Vue.js Reusable List Transitions
Last Updated :
24 Apr, 2022
Vue.js is a progressive javascript framework for developing web user interfaces. It is a versatile framework providing high speed and performance. We can create Single Page Applications as well as Full Stack applications.
Vue.js Reusable List transitions allow the developers to create one animation and then use it across all the components. In the TransitionGroup, we can perform the same list transition for different elements. We can have an item slide-in animation on a list and we may want to apply the same on the other list. This can be easily done on a list by providing the animation and using the common name on the lists we may want to have similar animations.
Vue.js Reusable List transitions Attribute:
- name: This attribute takes the name of the animation that we have defined in our style code. We will use the same animation name on the different lists.
- tag: This field asks for the type of element that the TransitionGroup is, whether an ordered list(ol) or unordered list(ul).
Syntax: We can as many lists on which we need to perform transition and then name the transition same across all elements.
<!-- First -->
<TransitionGroup tag="ul" name="list">
...
</TransitionGroup>
<!-- Second -->
<TransitionGroup tag="ul" name="list">
...
</TransitionGroup>
<!-- Third -->
<TransitionGroup tag="ul" name="list">
...
</TransitionGroup>
...
<style>
/*CSS Classes*/
.list-move{},
.list-enter-active{},
.list-leave-active {}
.list-enter-from{},
.list-leave-to {},
.list-leave-active {}
</style>
Example: In the following example, we have two lists, one has a list of tutorials and another list has a list of programming languages. Then, we have a shuffle button for each of the lists, on which clicking, the respective list shuffles.
Step 1: First create a project using the following command.
npm init vue@latest
Project Structure: The project structure is as follows:
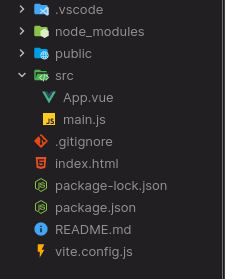
Project Structure
Step 2: First of all, install the package for shuffling the list using npm as follows:
npm i shuffle-array
Step 3: Now the script has the data for tutorials and the list and contains the function to shuffle the list.
App.vue
<script>
import shuffle from "shuffle-array" ;
export default {
data() {
return {
tutorials: [
"Data Structures" ,
"Competitive Programming" ,
"Interview preparation" ,
],
languages: [ "Java" ,
"C++" ,
"Python 3" ,
"Javascript" ],
};
},
methods: {
shuffleList(listIndex) {
if (listIndex === 1) this .tutorials = shuffle( this .tutorials);
else this .languages = shuffle( this .languages);
},
},
};
</script>
|
Step 4: Next in the template section, we have the TransitionGroup for each of the lists with the shuffle button.
App.vue
<template>
<center>
<h1 style= "text-align: center;
color: green" >
GeeksforGeeks
</h1>
<strong>
Vue.js Reusable List Transitions
</strong>
<br />
</center>
<div style= "margin: auto;
width: max-content" >
<h4>Topics</h4>
<center>
<button
style= "
background-color: greenyellow;
color: black;
font-size: x-small;
border-radius: 0.5rem;"
@click= "shuffleList(1)" >
Shuffle
</button>
</center>
<TransitionGroup tag= "ul"
style= "list-style-type: none"
name= "list" >
<li v- for = "tutorial in tutorials" :key= "tutorial"
style= "height: 1.6em" >
{{ tutorial }}
</li>
</TransitionGroup>
<h4>Programming Languages</h4>
<center>
<button
style= "
background-color: cadetblue;
color: black;
font-size: x-small;
border-radius: 0.5rem;"
@click= "shuffleList(2)" >
Shuffle
</button>
</center>
<TransitionGroup tag= "ul"
style= "list-style-type: none"
name= "list" >
<li v- for = "lang in languages" :key= "lang"
style= "height: 1.6em" >
{{ lang }}
</li>
</TransitionGroup>
</div>
</template>
|
Step 5: Finally the style part of the code, we have the entering and leaving transition as well as move transition.
App.vue
<style>
.list-move,
.list-enter-active,
.list-leave-active {
transition: all 1s cubic-bezier(0.075, 0.82, 0.165, 1);
}
.list-enter-from,
.list-leave-to {
opacity: 0;
transform: translateX(-2rem);
}
.list-leave-active {
position: absolute;
}
</style>
|
Here is the full code.
App.vue
<script>
import shuffle from "shuffle-array" ;
export default {
data() {
return {
tutorials: [
"Data Structures" ,
"Competitive Programming" ,
"Interview preparation" ,
],
languages: [ "Java" ,
"C++" ,
"Python 3" ,
"Javascript" ],
};
},
methods: {
shuffleList(listIndex) {
if (listIndex === 1) this .tutorials = shuffle( this .tutorials);
else this .languages = shuffle( this .languages);
},
},
};
</script>
<template>
<center>
<h1 style= "text-align: center;
color: green" >
GeeksforGeeks
</h1>
<strong>
Vue.js Reusable List Transitions
</strong>
<br />
</center>
<div style= "margin: auto;
width: max-content" >
<h4>Topics</h4>
<center>
<button
style= "
background-color: greenyellow;
color: black;
font-size: x-small;
border-radius: 0.5rem;"
@click= "shuffleList(1)" >
Shuffle
</button>
</center>
<TransitionGroup tag= "ul"
style= "list-style-type: none"
name= "list" >
<li v- for = "tutorial in tutorials" :key= "tutorial"
style= "height: 1.6em" >
{{ tutorial }}
</li>
</TransitionGroup>
<h4>Programming Languages</h4>
<center>
<button
style= "
background-color: cadetblue;
color: black;
font-size: x-small;
border-radius: 0.5rem;"
@click= "shuffleList(2)" >
Shuffle
</button>
</center>
<TransitionGroup tag= "ul"
style= "list-style-type: none"
name= "list" >
<li v- for = "lang in languages" :key= "lang"
style= "height: 1.6em" >
{{ lang }}
</li>
</TransitionGroup>
</div>
</template>
<style>
.list-move,
.list-enter-active,
.list-leave-active {
transition: all 1s cubic-bezier(0.075, 0.82, 0.165, 1);
}
.list-enter-from,
.list-leave-to {
opacity: 0;
transform: translateX(-2rem);
}
.list-leave-active {
position: absolute;
}
</style>
|
Step 6: Run the project using the following command and see the output.
npm run serve
Output: It will run the project on http://localhost:3000/ and the result will be as follows.
Reference: https://vuejs.org/guide/built-ins/transition.html#reusable-transitions
Share your thoughts in the comments
Please Login to comment...