b0 + b1 * x1 + b2 * x2 + b3 * x3 + …… bn * xn
Where,
Y -> dependent variable
x1, x2, x3, …… xn -> multiple independent variables
n -> number of features
b0 -> y- intercept
bn -> regression coefficient of nth feature
RGL Package
The rgl package in R is a great tool for creating interactive 3D visualizations, which makes it ideal for representing complex relationships in multiple regression models. It enables users to explore data interactively, visualize regression planes, and customize plots, thereby enhancing the understanding of the relationships between multiple dependent and independent variables.
Steps in Creating 3D Plot
Let us see step by step, how we can create a 3D multiple regression graph in R using the rgl package.
Install and load the rgl package
First, we install and then load the RGL package in our R script.
R
install.packages ( "rgl" )
library (rgl)
|
The rgl Package in R provides us with various functionalities to customize our 3D plots.
Preparing Data
We’ll start by generating some sample data for demonstration purposes. Here we’ll create two independent variables, x1 and x2, and one dependent variable, y.
Replace this sample data with your own dataset when working on your specific analysis.
R
set.seed (123)
n <- 100
x1 <- rnorm (n)
x2 <- rnorm (n)
y <- 2 * x1 + 3 * x2 + rnorm (n)
|
rnorm is the R function that simulates random variates having a specified normal distribution.
Fitting the Multiple Linear Regression Model
Next, we fit a multiple linear regression model to our data using the ‘lm’ function. This model will help us understand how x1 and x2 collectively influence the dependent variable y.
lm() function which stands for linear model. This function can be used to create a simple regression model. The same function can be used to generate linear regression model, with change of parameters.
R
lm_model <- lm (y ~ x1 + x2)
|
The model is specified using the formula y ~ x1 + x2, where the dependent variable y is being estimated using two independent variables, x1 and x2, in a multiple linear regression analysis.
Creating the 3D Plot
Now, let’s create an interactive 3D plot using the rgl package. We’ll start by initializing an empty 3D plot, adding data points, and then visualizing the regression plane.
#Create an empty 3D plot
open3d()
#3d plot using the lm_model
plot3d(lm_model)
# Add a title
title3d(“Multiple Linear Regression 3D Plot”)
Examples of Creating 3D Multiple Regression Graph
Now let us see an example to understand the concept. The code follows the same procedure as given in the above steps.
A 3D Plot for Multiple Linear Regression without Squared Variable
R
library (rgl)
open3d ()
lm_model= lm (mpg ~ wt + qsec, data = mtcars)
plot3d (lm_model, plane.col= 'red' )
title3d ( "Multiple Linear Regression 3D Plot" )
play3d ( spin3d (axis = c (0, 0, 1)), duration = 30)
|
Output:
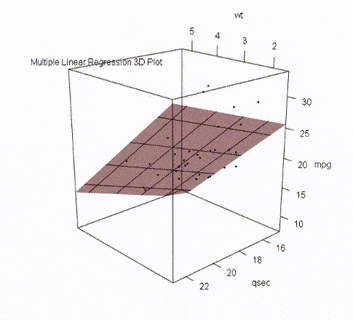
3D Multiple Regression Graph with rgl package in R
The outputs for this code will appear in a new small window because of the function ‘open_3d’ and not the console of R studio.
It looks like this:
-1024.png)
Output in R- studio
- Firstly, it installs and loads the ‘rgl‘ package, which enables 3D visualization capabilities. Next, it opens a new 3D plotting window because of ‘open3d‘ for visualization. The code then proceeds to create a multiple linear regression model (`lm_model`) using the ‘mtcars‘ dataset, with ‘mpg‘ (miles per gallon) as the response variable and ‘wt‘ (weight) and ‘qsec‘ (quarter mile time) as predictor variables.
- This model does not include a squared term, meaning it assumes a linear relationship between the predictors and the response. This implies, it may not effectively capture nonlinear patterns in the data. The code then generates a 3D plot of the regression model, representing the regression plane in red because of ‘plot3d‘ function, and labels it as “Multiple Linear Regression 3D Plot” using the ‘title3d’ function. Lastly, it adds an animation using the ‘play3d‘ function that rotates the 3D plot around the z-axis for 30 seconds, allowing for a dynamic exploration of the plot from various angles. You may even change the duration of the animation to occur.
