Vue.js Prop Validation
Last Updated :
25 Oct, 2023
Vue.js Prop Validation is a method to specify the requirements for their props. If the requirement is not met Vue warns in the browser’s console. To define a validator we can use an object with a requirement or string or array containing the props name. There are several types of validation for props, which are described below.
Prop Validation Types
- Basic type check: We only mention the type of props we require. We can use null and undefined to get any type of data.
- Multiple types: We can use an array of datatypes which are valid datatypes for props.
- Requirement Object: We can define objects that mention the type of the props.
- Object with required: We can define objects that mention the necessity condition for props.
- Default value: We can define a default value for props that will be used in case of props are not passed.
- Use Number as default value: Number is used as default value.
- Use Default function: Use a function that returns the default value.
- Object with default value: If we have props that expect an object in such case we can use default value for props which is used when props are not passed from the parent. The default value is defined using the default function which returns the default value of an object.
- Function with default value: We can use type as a function and can define the default function as the return default function.
- Custom Validator function: We can define a custom function that checks that props must be specific.
- Required String: We can define the type of props as a string and make it necessary using the required parameters.
Syntax
props: {
// Number, String, Boolean, Date, Array, Object, Function etc
type: Type,
// True for neccessity and false if not neccessary.
required: true / false,
default (props) {
// Default values e.g. 68, "Geeks"
return { data: 'value' };
}
validator(prop) {
// Validator function
return statement;
}
// Other user defined data
}
Properties
Inside the details of the props, the following properties are valid
- type: It should valid JavaScript constructor.
- required: It should be true if a prop is necessary else false.
- default: It is the default value passed to the props.
- validator(): It is a validation function that checks if the value passed is of a specific type or not.
There are several details related to props validation, which are described below:
- By default all props are optional.
- Use required props for mandatory conditions.
- The absence of Boolean props has the value false in case the default is not available.
- Absence of Other props Boolean has an undefined value in case the default is not available.
Example 1: In this example, we will validate the data type of each prop and see the output if we don’t pass the same type as required.
HTML
< template >
< h1 >GeeksforGeeks</ h1 >
< Student v-bind = "temp" />
</ template >
< script >
import Student
from './components/Student.vue';
import StudentC
from './components/StudentClass.vue';
export default {
name: 'App',
components: {
Student,
},
data() {
return { temp: { name: new StudentC('Sam', 'Snehil'),
Course: 'Mtech' } };
}
}
</ script >
< style >
#app {
text-align: center;
margin-top: 60px;
}
h1 {
color: rgb(40, 212, 103)
}
</ style >
|
HTML
< template >
< h2 >Chick on botton to view the props</ h2 >
< button v-on:click.left = "data = !data" >
Show
</ button >
< h2 v-if = "data" >Name: {{ name }}< br />
Batch: {{ Batch }}< br />
Course: {{ Course }}< br />
Age: {{ Age }}< br />
Year: {{ year }}< br />
</ h2 >
</ template >
< script >
import StudentC from './StudentClass.vue';
export default {
name: 'StudentL',
props: {
name: StudentC,
Batch: String,
Age: [String, Number],
Course: { validator(value) {
return ['Btech', 'MBA'].includes(value);
} },
year: { type: Number, default: 1 },
},
data() {
return { data: 0 };
}
}
</ script >
|
Javascript
<script>
export default class StudentC {
constructor(firstName, lastName) {
this .firstName = firstName;
this .lastName = lastName;
}
getFullName()
{
return this .firstName+ ' ' + this .lastName;
}
}
</script>
|
Output
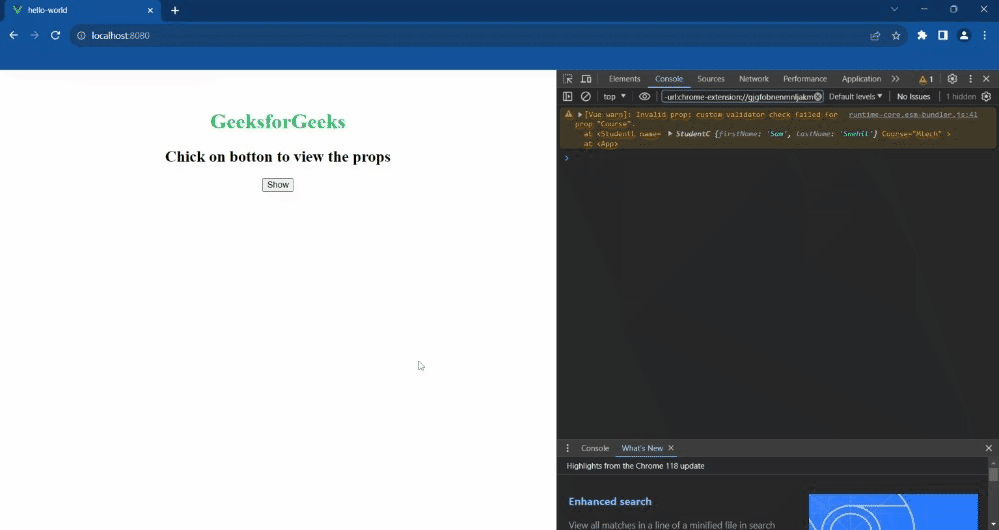
Example 2: In this example, we will validate the props by using the required attributes, while when we don’t pass it.
HTML
< template >
< h1 >GeeksforGeeks</ h1 >
< Student v-bind = "temp" />
</ template >
< script >
import Student
from './components/Student.vue';
import StudentC
from './components/StudentClass.vue';
export default {
name: 'App',
components: {
Student,
},
data() {
return { temp: { name: new StudentC('Sam', 'Snehil'),
Course: 'Mtech' } };
}
}
</ script >
< style >
#app {
text-align: center;
margin-top: 60px;
}
h1 {
color: rgb(40, 212, 103)
}
</ style >
|
HTML
< template >
< h2 >Chick on botton to view the props</ h2 >
< button v-on:click.left = "data = !data" >
Show
</ button >
< h2 v-if = "data" >
Name: {{ name.getFullName() }}
< br />
Batch: {{ Batch }}< br />
Course: {{ Course }}< br />
Age: {{ Age }}< br />
</ h2 >
</ template >
< script >
import StudentC from './StudentClass.vue';
export default {
name: 'StudentL',
props: {
name: StudentC, // {type: String, required: true},
Batch: { type: String, default() { return '1st' } },
Age: { type: String, default: '23' },
Course: { type: String, required: false }
},
data() {
return { data: 0 };
}
}
</ script >
|
Javascript
<script>
export default class StudentC {
constructor(firstName, lastName) {
this .firstName = firstName;
this .lastName = lastName;
}
getFullName()
{
return this .firstName+ ' ' + this .lastName;
}
}
</script>
|
Output
Reference: https://vuejs.org/guide/components/props.html#prop-validation
Share your thoughts in the comments
Please Login to comment...