What is the use of Bootstrap Datepicker in Angular?
Last Updated :
10 Jan, 2024
In this article, we will see the use of Bootstrap Datepicker in Angular. The Datepicker is used to make a component that will be shown by using a calendar and we can select the date from it. Adding Bootstrap to the Angular Project can make the better UI design, along with providing some in-built classes for Date that help us to implement it directly.
Bootstrap Datepicker in Angular can be used in the following ways:
- Adding Bootstrap through Angular CLI & NPM, & then creating & using the Datepicker Component
- By installing the Angular ngx Bootstrap & then using the Datepicker Component (Angular ng Bootstrap Datepicker Component)
Steps for Installing & Configuring the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Step 3: Install dependency
ng add @ng-bootstrap/ng-bootstrap
npm install --save angular-bootstrap-datetimepicker bootstrap moment open-iconic
Step 4: Add CSS to ./src/styles.css
@import '~bootstrap/dist/css/bootstrap.min.css';
@import '~open-iconic/font/css/open-iconic-bootstrap.css';
Project Structure
It will look like the following:
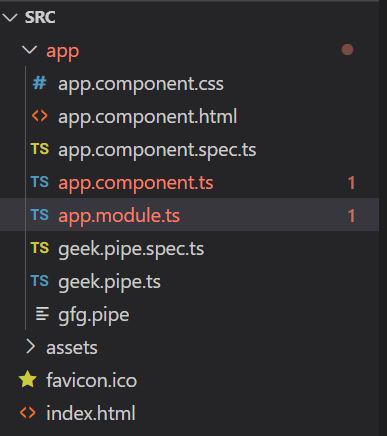
Example 1: In this example, we will create a simple Bootstrap Datepicker in Angular. We will be adding Bootstrap through Angular CLI & NPM, & then creating & using the Datepicker Component.
HTML
< h2 style = "color: green;" >GeeksforGeeks</ h2 >
< h2 >
What is the use of Bootstrap
Datepicker in Angular?
</ h2 >
< form class = "form-inline" >
< div class = "form-group" >
< div class = "input-group" >
< input class = "form-control"
placeholder = "yyyy-mm-dd"
name = "dp"
[(ngModel)]="model"
ngbDatepicker # d = "ngbDatepicker" >
< div class = "input-group-append" >
< button class = "btn btn-outline-secondary calendar"
(click)="d.toggle()"
type = "button" >
</ button >
</ div >
</ div >
</ div >
</ form >
|
Javascript
import { Component } from '@angular/core' ;
import { BrowserModule, DomSanitizer } from
'@angular/platform-browser'
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
model: any
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { FormsModule, ReactiveFormsModule }
from '@angular/forms' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { BrowserAnimationsModule }
from '@angular/platform-browser/animations' ;
import { AppComponent }
from './app.component' ;
import { NgbModule }
from '@ng-bootstrap/ng-bootstrap' ;
import { DlDateTimeDateModule, DlDateTimePickerModule }
from 'angular-bootstrap-datetimepicker' ;
@NgModule({
bootstrap: [
AppComponent
],
declarations: [
AppComponent
],
imports: [
FormsModule,
BrowserModule,
BrowserAnimationsModule,
ReactiveFormsModule,
DlDateTimeDateModule,
DlDateTimePickerModule,
]
})
export class AppModule { }
|
Output:
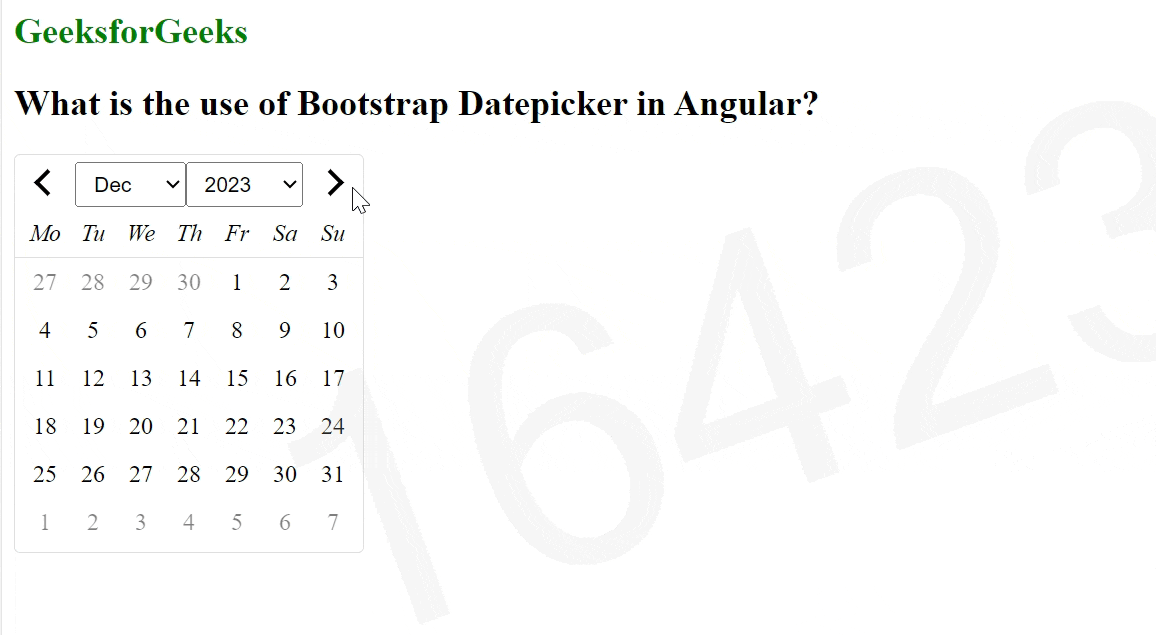
Example 2: In this example, we will use Bootstrap Datepicker in Angular by ngx Bootstrap & then use the Datepicker Component (Angular ng Bootstrap Datepicker Component). We will also display the selected date in the text.