- We can observe from the output that the plot shows the regression plot, which means the plane there is going to predict the mpg of the car using the input wt and qsec when provided.
A 3D Plot for Multiple Linear Regression with Squared Variable
R
library (rgl)
open3d ()
lm_model= lm (mpg ~ wt + I (wt^2) + qsec, data = mtcars)
plot3d (lm_model, plane.col= 'green' )
title3d ( "Multiple Linear Regression 3D Plot" )
play3d ( spin3d (axis = c (0, 0, 1)), duration = 30)
|
Output:
.gif)
3D Multiple Regression Graph with rgl package in R
In the above code, we used the same dataset of mtcars. The output looks like this in the R studio:
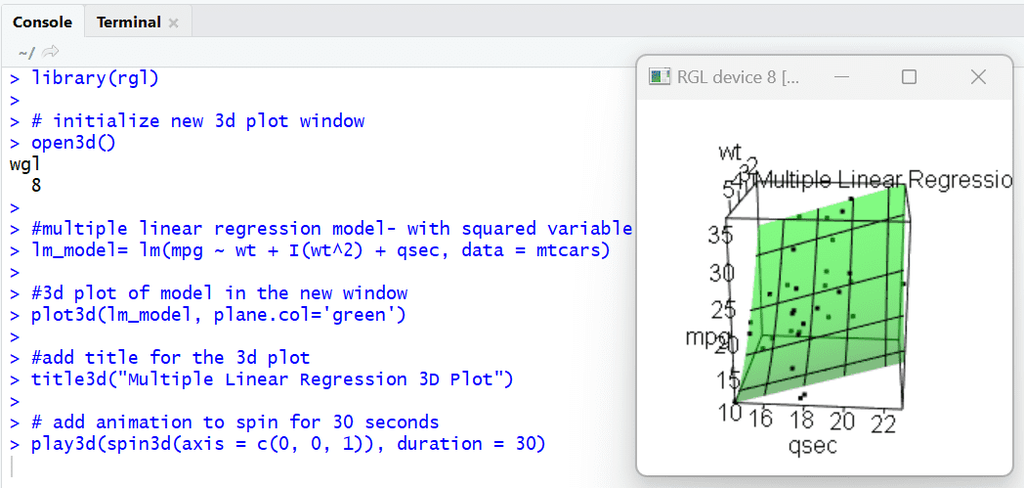
Output in R studio
- We used ‘wt^2‘ as one of the independent variable parameters, which is the square of ‘wt‘ variable. This may be necessary many times. In some real-world data, the relationship between the dependent variable and an independent variable may not be linear. Squaring the variable allows us to capture nonlinear relationships. Hence, we squared it. We can see, in the graph that a non-linearity is produced in the graph, which touches most of the points in the plot, thereby a better prediction/regression model.
Visualizing Multiple Linear Regression in 3D with R
We are going to create our own dataset to use for the multiple regression by using vectors concept in R and create a graph. The data we are going to use has two independent variables – age and experience and the dependent variable is income. Here the procedure same as the above codes.
Loading Packages
Building Linear Regression Model
R
age <- c (25,30,47,32,43,51,28,33,37,39,29,47,54,51,44,41,58,23,44,37)
exp <- c (1,3,2,5,10,7,5,4,5,8,1,9,5,4,12,6,17,1,9,10)
income <- c (30450,35670,31580,40130,47830,41630,41340,37650,40250,45150,27840,46110,
36720,34800,5130,38900,63600,30870,44190,48700)
lm_model= lm (income ~ age + exp)
print (lm_model)
|
Output:
Call:
lm(formula = income ~ age + exp)
Coefficients:
(Intercept) age exp
28982.46 68.95 1082.37
Visualizing Regression Graph
R
open3d ()
plot3d (lm_model, plane.col= 'blue' )
title3d ( "Multiple Linear Regression 3D Plot" )
play3d ( spin3d (axis = c (0, 0, 1)), duration = 30)
|
Output:
.gif)
3D Multiple Regression Graph with rgl package in R
The output shown here helps us to predict the income when the experience and income is given. We can also observe that the dataset had an outlier, which may cause the model performance to decrease.
The output in R-studio looks like this:
-(1).png)
Output in R studio
Conclusion
By following the steps outlined in this article, you can effectively generate interactive 3D plots that help you gain insights into your regression models.
Share your thoughts in the comments
Please Login to comment...