Vue.js Slots
Last Updated :
27 Dec, 2023
Vue.js slots are one of the powerful features in the slot system, allowing the creation of flexible and reusable components. Slots act as placeholders within a component, enabling dynamic content insertion from the parent component. Vue.js slots are a powerful feature that brings flexibility and reusability to component-based development.
Slot Concepts
- Slot Content and Outlet: Slots consist of a slot content (provided by the parent) and a slot outlet (specified in the child component).
- Render Scope: The content passed through a slot can access the scope of the child component where it is rendered.
- Fallback Content: Slots can have fallback content, which is displayed when the parent component doesn’t provide any content.
- Named Slots: Slots can be named, allowing the parent to inject content into specific placeholders within the child component.
- Dynamic Slot Names: Slot names can be dynamic, enabling conditional rendering of content based on the parent’s data.
- Scoped Slots: Scoped slots provide a method for the child component to provide data to the parent, allowing for more dynamic content rendering.
Let’s create a project in Vue.js
Step 1: Install Vue CLI
>> npm install -g @vue/cli
Step 2: Create a New Vue Project [by this command Create a new Vue project]
>> vue create slotwork
Step 2: Then Navigate into the project directory by the above command
>> cd slotwork
Project Structure:
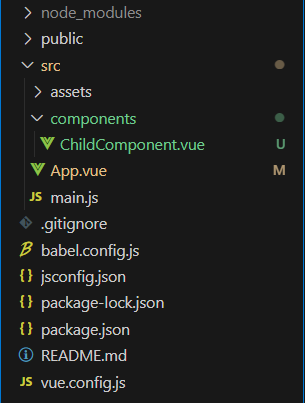
Project Structure
package.json:
"dependencies": {
"core-js": "^3.8.3",
"vue": "^3.2.13"
},
"devDependencies": {
"@babel/core": "^7.12.16",
"@babel/eslint-parser": "^7.12.16",
"@vue/cli-plugin-babel": "~5.0.0",
"@vue/cli-plugin-eslint": "~5.0.0",
"@vue/cli-service": "~5.0.0",
"eslint": "^7.32.0",
"eslint-plugin-vue": "^8.0.3"
}
Example 1: In this example, we will see the implementation of the slots.
Javascript
src/App.
<template>
<div>
<h1>Parent Component</h1>
<child-component>
<p>This content goes into the
default slot in the Child component.</p>
</child-component>
</div>
</template>
<script>
import ChildComponent from './components/ChildComponent.vue'
export default {
name: 'App' ,
components: {
ChildComponent
}
}
</script>
<style>
#app {
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
|
Javascript
/components/ChildComponent.vue
<template>
<div>
<slot></slot>
</div>
</template>
<script>
export default {
name: 'ChildComponent' ,
props: {
msg: String
}
}
</script>
|
The output will be HTML rendered by Vue.js. In the the example, the content passed into the default slot will be displayed.
Steps to Run the Application:
>> npm run serve
Output: Open the browser and navigate to http://localhost:3000
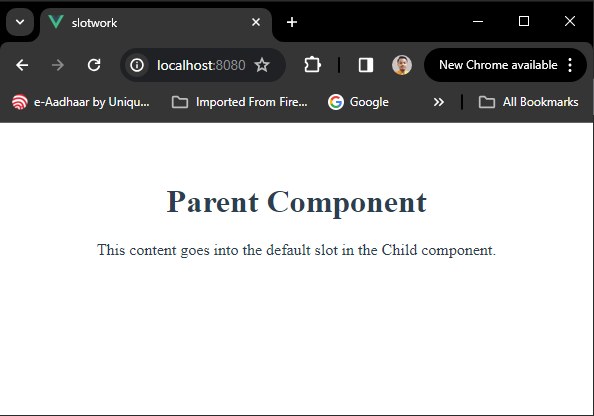
Output of example1
Example 2: This example shows the use of Named Slots
Javascript
<template>
<div>
<h1>Parent Component</h1>
<child-component>
<template v-slot:header>
<h1>This is the header slot</h1>
</template>
<p>This content goes into the default slot.</p>
<template v-slot:footer>
<p>This is the footer slot</p>
</template>
</child-component>
</div>
</template>
<script>
import ChildComponent from './components/ChildComponent.vue'
export default {
name: 'App' ,
components: {
ChildComponent
}
}
</script>
<style>
#app {
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
|
Javascript
<template>
<div>
<slot name= "header" ></slot>
<div>
<slot></slot>
</div>
<slot name= "footer" ></slot>
</div>
</template>
<script>
export default {
name: 'ChildComponent' ,
props: {
msg: String
}
}
</script>
|
The output will be HTML rendered by Vue.js. In the the example, the content passed into the default slot will be displayed.
Steps to Run the Application:
>> npm run serve
Output: This output will be HTML rendered by Vue.js. In the example, the header, default content, and footer will be rendered in their respective slots. Open the browser and navigate to http://localhost:3000
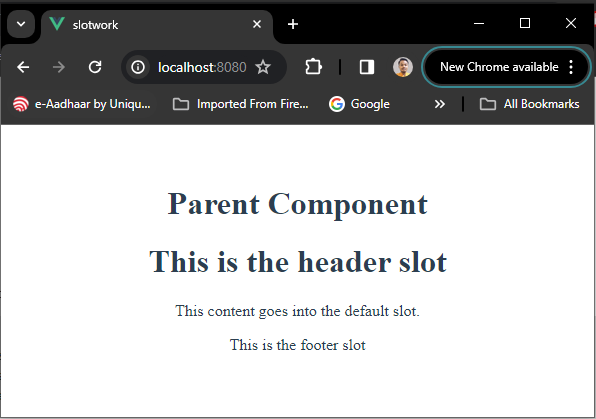
Output Example2
Conclusion: Understanding Vue.js slots gives developers with a powerful method for creating flexible and reusable components, improves the modularity of Vue.js applications. Vue.js slots provides a simple and efficient approach for component composition. By knowing the concepts of slot content, named slots, and scoped slots, developers can enhance the flexibility and maintainability of their Vue.js applications. Their use, however, should be carefully examined, evaluating the benefits against the possible negatives, especially in smaller components or for developers new to Vue.js.
Share your thoughts in the comments
Please Login to comment...