How to work with Form Elements in TypeScript ?
Last Updated :
09 Apr, 2024
When developing web applications, forms serve as essential components for collecting user input. Form elements can be called the building blocks of a form. They allow users to provide input, make selections, and interact with the application, enabling various functionalities such as data submission, validation, and retrieval. TypeScript which is a typed superset of JavaScript provides us with several features for managing form elements.
In typeScript it’s crucial to use correct types, therefore we use HTMLInputElement as a type for input elements. By using HTMLInputElement, TypeScript provides type checking and auto-completion for properties and methods that are available on input elements such as value, checked, disabled, etc.
Example: To demonstrate handling the form submission in typescript and finally show the submitted fields on the browser.index.html
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
</head>
<body>
<h1>User Feedback</h1>
<form id="myForm">
<div>
<h3>Enter username</h3>
<input type="text"
name="username"
id="username">
</div>
<div>
<h3>Type Feedback</h3>
<textarea name="feedback"
id="feedback"
cols="40"
rows="7"></textarea>
</div>
<button type="submit">Add</button>
</form>
<div>
<h3 id="user"></h3>
<p id="feed"></p>
</div>
<script src="script.js"></script>
</body>
</html>
JavaScript
/script.ts
interface FormElements {
username: HTMLInputElement;
feedback: HTMLInputElement;
}
const form = document
.getElementById('myForm') as HTMLFormElement;
const user = document
.getElementById('user') as HTMLDivElement;
const feed = document
.getElementById('feed') as HTMLDivElement;
const elements: FormElements = {
username: document
.getElementById('username') as HTMLInputElement,
feedback: document
.getElementById('feedback') as HTMLInputElement
};
form
.addEventListener('submit', (event) => {
event
.preventDefault();
const usernameValue = elements
.username.value.trim();
const feedbackValue = elements
.feedback
.value
.trim();
user.innerHTML = usernameValue;
feed.innerHTML = feedbackValue;
});
In the above TypeScript code we first get references to the form element, input element for username, textbox element for feedback and output heading and paragraph elements. Then, we used a type assertion as HTMLInputElement for form elements. We added an event listener on the form and used ‘value ‘ property on input elements to retrieve their values. Finally, we embedded the values to the output heading element and the paragraph element.
Compile the the above TypeScript code into the JavaScript code using the following command.
tsc script.ts
Output:
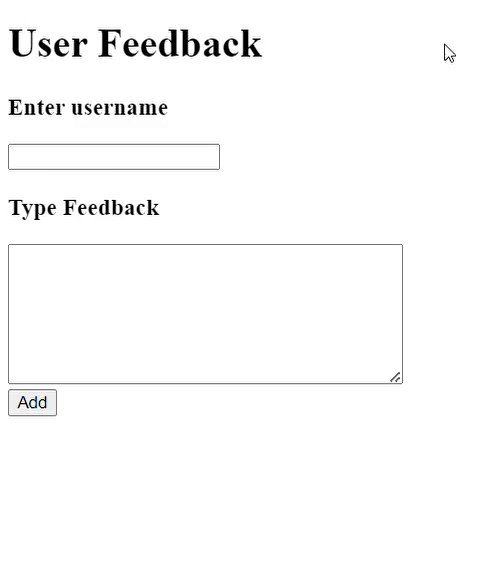
output for example 1
In this we handle the input elements we also validate the values. Here we’ll allow user to enter the username and the password and then we’ll validate whether both the fields are present or not. If not then we’ll throw an error.
Example: To demonstrate handling form submission with validation using form element in TypeScript.
index.html
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Form Example</title>
</head>
<body>
<form id="myForm">
<input type="text"
id="username"
name="username"
placeholder="Enter your username">
<input type="password"
id="password"
name="password"
placeholder="Enter your password">
<button type="submit">Submit</button>
</form>
<div id="result"></div>
<p id="error" style="color: red"></p>
<script src="script.js"></script>
</body>
</html>
JavaScript
script.ts
const form = document
.getElementById('myForm') as HTMLFormElement;
const error = document
.getElementById('error') as HTMLParagraphElement;
form
.addEventListener('submit', (event) => {
event
.preventDefault();
const username = (document
.getElementById('username') as HTMLInputElement)
.value;
const password = (document
.getElementById('password') as HTMLInputElement)
.value;
if (username
.trim() === '' || password.trim() === '') {
error
.innerHTML = 'Please fill in all fields';
}
});
Output:
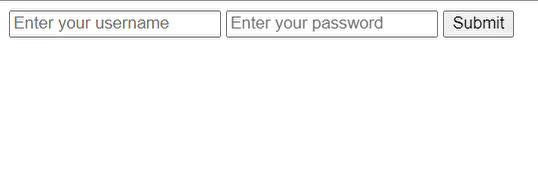
output for example 2
Share your thoughts in the comments
Please Login to comment...