What is the difference between export.create and router.post?
Last Updated :
19 Dec, 2023
export.create
and router.post
are used in the context of backend frameworks like Express JS. They both are used for handling HTTP requests and operate at different levels within the framework. In this article we will learn about export.create and router.post and see the key differences between them.
Prerequisites
Steps to Create Express JS Application
Step 1: In the first step, we will create the new folder by using the below command in the VScode terminal.
mkdir folder-name
cd folder-name
Step 2: After creating the folder, initialize the NPM using the below command. Using this the package.json file will be created.
npm init -y
Step 3: Now, we will install the express dependency for our project using the below command.
npm i express
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2"
}
export.create
In Web Programming the export.create is used for making a function, variable, or module available for use in other parts of the program. Using this, we can properly modularize the code by exporting a specific function that is responsible for creating or initializing the objects.
Syntax:
exports.create = (data) => {
// data or perform some action
};
Project Structure:
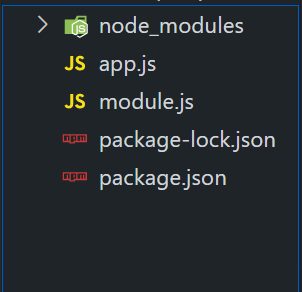
Example: In the below example, we have created the module.js module that exports the create function and in the app.js we are importing this module and using the create function to print the square of the given number.
Javascript
exports.create = (n) => {
const res = n * n;
return res;
};
|
Javascript
const { create } = require( './module' );
const num = 5;
const res = create(num);
console.log(`The square of ${num} is: ${res}`)
|
Output:
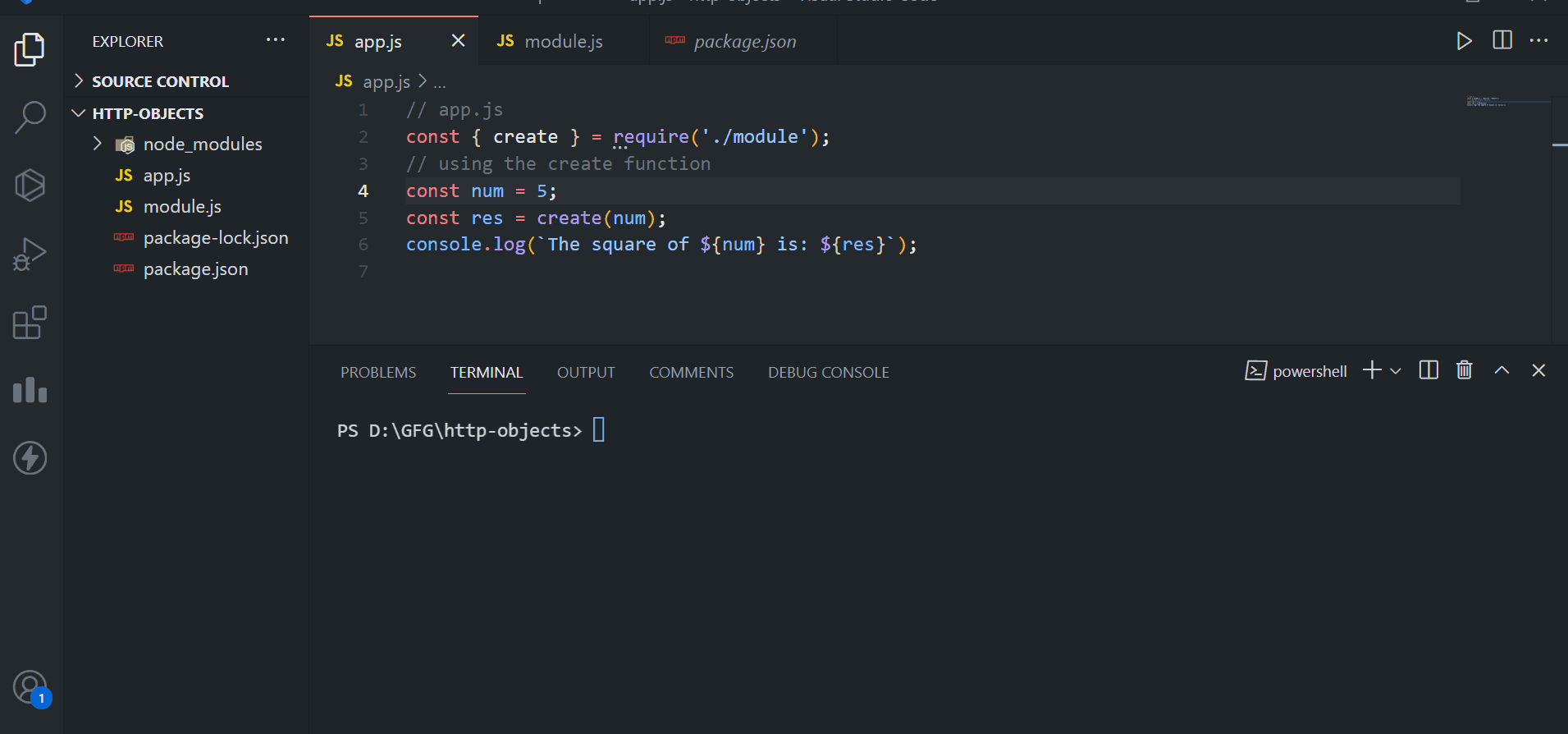
router.post
The router.post is the method that is used to define the route for handling the HTTP POST request on the specified path. Here the post method is linked with creating or submitting the data to the server and it also takes the callback function as its second argument which specifies the logic to be executed when the POST request is done on the route.
