What is the Lifecycle of an AngularJS Controller ?
Last Updated :
12 Jan, 2024
The Lifecycle defines the journey of the application, which it goes through, right from creation to destruction. The lifecycle of AngularJS determines how the data interacts with AngularJS. Understanding the lifecycle enables the developer to more efficiently develop applications with greater code reusability and maintainability. During the lifecycle, all these components are monitored and governed by AngularJS itself. It allows the developers to maintain and update the components and code, while it goes through the creation to destruction phase.
The lifecycle of an AngularJS controller refers to the sequence of events that occur from the creation to the destruction of the controller. AngularJS provides a set of predefined methods, often referred to as “lifecycle hooks,” that developers can use to interact with different phases of the controller’s existence.
Lifecycle of an AngularJS Controller
The following is the lifecycle of the  AngularJS Controller through which the Angular Applications are compiled:
Initialization Phase
In the initialization phase, you can set up initial data, define functions, and perform any necessary setup tasks. The $scope.init function is invoked to initialize variables, ensuring that the controller starts with the desired state.
Example:
HTML
<!DOCTYPE html>
< html lang = "en" ng-app = "app" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >
Lifecycle of an AngularJS Controller
</ title >
< script src =
</ script >
</ head >
< body ng-controller = "MyController" >
< h2 style = "color: green;" >
GeeksforGeeks
</ h2 >
< h2 >
What is the lifecycle of an AngularJS Controller?
</ h2 >
< script >
angular.module('app', [])
.controller('MyController', ['$scope', function ($scope) {
// Initialization and Configuration Phase
console.log('Initialization and Configuration Phase');
// Controller logic goes here
$scope.message = 'Hello, Geek!';
}]);
</ script >
</ body >
</ html >
|
Output
Â

Compilation and Binding Phase
In the Compilation and Binding phase, the controller establishes data binding with the view. In this example, the $scope.message variable is bound to the view, and a $watch expression is used to monitor changes to the variable. AngularJS compiles the HTML template and associates it with the controller. The ng-controller directive is used to link the controller (MyController) with the HTML element.
Example:
HTML
<!DOCTYPE html>
< html lang = "en" ng-app = "app" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >
Lifecycle of an AngularJS Controller
</ title >
< script src =
</ script >
</ head >
< body ng-controller = "MyController" >
< h2 style = "color: green;" >
GeeksforGeeks
</ h2 >
< h2 >
What is the lifecycle of an AngularJS Controller?
</ h2 >
< input type = "text" ng-model = "message" >
< p >{{ message }}</ p >
< script >
angular.module('app', [])
.controller('MyController', ['$scope', function ($scope) {
$scope.message = 'Hello, Geeks!';
}]);
</ script >
</ body >
</ html >
|
Output:
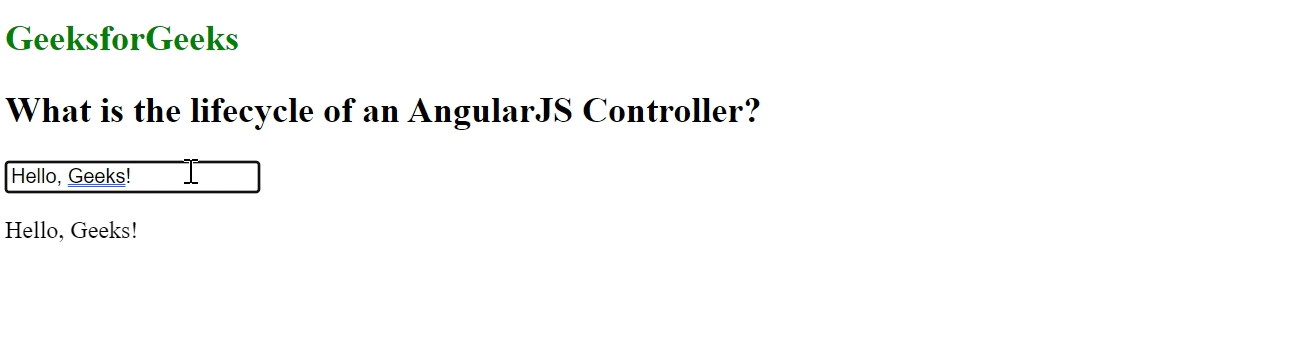
Runtime Phase
The runtime phase encompasses the time when the application is running. In this example, the $scope.changeMessage function represents a runtime action that changes the value of the message variable, triggering any associated data-binding updates.
