Vue.js Dynamic List Transitions
Last Updated :
01 Aug, 2023
Dynamic List Transition
Dynamic List Transitions defines as the animated transitions that occur when the elements are added, remove, or rearranged in a list. This process wraps the list in transition components by adding CSS for transition and adding animations to the transition components.
Implementing Dynamic List Transition
- Adding Transitions: The programmer can wrap the list component with the
<transition-group>
element provided by Vue.js. This element acts as a container for the list and transition which applies to list items.
- Defining Transition Classes: It includes some set of predefined CSS classes which can be used to define different levels of the transition. These classes contain
v-enter
, v-enter-active
, v-leave
, v-leave-active
, etc. programmer can define CSS rules for these classes to create the desired transition.
- Applying Key Attribute: Each item in a list should have a unique
:key
attribute. this key attribute in Vue.js are helping to identify items and apply appropriate transition effects when they are added, removed, or rearranged.
- Transition Modes and Customization: Vue.js are providing different transition modes, like “in-out” and “out-in”, which is determine the order of animation when items are added or removed simultaneously. Vue.js serves various transition properties and provides hooks that can be used to customize the timing, behavior, and duration of the transitions.
Syntax :
<transition-group
name="list" tag="ul"
enter-active-class="fade-enter-active"
leave-active-class="fade-leave-active"
appear
appear-class="fade-appear"
appear-active-class="fade-appear-active"
>
<li
v-for="(item, index) in items"
:key="item.id"
v-bind="item"
>
{{ item.text }}
</li>
</transition-group>
To Install Vue.js in your system, follow to given steps :
Step 1: To create a new Vue.js project execute the following command.
npm init vue@latest
Project Structure
The project Structure will look like this after the installation.
.png)
Here are some examples of Dynamic List Transitions. So you can try these examples in your Vue.js project. Copy the Code and paste it as it is in App.vue file.
Example 1: In this example, we created a list by using dynamic List Transitions. And put all the code in App.vue file for the execution.
HTML
< template >
< div >
< h2 >My List</ h2 >
< button @ click = "addItem" >Add Item</ button >
< transition-group name = "list" tag = "ul" >
< li v-for = "item in items" :key = "item.id" >
{{ item.name }}
</ li >
</ transition-group >
</ div >
</ template >
< script >
export default {
data() {
return {
items: [
{ id: 1, name: "Item 1" },
{ id: 2, name: "Item 2" },
{ id: 3, name: "Item 3" },
{ id: 4, name: "Item 4" },
],
nextItemId: 5,
};
},
methods: {
addItem() {
this.items.push({
id: this.nextItemId,
name: "New Item",
});
this.nextItemId++;
},
},
};
</ script >
< style >
.list-enter-active,
.list-leave-active {
transform: scale(0);
transition: opacity 0.5s, transform 0.5s;
}
.list-enter,
.list-leave-to {
transform: scale(1);
opacity: 0;
transform: translateY(30px);
}
</ style >
|
Output: In this example, the list item was added to the list in non-ordered. It is a simple basic list.
.gif)
Example 2: In this example, when the user clicks the button the item was printed on the window display in numeric order.
HTML
< template >
< div >
< h1 >GeeksforGeeks</ h1 >
< transition-group name = "list" tag = "ul" >
< li
v-for = "item in items"
:key = "item.id"
:class = "{ active: item.active }"
>
{{ item.name }}
</ li >
</ transition-group >
< button @ click = "addItem" :disabled = "addingItem" >
Add Item
</ button >
</ div >
</ template >
< script >
export default {
data() {
return {
items: [],
addingItem: false,
};
},
methods: {
addItem() {
const newItem = {
id: this.items.length + 1,
name: "Item " + (this.items.length + 1),
active: false,
};
this.items.push(newItem);
this.addingItem = true;
// Triggering the transition
this.$nextTick(() => {
newItem.active = true;
setTimeout(() => {
this.addingItem = false;
}, 500);
});
},
},
};
</ script >
< style scoped>
h2 {
color: green;
font-size: 3rem;
}
body {
margin: 5rem;
}
.list {
list-style-type: none;
padding: 0;
}
.list li {
opacity: 0;
transform: scale(0.5);
transition: opacity 0.5s, transform 0.5s;
}
.list li.active {
opacity: 1;
transform: scale(1);
}
</ style >
|
Output:
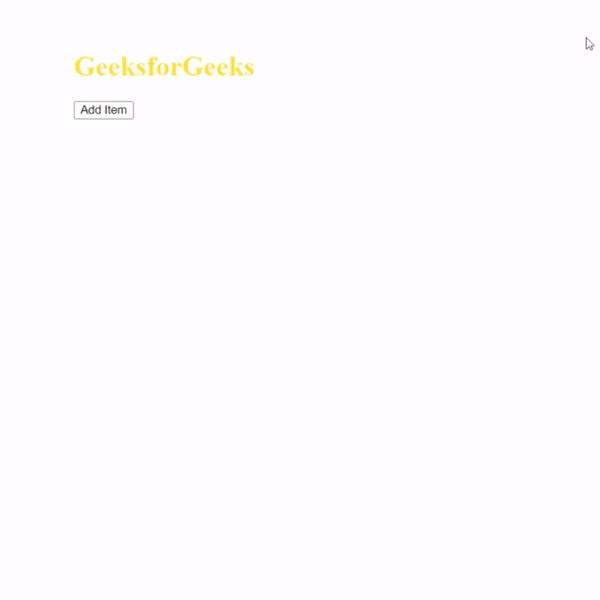
Share your thoughts in the comments
Please Login to comment...