Why Array Data Structures is needed?
Last Updated :
16 Feb, 2024
Assume there is a class of five students and if we have to keep records of their marks in the examination then, we can do this by declaring five variables individual and keeping track of records.
C++
#include <iostream>
using namespace std;
int main()
{
int v1 = 10;
int v2 = 20;
int v3 = 30;
int v4 = 40;
int v5 = 50;
return 0;
}
|
Java
public class Main {
public static void main(String[] args) {
int v1 = 10 ;
int v2 = 20 ;
int v3 = 30 ;
int v4 = 40 ;
int v5 = 50 ;
}
}
|
Python3
def main():
v1 = 10
v2 = 20
v3 = 30
v4 = 40
v5 = 50
if __name__ = = "__main__" :
main()
|
C#
#pragma warning disable CS0219
using System;
class Program
{
static void Main( string [] args)
{
int v1 = 10;
int v2 = 20;
int v3 = 30;
int v4 = 40;
int v5 = 50;
}
}
#pragma warning restore CS0219
|
Javascript
let v1 = 10;
let v2 = 20;
let v3 = 30;
let v4 = 40;
let v5 = 50;
|
But what if the number of students becomes very large
It would be challenging to manipulate and maintain the data.
What it means is that we can use normal variables (v1, v2, v3, …) when we have a small number of objects. But if we want to store a large number of instances, it becomes difficult to manage them with normal variables.
The idea of an array is to represent many instances in one variable.
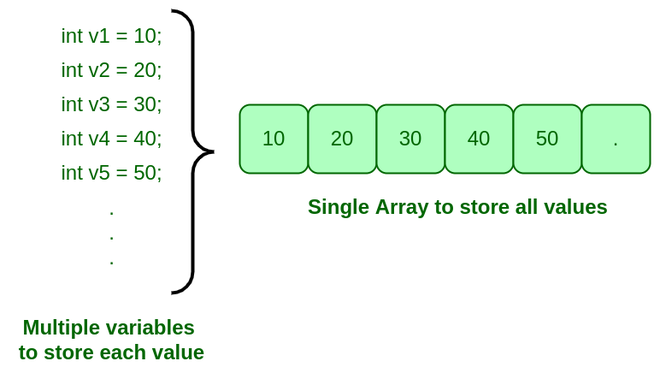
Need for Arrayc
Advantages of array data structure:
- Efficient access to elements:Â Arrays provide direct and efficient access to any element in the collection. Accessing an element in an array is an O(1) operation, meaning that the time required to access an element is constant and does not depend on the size of the array.
- Fast data retrieval:Â Arrays allow for fast data retrieval because the data is stored in contiguous memory locations. This means that the data can be accessed quickly and efficiently without the need for complex data structures or algorithms.
- Memory efficiency:Â Arrays are a memory-efficient way of storing data. Because the elements of an array are stored in contiguous memory locations, the size of the array is known at compile time. This means that memory can be allocated for the entire array in one block, reducing memory fragmentation.
- Versatility:Â Arrays can be used to store a wide range of data types, including integers, floating-point numbers, characters, and even complex data structures such as objects and pointers.
- Easy to implement:Â Arrays are easy to implement and understand, making them an ideal choice for beginners learning computer programming.
- Compatibility with hardware:Â The array data structure is compatible with most hardware architectures, making it a versatile tool for programming in a wide range of environments.
Share your thoughts in the comments
Please Login to comment...