Example:
HTML
<!DOCTYPE html>
< html lang = "en" ng-app = "app" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >
Lifecycle of an AngularJS Controller
</ title >
< script src =
</ script >
</ head >
< body ng-controller = "MyController" >
< h2 style = "color: green;" >
GeeksforGeeks
</ h2 >
< h2 >
What is the lifecycle of an AngularJS Controller?
</ h2 >
< h1 >{{ message }}</ h1 >
< script >
angular.module('app', [])
.controller('MyController', ['$scope', '$timeout',
function ($scope, $timeout) {
// Runtime Phase
console.log('Runtime Phase');
// Controller logic goes here
$scope.message = 'Hello, Geeks!';
// Simulating a runtime change
$timeout(function () {
$scope.message = 'Runtime Change!';
}, 5000);
}]);
</ script >
</ body >
</ html >
|
Output:
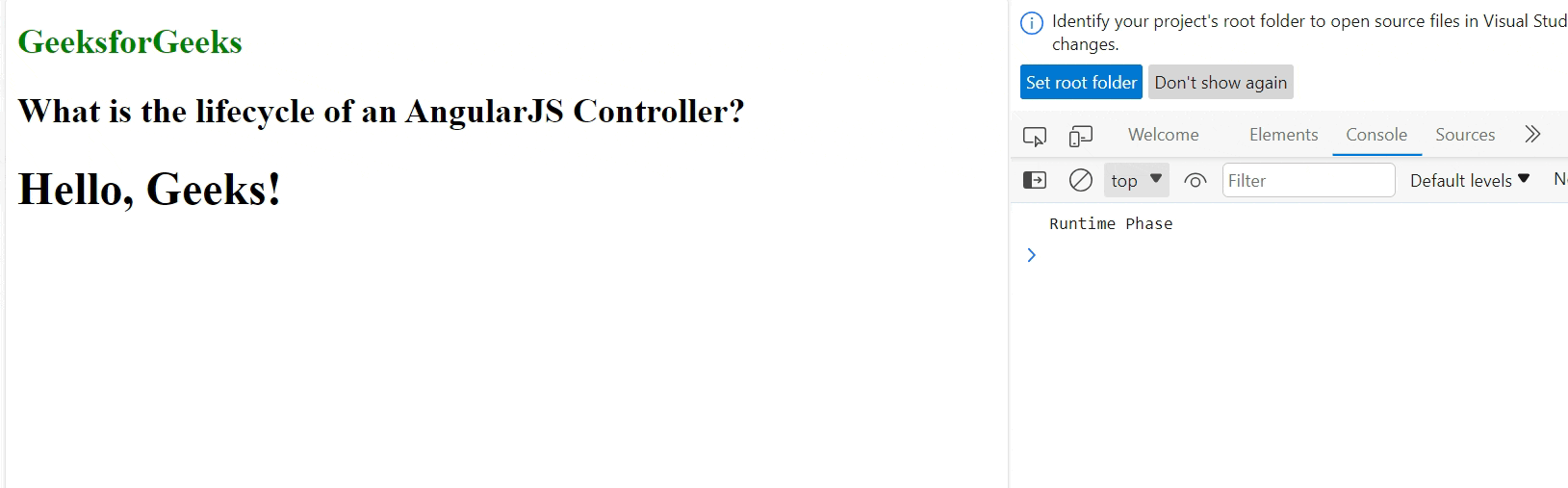
Destruction Phase
The destruction phase occurs when the controller is about to be removed. In this example, the $scope.$on(‘$destroy’) event handler is used to perform cleanup tasks or release resources before the controller is destroyed.
Example:
HTML
<!DOCTYPE html>
< html >
< head >
< title >
Lifecycle of an AngularJS Controller
</ title >
< script src =
</ script >
</ head >
< body ng-app = "myApp" >
< h2 style = "color: green;" >
GeeksforGeeks
</ h2 >
< h2 >
What is the lifecycle of an AngularJS Controller?
