What is React Select ?
Last Updated :
30 Nov, 2023
React Select is a drop-down select component that has multiple features like multi select and autocomplete. React select also offers custom styling and fixed selection in it. In this article, we are going to learn and implement React Select.
Prerequisites
Installation
React Select is a module of React JS that is used to implement a drop-down that has multiple features like multiselect and autocomplete. To install this React Select component, we need to use a given command.
npm i react-select
Syntax for Importing:
import Select from 'react-select'
Animated Components
The React Select module provides animation and other features that help improve the user interface. There is another module called makeAnimated from the same library that is used to provide animation to the components used in Select.
Syntax:
import makeAnimated from 'react-select/animated';
Custom Styles
In React Select components, we can also apply CSS stylings other than default styling. For that styling, we have to create a JSON object of styling and pass that object to Select as style props.
Syntax:
<Select styles={styleObject}/>
Async
Async is mostly used for making HTTP requests here if we need to display options from a server, so we have a module AsyncSelect that will take loadOptions as props when we have to pass the function to load options.
Syntax:
import AsyncSelect from 'react-select'
<AsyncSelect loadOptions={function}/>
Creatable
Creatable is a component of React Select that is used to create new components while selecting options. As usual, first we need to import it, then we can use it in our code.
Syntax:
import CreatableSelect from 'react-select/creatable';
<CreatableSelect isClearable options={colourOptions} />
Fixed Options
In React Select, we can set some options as fixed means by default; in the case of multiselect, they should be selected.
Example : Implementation of React Select.
Javascript
import React from 'react' ;
import Select from 'react-select' ;
import makeAnimated from 'react-select/animated' ;
import "./App.css"
const animatedComponents = makeAnimated();
export default function AnimatedMulti() {
const option = [
{ label: "Web Development" , value: "Web Development" },
{ label: "Data Science" , value: "Data Science" },
{ label: "Machine Learning" , value: "Machine Learning" },
{ label: "Game Development" , value: "Game Development" },
{ label: "Design" , value: "Design" },
{ label: "Data Structure" , value: "Data Structure" },
]
return (
<div className= 'App' >
<h2>GeeksforGeeks</h2>
<Select closeMenuOnSelect={ false }
components={animatedComponents}
isMulti
defaultValue={[option[2], option[3]]}
options={option}
className= 'select' />
</div>
);
}
|
CSS
.App {
padding : 10px ;
width : 30 vw;
margin : auto ;
display : flex;
flex- direction : column;
align-items: center ;
color : green ;
font-size : 24px ;
}
.select {
min-width : 20 vw;
}
|
Output:
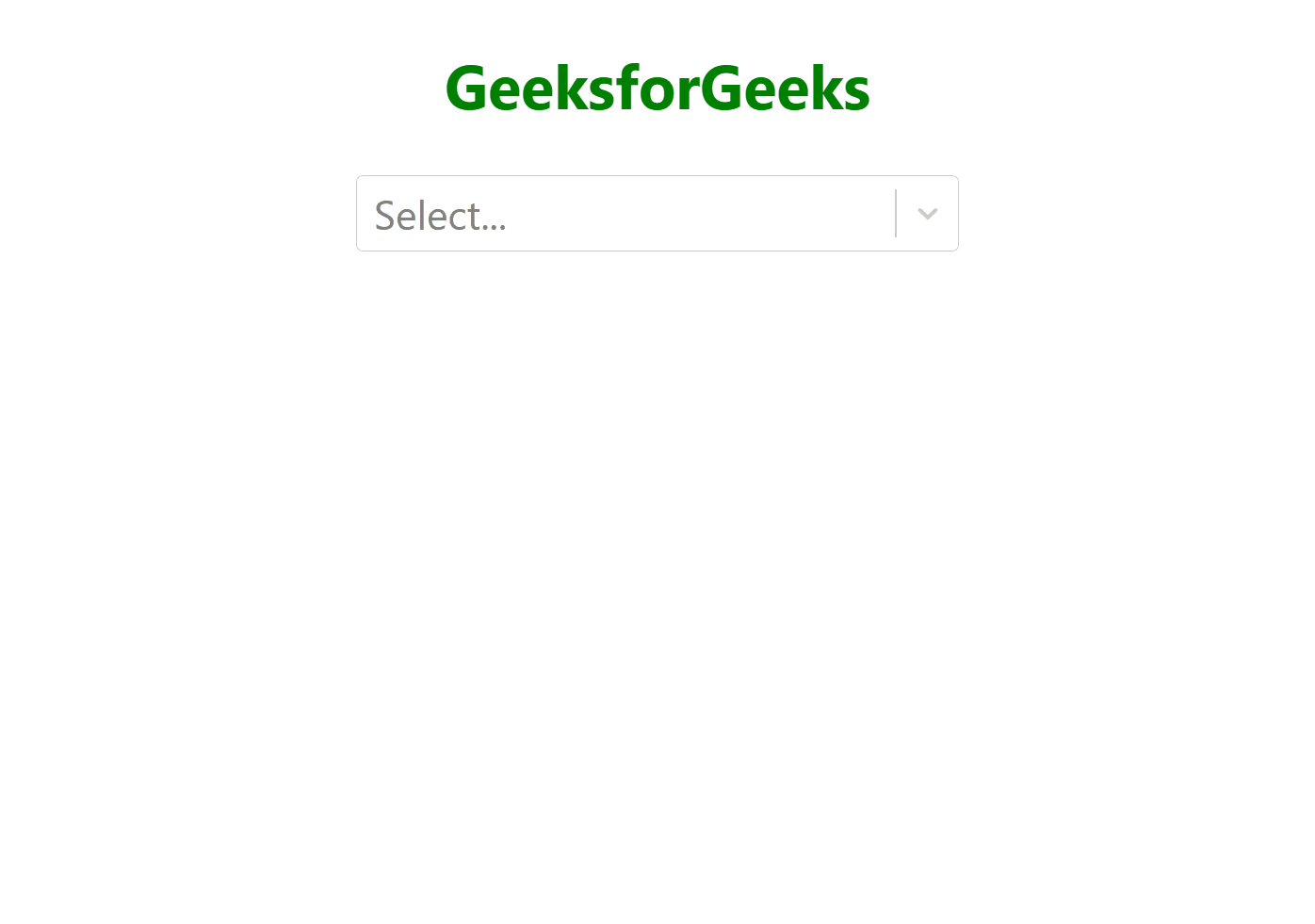
Share your thoughts in the comments
Please Login to comment...