How to pass input to a custom directive in Angular JS?
Last Updated :
15 Mar, 2024
In AngularJS, passing input to a custom directive allows the customization of components within an application. Directives can receive input via attributes, expressions, or controller functions, allowing for dynamic behavior and data binding.
In this article, we will explore two different approaches to pass input to a custom directive.
Steps to Configure the AngularJS Applications
The below steps will be followed to configure the AngularJS Applications:
Step 1: Create a new folder for the project. We are using the VSCode IDE to execute the command in the integrated terminal of VSCode.
mkdir custom-directive
cd custom-directive
Step 2: Create the index.html file in the newly created folder, we will have all our logic and styling code in this file.
Approach 1: Using Attribute Binding
In this approach, we are using attribute binding to pass input to a custom directive in AngularJS. The directive is defined with restrict: ‘A’, and the input value is passed through the myDirective attribute. Within the directive’s link function, we watch for changes in the myDirective value and update the element’s text.
Syntax:
<div my-directive="name"></div>
Example: The below example uses Attribute Binding to pass input to a custom directive in AngularJS.
HTML
<!-- index.html -->
<!DOCTYPE html>
<head>
<title>Approach 1: Attribute Binding</title>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.8.2/angular.min.js">
</script>
</head>
<body ng-app="myApp" ng-controller="myCtrl">
<h1 style="color: green;">GeeksforGeeks</h1>
<h3>Approach 1: Using Attribute Binding</h3>
<input type="text" ng-model="name" placeholder="Enter your name">
<div my-directive="name"></div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function ($scope) {
$scope.name = "";
});
app.directive('myDirective', function () {
return {
restrict: 'A',
scope: {
myDirective: '='
},
link: function (scope, element, attrs) {
scope.$watch('myDirective',function(newVal,oldVal){
if (newVal !== oldVal) {
element.text("Hello, " + newVal);
}
});
}
};
});
</script>
</body>
</html>
Output:
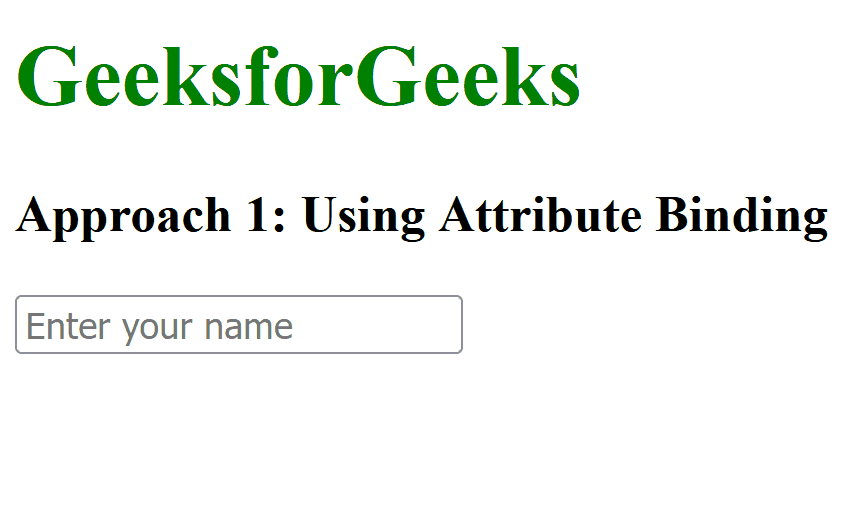
Approach 2: Using Services
In this approach, we are using an AngularJS service to perform communication between the controller and the directive. The service (inputService) stores the user input and provides methods to manipulate it. The controller updates the service with the user input, and the directive listens for changes in the service’s data and updates the DOM.
Syntax:
app.service('serviceName', function() {
 // service code
  this.someMethod = function() {
    // Method implementation
  };
  this.someProperty = 'value';
});
Example: The below example uses Services to pass input to a custom directive in AngularJS.
HTML
<!-- index.html -->
<!DOCTYPE html>
<head>
<title>Approach 2: Using Services</title>
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.8.2/angular.min.js">
</script>
</head>
<body ng-app="myApp" ng-controller="myCtrl">
<h1 style="color: green;">GeeksforGeeks</h1>
<h3>Approach 2: Using Services</h3>
<input type="text" ng-model="name" placeholder="Enter your name">
<button ng-click="approachFn()">Click Me</button>
<div my-directive></div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function ($scope, inputService) {
$scope.name = "";
$scope.approachFn = function () {
inputService.approachFn($scope.name);
};
});
app.service('inputService', function () {
this.uInput = "";
this.approachFn = function (name) {
this.uInput = "Hello, " + name;
};
this.getFn = function () {
return this.uInput;
};
});
app.directive('myDirective', function (inputService) {
return {
restrict: 'A',
link: function (scope, element, attrs) {
scope.$watch(function () {
return inputService.getFn();
}, function (newVal, oldVal) {
if (newVal !== oldVal) {
element.text(newVal);
}
});
}
};
});
</script>
</body>
</html>
Output:
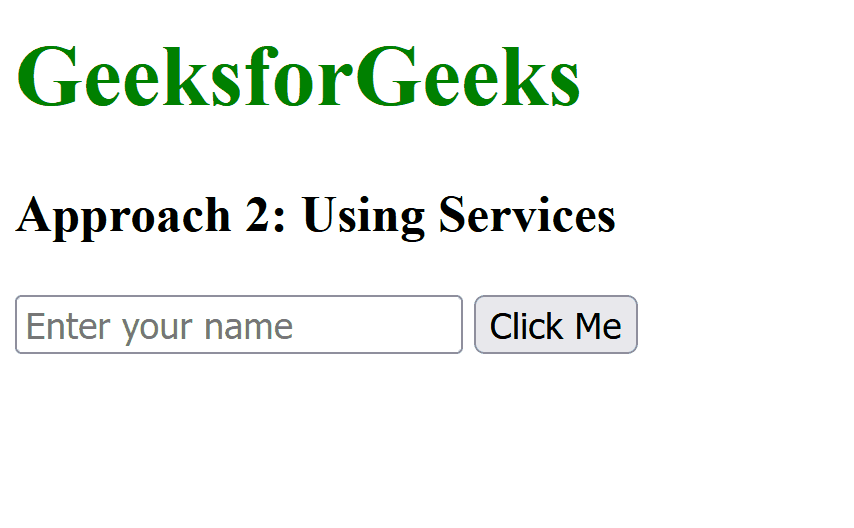
Share your thoughts in the comments
Please Login to comment...