Working with Forms in React
Last Updated :
17 Nov, 2023
React Forms are an important part of most web applications. They allow users to input data and interact with the application. Forms are used to collect the data from the user and process it as required whether in login-signup forms or surveys etc.
Prerequisites:
These are the approaches to use the Forms in React JS.
Steps to Create React Application:
Step 1: Create a React application using the following command.
npx create-react-app gfg
Step 2: After creating your project folder(i.e. gfg), move to it by using the following command.
cd gfg
Project Structure:
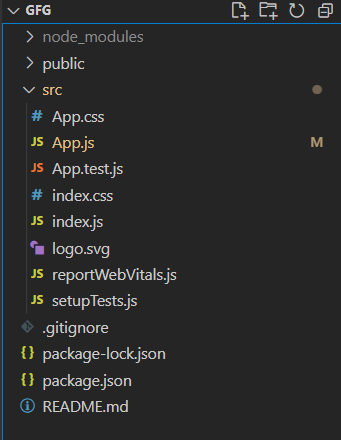
The first thing we need to do is create a form. We can do this by using the <form> tag. Inside of the <form> tag, we can add any number of input fields. Input Fields are the basic elements of a form. They allow the user to input data. We can add an Input field in React using the <input> tag but to change its value we have to use the useState hook.
Example: This example implements a basic form with the HTML input and react hook to store the data in states..
Javascript
import React, { useState } from "react" ;
export default function App() {
const [value, setValue] = useState( "" );
return (
<div>
<h2>GeeksforGeeks - ReactJs Form</h2>
<form>
<label>
Input Field:-
<input
value={value}
onChange={(e) => {
setValue(e.value);
}}
/>
</label>
</form>
</div>
);
}
|
Step to run the application: Start the application using the following command:
npm run start
Output: This output will be visible on the http://localhost:3000/ on the browser window.
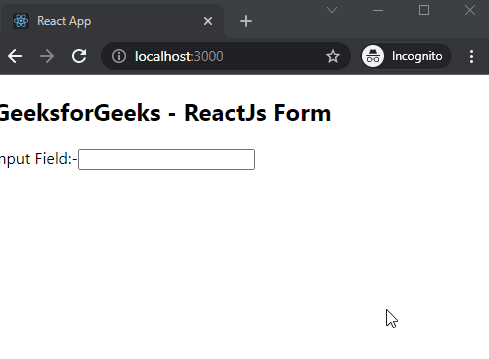
Method 2: Form Using TextArea field
A TextArea is a larger text input field. It allows the user to enter multiple lines of text. We can add a TextArea in React using the <textarea> tag but to change its value we have to use the useState hook.
Example: This example implements a form using the TextArea input field.
Javascript
import React, { useState } from "react" ;
export default function App() {
const [value, setValue] = useState( "" );
return (
<div>
<h2>GeeksforGeeks - ReactJs Form</h2>
<form>
<label>
Input Field:-
<textarea
value={value}
onChange={(e) => {
setValue(e.value);
}}
/>
</label>
</form>
</div>
);
}
|
Step to run the application: Start the application using the following command:
npm run start
Output: This output will be visible on the http://localhost:3000/ on the browser window.:
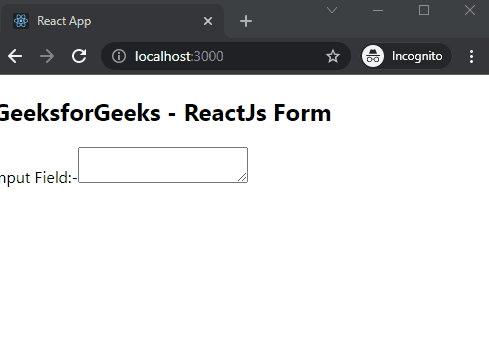
A Select is a field that allows the user to select one option from a list. We can add a Select Field in React using the <select> tag.
Example: This example implemens a form using the select and options input.
Javascript
import React, { useState } from "react" ;
export default function App() {
const [value, setValue] = useState( "" );
return (
<div>
<h2>GeeksforGeeks - ReactJs Form</h2>
<form>
<label>
Select Field:-
<select
value={value}
onChange={(e) => {
setValue(e.value);
}}
>
<option value= "gfg1" >GfG1</option>
<option value= "gfg2" >GfG2</option>
<option value= "gfg3" >GfG3</option>
<option value= "gfg4" >GfG4</option>
</select>
</label>
</form>
</div>
);
}
|
Step to run the application: Start the application using the following command:
npm run start
Output: This output will be visible on the http://localhost:3000/ on the browser window.:
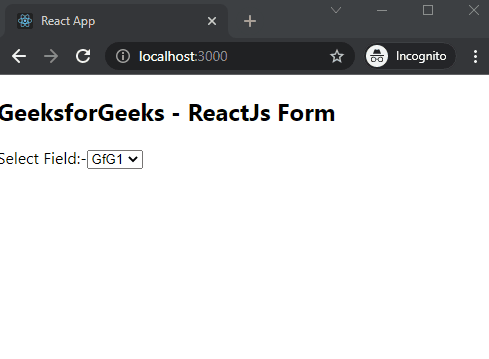
Share your thoughts in the comments
Please Login to comment...