How can AngularJS controller inherit from another controller in the same Module ?
Last Updated :
29 Oct, 2023
In the AngularJS Framework, Controller Inheritance allows the controller to inherit the properties, methods, and other kinds of utilities from the other controller in the same module. It is nothing but the inheritance concept of OOP. So, in AngularJS, to perform the controller inheritance, we can use two different approaches, i.e., the $controller service and the Object Prototypes. In this article, we will cover both approaches with a demonstration of the approaches in terms of examples.
Steps for Configuring the AngularJS Applications
The below steps will be followed to configure the AngularJS Applications, to perform controller inheritance:
Step 1: Create a new folder for the project. We are using the VSCode IDE to execute the command in the integrated terminal of VSCode.
mkdir controller-inherit
cd controller-inherit
Step 2: Create the index.html file which will have the entire behavior of the application including the styling, AngularJS code, and structural HTML code.
We will explore the above approaches & understand them with the help of suitable examples.
In this approach, to perform the controller inheritance from another controller in the same module we are using the $controller service. Here, we have defined the BaseController using the app. controller. This controller has the methods and the properties that we want to inherit from another controller. Then we have defined one more controller as ChildController. This controller is also defined as app.controller. This uses the $controller service which mainly inherits the properties and the methods from the BaseController.
Syntax
$controller('BaseController', { $scope: $scope });
Here, the instance of the BaseController is made and it extends the child controller’s scope ($scope) with all the properties and the methods defined in the BaseController.
Example: Below is an example that demonstrates How can an AngularJS controller inherit from another controller in the same module using $controller Service.
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< title >
Controller Inheritance
</ title >
< style >
.container {
text-align: center;
}
.box {
border: 1px solid #ccc;
padding: 10px;
margin: 10px;
background-color: #f5f5f5;
}
h1 {
color: green;
}
</ style >
< script src =
</ script >
</ head >
< body ng-controller = "BaseController" >
< div class = "container" >