</ h2 >
< div ng-controller = 'test'
ng-if = "toggle" >
< button ng-click = 'dispatch()' >
EVENT
</ button >
</ div >
< button ng-click = 'toggle = !toggle' >
Click to add / remove an event
</ button >
< script >
var myApp = angular.module('myApp', []);
var startingDate = (new Date()).getTime();
myApp.controller('test', function ($scope) {
// Date controller was created
var controllerDate =
(new Date()).getTime() - startingDate;
console.log('Controller created at ', controllerDate);
// Listener that logs controller date
$scope.$on('dispatched', function () {
console.log('listener created at:', controllerDate);
});
// Function to dispatch event
$scope.dispatch = function () {
$scope.$emit('dispatched');
};
$scope.$on('$destroy', function () {
console.log('Controller Destroyed',
controllerDate, ' destroyed');
});
});
</ script >
</ body >
</ html >
|
Here, the $scope.$on(‘$destroy’) event handler is used to listen for the destruction event. When the controller is about to be destroyed, the associated callback function is executed, allowing you to perform cleanup operations.
Output:
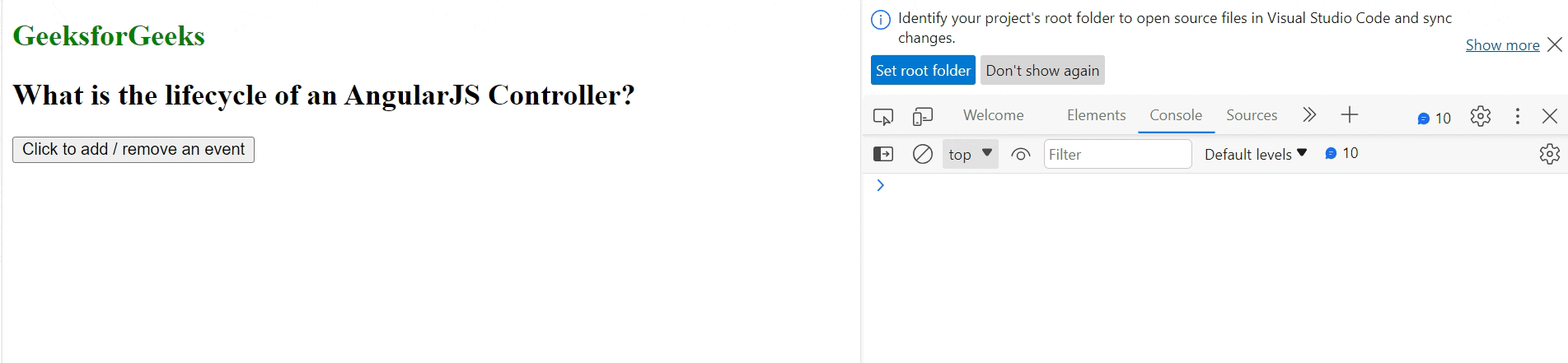
Note
- Controllers in AngularJS follow a similar lifecycle pattern for each instance, and the described phases are part of this general lifecycle.
- The examples provided are simplified and may not cover all use cases. Actual implementations depend on the specific requirements of your application.
Key Benefits and Use Cases
- Resource Management: Developers can use the destruction phase to release resources, such as event listeners or subscriptions, preventing memory leaks and improving overall application performance.
- Dynamic Updates: The binding and runtime phases enable developers to create dynamic and responsive user interfaces by updating the view based on changes in the model and handling user interactions.
- Initialization Logic: Initialization allows developers to set up the controller with the required initial state, reducing redundancy in code and ensuring a consistent starting point.
- Integration with External Services: Developers can use lifecycle hooks to integrate the controller with external services, such as data fetching or authentication, ensuring that these processes are initiated and managed at appropriate times.
- Custom Behavior: By leveraging the lifecycle hooks, developers can introduce custom behavior or functionality specific to the controller, tailoring its behavior to the needs of the application.
- Event Handling: The runtime phase is crucial for handling user events and interactions. Developers can respond to these events in real time, updating the controller’s state and the associated view.
- Optimizing Performance: Properly managing the lifecycle allows developers to optimize performance by efficiently handling resource allocation and deallocation, reducing unnecessary computations, and improving the overall responsiveness of the application.
Conclusion
Understanding the lifecycle of an AngularJS controller is crucial for effective development and resource management within the framework. Developers can leverage these lifecycle hooks to initialize, bind, and clean up resources as needed throughout the controller’s existence. The AngularJS controller lifecycle provides a structured way for developers to manage the various stages of a controller’s existence. By utilizing lifecycle hooks, developers can ensure proper initialization, effective data binding, responsive runtime behavior, and clean resource disposal, leading to well-structured and maintainable AngularJS applications.
Share your thoughts in the comments
Please Login to comment...