HTML
< h2 style = "color: green;" >GeeksforGeeks</ h2 >
< h2 >
What is the use of Bootstrap
Datepicker in Angular?
</ h2 >
< ngb-datepicker #dp [(ngModel)]="model"
(navigate)="date = $event.next">
</ ngb-datepicker >
< hr />
< pre >Selected Date: {{ model | json }}</ pre >
|
Javascript
import { Component, inject }
from '@angular/core' ;
import { NgbCalendar, NgbDatepickerModule, NgbDateStruct }
from '@ng-bootstrap/ng-bootstrap' ;
import { FormsModule } from '@angular/forms' ;
import { JsonPipe } from '@angular/common' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ],
imports: [NgbDatepickerModule, FormsModule, JsonPipe],
standalone: true ,
})
export class AppComponent {
model: NgbDateStruct;
date: { year: number; month: number };
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { FormsModule, ReactiveFormsModule }
from '@angular/forms' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { BrowserAnimationsModule }
from '@angular/platform-browser/animations' ;
import { AppComponent }
from './app.component' ;
import { NgbModule }
from '@ng-bootstrap/ng-bootstrap' ;
@NgModule({
bootstrap: [
AppComponent
],
declarations: [
AppComponent
],
imports: [
FormsModule,
BrowserModule,
BrowserAnimationsModule,
ReactiveFormsModule,
NgbModule
]
})
export class AppModule { }
|
Output:
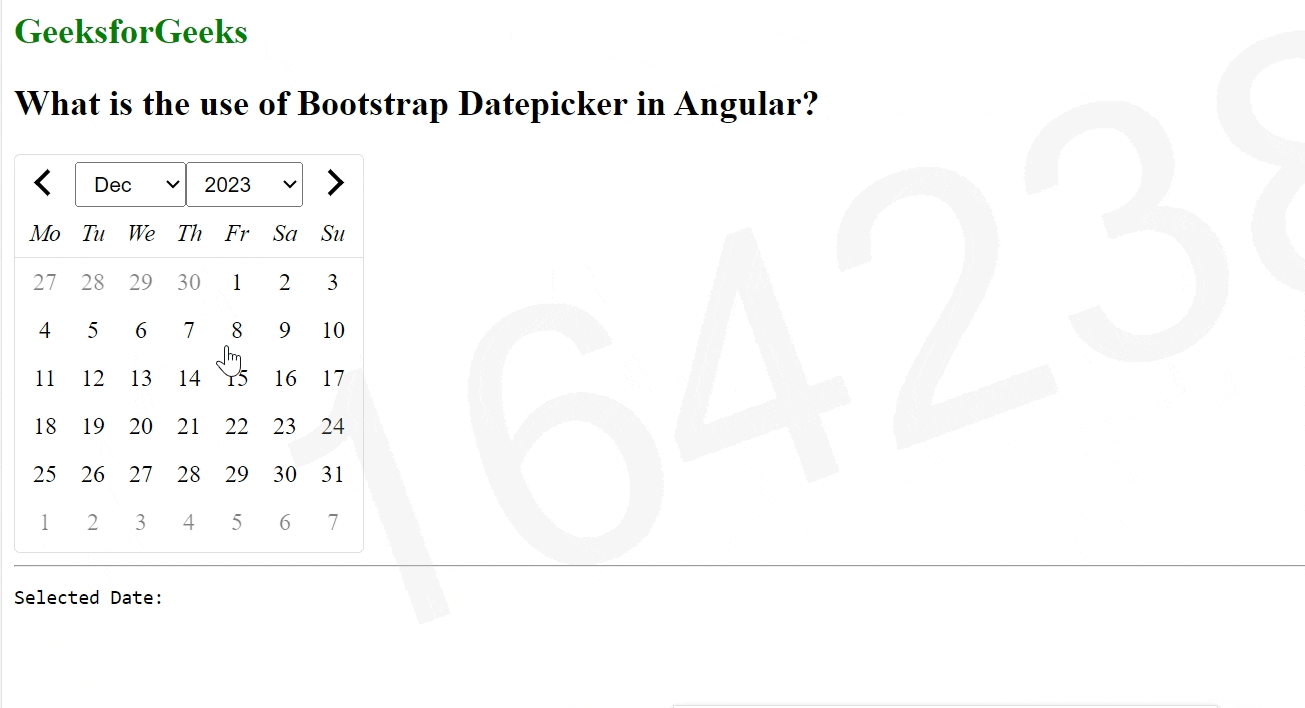
Share your thoughts in the comments
Please Login to comment...