< h1 >GeeksforGeeks</ h1 >
< h3 >
Approach 1: Using $controller service
</ h3 >
< div class = "box" >
< h2 >{{ title }}</ h2 >
< button ng-click = "baseButtonClick()" >
Base Controller Button
</ button >
</ div >
< div class = "box"
ng-controller = "ChildController" >
< h2 >{{ title }}</ h2 >
< button ng-click = "baseButtonClick()" >
Inherited Controller Button
</ button >
< button ng-click = "childButtonClick()" >
Child Controller Button
</ button >
< p >
Inheritance allows child
controllers to inherit properties
and methods from their parent controllers.
</ p >
</ div >
</ div >
< script >
var app = angular.module('myApp', []);
// Base Controller
app.controller('BaseController', function ($scope) {
// Properties and methods
// for the base controller
$scope.title = 'Base Controller';
$scope.baseButtonClick = function () {
alert('Base controller button clicked');
};
});
// Child Controller inherits from BaseController
app.controller('ChildController',
function ($scope, $controller) {
// Inherit from BaseController
$controller('BaseController', { $scope: $scope });
// Additional properties and
// methods for ChildController
$scope.title = 'Inherited Controller';
$scope.childButtonClick = function () {
alert('Child controller button clicked');
};
});
</ script >
</ body >
</ html >
|
Output:
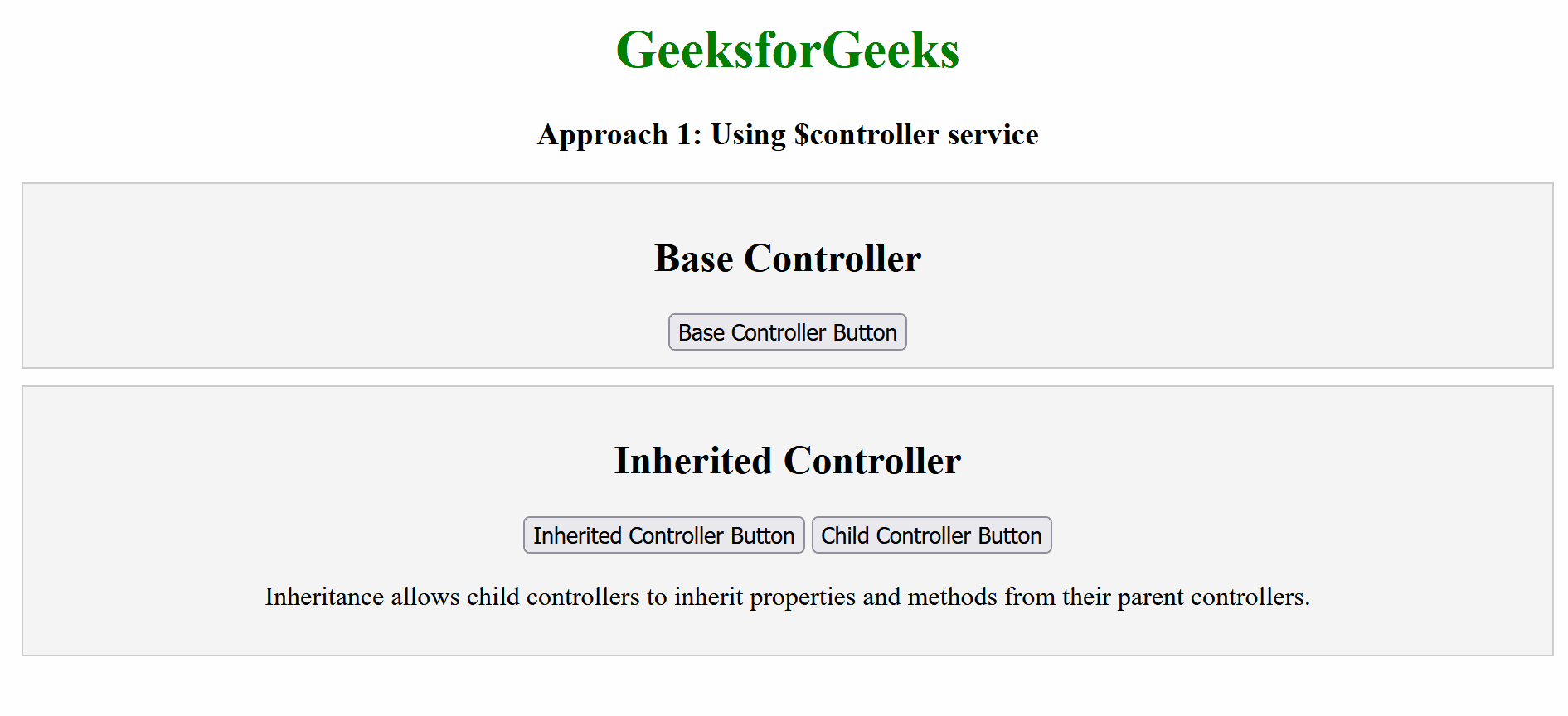
In this approach, we are using the Object Prototypes. Here we have defined the Object Prototypes, these are nothing but her objects that are been associated with the prototype object. This prototype object can be seen as the “template” in which the object can inherit the properties and the methods. Here, we have defined the Base controller as BaseController, which has the specific properties and the methods, and then we have defined the ChildController which inherits the properties from the BaseController by triggering it within its definition using the ‘BaseController($scope)’. This mainly extends the child controller’s cope with the properties and the methods that are defined in the BaseController.
Example: Below is an example that showcases How can an AngularJS controller inherit from another controller in the same module using Object Prototypes.
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< title >
Controller Inheritance
</ title >
< script src =
</ script >
< style >
body {
font-family: Arial, sans-serif;
background-color: #f3f3f3;
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.container {