Syntax:
router.post('/example', (req, res) => {
// handle the POST request
res.send('Handling POST request');
});
Project Structure:
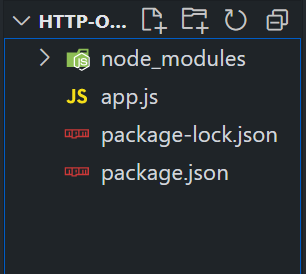
Example: In the below example, we have set up a simple Express server that listens for the POST request on the /api/example route and responds with he JSON message when the request is been handled.
Javascript
const express = require( 'express' );
const app = express();
const port = 3000;
app.use(express.json());
app.post( '/api/example' , (req, res) => {
const data = req.body;
res.json({ message: 'Hey Geek! POST request received' , data });
});
app.listen(port, () => {
console.log(`Server is listening on port ${port}`);
});
|
Output:
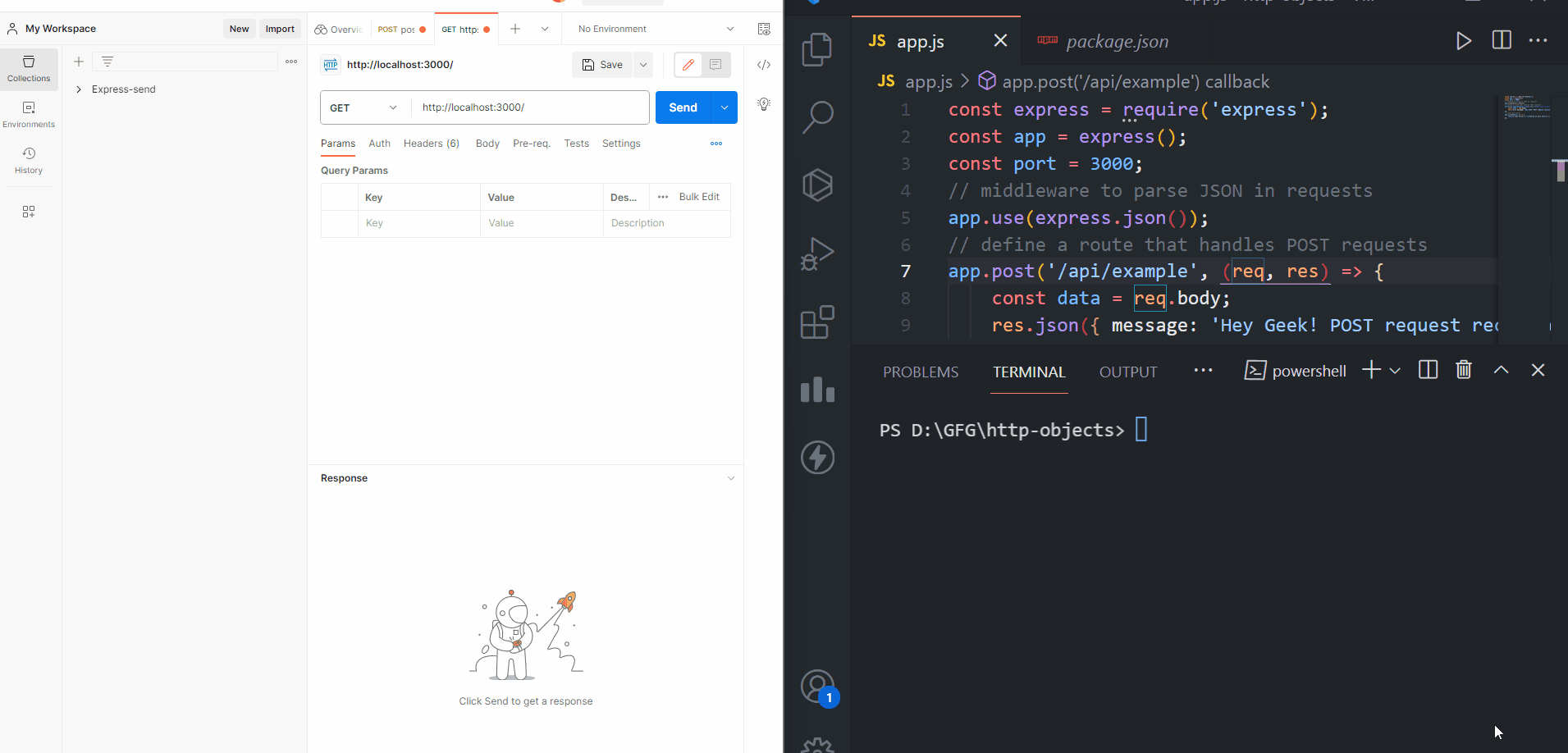
Difference between export.create and router.post
Use
|
export.create is used to export a function or object from a module.
|
router.post is used to handle HTTP POST requests in the Express application.
|
Syntax
|
exports.create = (req, res) => { /* logic */ };
|
app.post('/api/example', (req, res) => { /* logic */ });
|
Functionality
|
This depends on the logic which is inside the exported function.
|
This defines the; logic for handling POST requests.
|
Request Parameter
|
Depends on the function’s logic and input parameters.
|
Accesses the request parameters like req.body and req.params.
|
Conclusion:
In conclusion, export.Create is a custom feature of exporting modules, on the other hand router.Post is an inbuilt feature in Express used for defining routes that deals with HTTP POST requests. They serve different purposes and are used in different contexts.
Share your thoughts in the comments
Please Login to comment...