Vue.js Prop Passing Details
Last Updated :
07 Nov, 2023
Vue.js Props are used to pass data to the component. For passing details to props we have to first declare props. To declare the prop name we choose a name that is more descriptive of the value that it holds. In this article, we will see the Prop passing the details in Vue.js. The following are the different properties of the prop:
We will explore the above-mentioned Props in Vue.js in detail, along with understanding their basic implementation through the illustration.
Prop Name Casing
- We have different types of conventions for writing the names of props such as camelCasel, kebab-case, PascalCase, etc. We generally use camelCase to long prop name because it allows us to directly reference them in dom because they are valid JavaScript identifiers.
props : { newProp1 : String // cameCase example }
- We can use the Kebab case to pass the details of the props to the component.
<Student student-name='Sam Snehil' />
- We use PascalCase for component tags which differentiates the component tag from the native tag which helps readers to understand the code.
<StudentClass /> // component tag
static vs. Dynamic Props
- We have different ways to pass details to Prop Sometimes it can be static and sometimes it can be dynamic. Static props are a value that is fixed throughout the existence of a web page. Like below:
<Student student-name='Sam' />
- Dynamic value is bound with a variable if the variable is changed props data will also be changed.
<Student :student-name='variable' />
Passing different values
We can pass all possible data types to props. Some of them are:
<Student Age=23 /> // Static
<Student :Age='age' /> // Dynamic
<Student name='Same /> // Static
<Student :name='name' /> // Dynamic
<Student StudentPass='false' />
<Student :StudentPass="stud1.pass" />
<Student student-marks='[89, 38, 83]' />
<Student :student-marks="stud1.marks" />
<Students student1="{ name: 'sam', age: '23' }" />
<Students :student1="students.stud1" />
Binding Multiple Properties Using an Object
If we want to pass all pros in one object we can bind all properties using Objects and v-bind directive
// One props is name and one age we want to pass it together
let stud1 = { name : 'Sam', age: 23 }
// It is equivalent to <Student :name="stud1.name" :age="stud1.age" />
<Students v-bind="sutd1" />
Syntax
<ComponentName props = 'PropsValue' />
Parameter
- props: It name of the props to which we pass data.
- PropsValue: It is the details we pass to prop.
Example 1: In this example, we will pass static details to props and view them using name case camel case, and kebab-case.
HTML
< template >
< h1 >GeeksforGeeks</ h1 >
< Student student-name = "Sam"
student-batch = "5th"
student-age = 23
student-course = "Mtech" />
</ template >
< script >
import Student from './components/Student.vue';
export default {
name: 'App',
components: {
Student,
},
}
</ script >
< style >
#app {
text-align: center;
margin-top: 60px;
}
h1 {
color: rgb(40, 212, 103)
}
</ style >
|
HTML
< template >
< h2 >Chick on botton to view the props</ h2 >
< button v-on:click.left = "data = !data" >
Show
</ button >
< h2 v-if = "data" >Name: {{ studentName }}< br />
Batch: {{ studentBatch }}< br />
Course: {{ studentCourse }}< br />
Age: {{ studentAge }}< br />
</ h2 >
</ template >
< script >
export default {
name: 'StudentL',
props: {
studentName: String,
studentBatch: String,
studentAge: String,
studentCourse: String
},
data() {
return { data: 0 };
}
}
</ script >
|
Output:
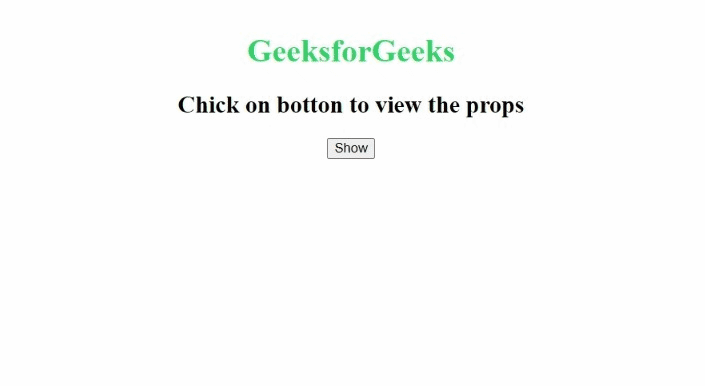
static
Example 2: In this example, we will pass dynamic details to props and view them.
HTML
< template >
< h1 >GeeksforGeeks</ h1 >
< Student v-bind = 'student[i]' />
< button @ click = "i=(i+1)%2" >
change
</ button >
</ template >
< script >
import Student from './components/Student.vue';
export default {
name: 'App',
components: {
Student,
},
data() {
return {
student: [
{ name: 'Sam',
Age: 23,
Course: "Btech",
Batch: '3rd' },
{ name: 'Sam2',
Age: 23,
Course: "Mtech",
Batch: '4rd' }],
i: 0
};
}
}
</ script >
< style >
#app {
text-align: center;
margin-top: 60px;
}
h1 {
color: rgb(40, 212, 103)
}
</ style >
|
HTML
< template >
< h2 >Chick on botton to view the props</ h2 >
< button v-on:click.left = "data = !data" >
Show
</ button >
< h2 v-if = "data" > Name : {{ name }} < br />
Course : {{ Course }} < br />
Age : {{ Age }}< br />
Batch : {{ Batch }}</ h2 >
</ template >>
< script >
export default {
name: 'StudentL',
props: {
name: String,
Course: String,
Age: Number,
Batch: String,
},
data() {
return { data: 0 };
}
}
</ script >
|
Output:
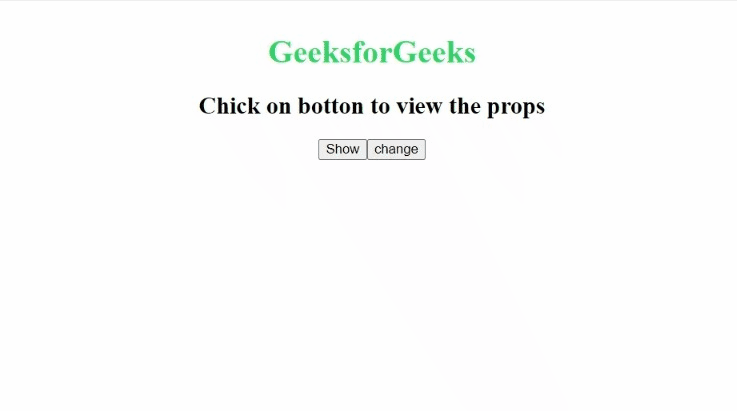
dynamic
Reference: https://vuejs.org/guide/components/props.html#prop-passing-details
Share your thoughts in the comments
Please Login to comment...