text-align: center;
max-width: 600px;
padding: 20px;
background-color: #fff;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.2);
}
.box {
border: 1px solid #ccc;
padding: 10px;
margin: 10px;
}
.form-group {
text-align: left;
margin-bottom: 10px;
}
</ style >
</ head >
< body ng-controller = "ChildController" >
< div class = "container" >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >
Approach 2: Using Object Prototypes
</ h3 >
< div class = "box" >
< h2 >
User Information (Base Controller)
</ h2 >
< div class = "form-group" >
< label >
< strong >Name:</ strong >
</ label >
< p >{{ user.name }}</ p >
</ div >
< div class = "form-group" >
< label >
< strong >Email:</ strong >
</ label >
< p >{{ user.email }}</ p >
</ div >
</ div >
< div class = "box" >
< h2 >Additional Information</ h2 >
< div class = "form-group" >
< label >
< strong >Age:</ strong >
</ label >
< p >{{ user.age }}</ p >
</ div >
< div class = "form-group" >
< label >
< strong >Location:</ strong >
</ label >
< p >{{ user.location }}</ p >
</ div >
</ div >
</ div >
< div class = "container" >
< div class = "box" >
< h2 >Edit User Info (Child Controller)</ h2 >
< form ng-submit = "updateUserInfo()" >
< div class = "form-group" >
< label >Name:</ label >
< input type = "text"
class = "form-control"
ng-model = "user.name" >
</ div >
< div class = "form-group" >
< label >Email:</ label >
< input type = "email"
class = "form-control"
ng-model = "user.email" >
</ div >
< div class = "form-group" >
< label >Age:</ label >
< input type = "number"
class = "form-control"
ng-model = "user.age" >
</ div >
< div class = "form-group" >
< label >Location:</ label >
< input type = "text"
class = "form-control"
ng-model = "user.location" >
</ div >
< button type = "submit"
class = "btn btn-primary" >
Update
</ button >
</ form >
</ div >
</ div >
< script >
var app = angular.module('myApp', []);
// Base Controller
function BaseController($scope) {
$scope.user = {
name: 'GFG1',
email: 'gfg@gmail.com',
};
}
// Child Controller
function ChildController($scope) {
BaseController($scope);
$scope.user.age = 30;
$scope.user.location = 'Noida';
$scope.updateUserInfo = function () {
alert('User info updated successfully!');
};
}
app.controller('BaseController', BaseController);
app.controller('ChildController', ChildController);
</ script >
</ body >
</ html >
|
Output:
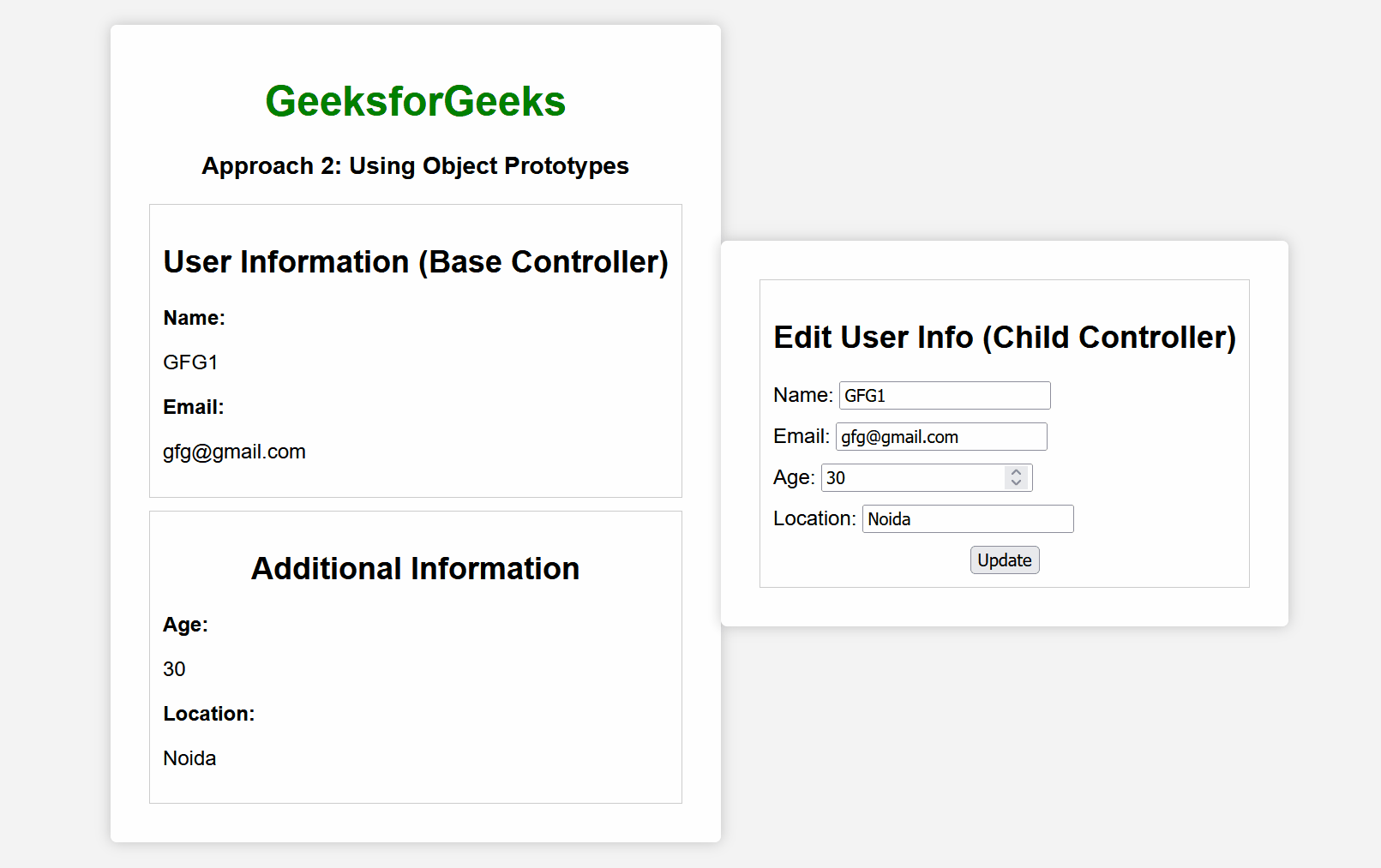
Share your thoughts in the comments
Please Login to comment...